Question
BONUS_RATE = 1.50 class WorkoutClass: A workout class that can be offered at a gym. === Public Attributes === name: The name of the workout
BONUS_RATE = 1.50
class WorkoutClass: """A workout class that can be offered at a gym.
=== Public Attributes === name: The name of the workout class.
=== Private Attributes === _required_certificates: The certificates that an instructor must hold to teach this WorkoutClass. """ name: str _required_certificates: list[str]
def __init__(self, name: str, required_certificates: list[str]) -> None: """Initialize a new WorkoutClass called
>>> workout_class = WorkoutClass('Kickboxing', ['Strength Training']) >>> workout_class.name 'Kickboxing' """ self.name = name self._required_certificates = required_certificates[:]
def get_required_certificates(self) -> list[str]: """Return all the certificates required to teach this WorkoutClass.
>>> workout_class = WorkoutClass('Kickboxing', ['Strength Training']) >>> needed = workout_class.get_required_certificates() >>> needed ['Strength Training'] >>> needed.append('haha') >>> try_again = workout_class.get_required_certificates() >>> try_again ['Strength Training'] """ # Make a copy of the list to avoid aliasing return self._required_certificates[:]
def __eq__(self, other: Any) -> bool: """Return True iff this WorkoutClass is equal to
Two WorkoutClasses are considered equal if they have the same name and the same required certificates.
>>> workout_class = WorkoutClass('Kickboxing', ['Strength Training']) >>> workout_class2 = WorkoutClass('Kickboxing', ['Strength Training']) >>> workout_class == workout_class2 True >>> d = {1: 17} >>> workout_class == d False """ if not isinstance(other, WorkoutClass): return False return (self.name == other.name and self._required_certificates == other._required_certificates)
class Instructor: """An instructor at a Gym. Each instructor may hold certificates that allows them to teach specific workout classes. === Public Attributes === name: This Instructor's name. === Private Attributes === _id: This Instructor's identifier. _certificates: The certificates held by this Instructor. """ name: str _id: int _certificates: List[str]
def __init__(self, instructor_id: int, instructor_name: str) -> None: """Initialize a new Instructor with an
def get_id(self) -> int: """Return the id of this Instructor. >>> instructor = Instructor(1, 'Matylda') >>> instructor.get_id() 1 """ return self._id
def add_certificate(self, certificate: str) -> bool: """Add the
def get_num_certificates(self) -> int: """Return the number of certificates held by this instructor. >>> instructor = Instructor(1, 'Matylda') >>> instructor.add_certificate('Strength Training') True >>> instructor.get_num_certificates() 1 """ return len(self._certificates)
def can_teach(self, workout_class: WorkoutClass) -> bool: """Return True iff this instructor has all the required certificates to teach the workout_class. >>> matylda = Instructor(1, 'Matylda') >>> kickboxing = WorkoutClass('Kickboxing', ['Strength Training']) >>> matylda.can_teach(kickboxing) False >>> matylda.add_certificate('Strength Training') True >>> matylda.can_teach(kickboxing) True """ for item in self._certificates: if item not in \ WorkoutClass.get_required_certificates(workout_class): return False return True
def get_certificates(self): """ Return the list of certificates that the instructor has >>> instructor = Instructor(1, 'Matylda') >>> instructor.add_certificate('Strength Training') True >>> instructor.get_certificates() ['Strength Training'] """ return self._certificates
class Gym: """A gym that hosts workout classes taught by instructors.
All offerings of workout classes start on the hour and are 1 hour long. If a class starts at 7:00 pm, for example, we say that the class is "at" the timepoint 7:00, or just at 7:00.
=== Public Attributes === name: The name of the gym.
=== Private Attributes === _instructors: The instructors who work at this Gym. Each key is an instructor's ID and its value is the Instructor object representing them. _workouts: The workout classes that are taught at this Gym. Each key is the name of a workout class and its value is the WorkoutClass object representing it. _room_capacities: The rooms and capacities in this Gym. Each key is the name of a room and its value is the room's capacity, that is, the number of people who can register for a class in the room. _schedule: The schedule of classes offered at this gym. Each key is a date and time and its value is a nested dictionary describing all offerings that start then. In the nested dictionary, each key is the name of a room that has an offering scheduled then, and its value is a tuple describing the offering. The tuple elements record, in order: - the instructor teaching the class, - the workout class itself, and - a list of registered clients. Each client is represented in the list by a unique string.
