Question
C++: 1) Implement the unfinished methods of DLL class. Modify the methods in DLL.cpp, do NOT change anything in DLL.h or test.cpp. Use test.cpp to
C++:
1) Implement the unfinished methods of DLL class. Modify the methods in DLL.cpp, do NOT change anything in DLL.h or test.cpp. Use test.cpp to test your implementations.
This is DLL.h:
#include
struct Node{ string ssn; string name; Node* succ; Node* pred; };
class DLL{ private: Node* headPtr; int itemCount; public: DLL(); DLL(DLL& n); virtual ~DLL(); Node* getHeadPtr(); int search(string ss) const; bool insert(string ss, string name, int & count); bool remove(string ss, int & count); int size(); void display(); };
This is DLL.cpp w/ Unfinished Methods:
#include "DLL.h" #include
// default constructor is already implemented // do not modify the default constructor DLL::DLL(){ headPtr = nullptr; itemCount = 0; }
// return the head pointer of the list // it is already implemented, do not modify it Node* DLL::getHeadPtr(){ return headPtr; }
// copy construct, which copies an existing list n // the new list is a different object from n // the new list and object n have the same contents // Please implement it DLL::DLL(DLL& n){ }
// destructor // release every node of the list // Please implement it DLL::~DLL(){ }
// if some node has SSN matcthes string ss // return the index value of the node // the index value of the first node is 0, the second node is 1, etc. // if there is node matching ss, return -1 int DLL::search(string ss)const{
return -1; }
// insert (ss, name) to the existing list // the SSN values are each node are organized in INCREASING order // if there is a node matching ss value, return false; otherwise true // else create a node with (ss, name), insert the node to the proper position // parameter count is the counter of number of valid insertion // when you implement this method, consider the following situations: // 1. list is empty // 2. node should be inserted to the beginning of the list // 3. node should be inserted to the middle of the list // 4. node should be inserted to the end of the list bool DLL::insert(string ss, string name, int & count){ return false; }
// remove node containing ss value // if there is no node containing ss, return false; otherwise true // consider the following situations: // 1. list is empty // 2. node containing ss value is the first node // 3. node containing ss value is in the middle of the list // 4. node containing ss value is the last node of the list bool DLL::remove(string ss, int & count){ return false; }
// return the number of the nodes // it is already implemented, do not modify it int DLL::size(){ return itemCount; }
// iterate through each node // print out SSN and memory address of each node // do not modify this method void DLL::display(){ Node* temp; temp = headPtr; while (temp!= nullptr) { cout << temp->ssn << "\t" << temp << endl; temp = temp->succ; } }
This is the test.cpp that will test the methods:
#include "DLL.h" #include
using namespace std;
int main(){
DLL myList; int counter=0; int dCounter=0; myList.insert("30", "Jack Eblin", counter); myList.insert("40", "Liz Smith", counter); myList.insert("10", "Mike Dutter", counter); myList.insert("20", "Kitty Lekberg", counter); myList.insert("50", "Carma Meshew", counter);
cout << "After insertion, we should have 10 20 30 40 50 in order" << endl; myList.display(); cout << "Searching 30 in the list, result should be 2" << endl; cout << myList.search("30") << endl; myList.remove("10", dCounter); myList.remove("50", dCounter); cout <<"After deletion, we should have 20 30 40 in order"<< endl; myList.display(); cout << "Testing copy constructor" << endl; DLL* temp = new DLL(myList); cout << "Contents of the original list" << endl; myList.display(); cout << "Contents of the new list, the memory address of the this list must be different from the original list" << endl; if (myList.getHeadPtr() != nullptr) { temp->display(); } return 0; }
This is the text file that the file is working on:
i 586412373 NICOLA EVANGELISTA i 177228167 MEAGAN LEKBERG i 586412373 JEFF DUTTER i 760846483 KITTY MANZANERO i 061899135 CATHERIN MCCREIGHT i 087300880 CARMA KULHANEK i 177264549 VALERY KOSAKOWSKI i 210044984 SHEILAH MONGES d 760846483 KITTY MANZANERO r 760846483 KITTY MANZANERO r 007980295 DELPHIA SIMISON i 493515916 VERONIKA TADENA d 401991909 MCKINLEY WESTERFELD i 793267575 TEMIKA MESHEW i 319373939 MARGIT EBLIN
Step by Step Solution
There are 3 Steps involved in it
Step: 1
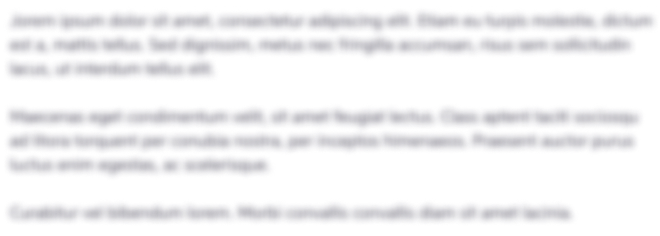
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started