=== Representation Invariants === - All instructors in _schedule are in _instructors (the reverse is not necessarily true). - All workout classes in _schedule are in _workouts (the reverse is not necessarily true). - All rooms recorded in _schedule are also recorded in _room_capacities (the reverse is not necessarily true). - Two workout classes cannot be scheduled at the same time in the same room. - No instructor is scheduled to teach two workout classes at the same time. I.e., there does not exist timepoint t, and rooms r1 and r2 such that _schedule[t][r1][0] == _schedule[t][r2][0] - No client can take two workout classes at the same time. I.e., there does not exist timepoint t, and rooms r1 and r2 such that c in _schedule[t][r1][2] and c in _schedule[t][r2][2] - If an instructor is scheduled to teach a workout class, they have the necessary qualifications. - If there are no offerings scheduled at date and time
FUNCTIONS TO COMPLETE
def offerings_at(self, time_point: datetime) -> list[dict[str, str | int]]: """Return a list of dictionaries, each representing a workout offered at this Gym at
The offerings should be sorted by room name, in alphabetical ascending order.
Each dictionary must have the following keys and values: 'Date': the weekday and date of the class as a string, in the format 'Weekday, year-month-day' (e.g., 'Monday, 2022-11-07') 'Time': the time of the class, in the format 'HH:MM' where HH uses 24-hour time (e.g., '15:00') 'Class': the name of the class 'Room': the name of the room 'Registered': the number of people already registered for the class 'Available': the number of spots still available in the class 'Instructor': the name of the instructor If there are multiple instructors with the same name, the name should be followed by the instructor ID in parentheses e.g., "Diane (1)"
If there are no offerings at
NOTE: - You MUST use the helper function create_offering_dict from gym_utilities to create the dictionaries, in order to make sure you match the format specified above. - You MUST use the helper method _is_instructor_name_unique when deciding how to format the instructor name.
>>> ac = Gym('Athletic Centre') >>> diane1 = Instructor(1, 'Diane') >>> diane1.add_certificate('Cardio 1') True >>> diane2 = Instructor(2, 'Diane') >>> david = Instructor(3, 'David') >>> david.add_certificate('Strength Training') True >>> ac.add_instructor(diane1) True >>> ac.add_instructor(diane2) True >>> ac.add_instructor(david) True >>> ac.add_room('Dance Studio', 50) True >>> ac.add_room('Room A', 20) True >>> boot_camp = WorkoutClass('Boot Camp', ['Cardio 1']) >>> ac.add_workout_class(boot_camp) True >>> kickboxing = WorkoutClass('KickBoxing', ['Strength Training']) >>> ac.add_workout_class(kickboxing) True >>> t1 = datetime(2022, 9, 9, 12, 0) >>> ac.schedule_workout_class(t1, 'Dance Studio', boot_camp.name, 1) True >>> ac.schedule_workout_class(t1, 'Room A', kickboxing.name, 3) True >>> ac.offerings_at(t1) == [ ... { 'Date': 'Friday, 2022-09-09', 'Time': '12:00', ... 'Class': 'Boot Camp', 'Room': 'Dance Studio', 'Registered': 0, ... 'Available': 50, 'Instructor': 'Diane (1)' }, ... { 'Date': 'Friday, 2022-09-09', 'Time': '12:00', ... 'Class': 'KickBoxing', 'Room': 'Room A', 'Registered': 0, ... 'Available': 20, 'Instructor': 'David' } ... ] True """
Step by Step Solution
There are 3 Steps involved in it
Step: 1
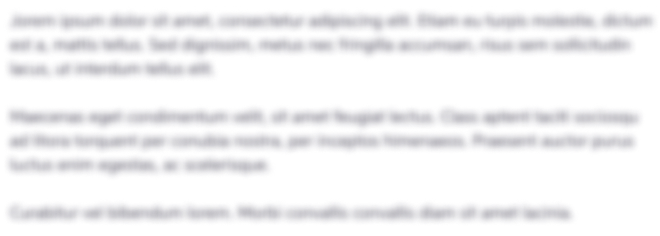
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started