Question
**C++, Case 6 Code Provided*** One of the most common reasons we use computers is to search for some type of information. In this lab
**C++, Case 6 Code Provided***
One of the most common reasons we use computers is to search for some type of information. In this lab you are going to write an application that allows users to enter baby names and determine their popularity. You will be getting practice using a number of topics you have learned recently, including:
vectors
file I/O
I/O manipulators
We will be requiring you to write and use a number of functions in an effort to help show you how you can use functions to make your program more readable, by moving some of the logic out of main.
String streams
PART 1 Using Vectors and String streams.
A baby name file is a space-delimited file in which each line consists of a baby name, the names gender, and the number of babies given that name in the given birth year. The most popular names are listed first and the least popular names are listed last. For example, the file begins with:
Emma F 20799
Olivia F 19674
Noah M 19144
Sophia F 18490
Liam M 18342
Mason M 17092
Isabella F 16950
The first line indicates that Emma was the most popular girls name and was the name given to 20,799 babies. The third line indicates that Noah is the most popular boy name and was the name given to 19,144 babies.
In this program you will modify case 6 (attached at end) in the following ways.
- You may no longer use atoi you need to convert the number of names to print from the command line to an int using stringstreams.
- Convert the name and frequency arrays into vectors (you will no longer need to keep the size of the arrays so do away with that ).
- You will then input the names into the 4 vectors by getting an entire line of data then using string streams to separate it into fields (use getline and string streams)
- You will now be passing vectors instead of arrays.
- You will still be outputting from the vectors to the file.
Part 2 allowing a user to search for names
In this phase you will extend the program from by having your program repeatedly read names from stdin until the input from stdin is exhausted. The program will search for a matching name among the girls and boys. If a match is found, your program should output the rank (i.e., 1st, 2nd, 3rd most popular) and the frequency of the name. The program should also indicate if there is no match.
Here is a sample interaction with the program (input from stdin is in blue):
Brad
Name Girl Rank Frequency Boy Rank Frequency
Brad --- --- 1911 75
Smiley
Smiley --- --- --- ---
Justice
Justice 421 756 531 518
Miah
Miah 622 459 --- ---
Bradley
Bradley 3579 43 184 2308
Please note that:
If a name is not found, then you print three dashes (---) in the rank and frequency fields to indicate that there is not a match.
You only print the header for the report once. The header reads:
Name Girl Rank Frequency Boy Rank Frequency
You will find that when the report gets printed in Code Assessor, it looks very nice if the header is only printed once.
The output is to be formatted as follows:
Name field: 11 characters wide, left-justified.
Girl Rank/Boy Rank: 10 characters wide, right-justified
Frequency: 10 characters wide, right-justified
No spaces between fields
Implementation: You must implement this part by writing a function named searchNames that takes a name vector and a search name as parameters. It returns the index at which that name appears in the vector or -1 if that name could not be found. Since your boy and girl vectors sort names by decreasing rank, the index will give you the names rank, although you will need to add 1 to obtain the names rank since ranks start at 1 and indices start at 0. For example, Emma will appear at index 0 but her rank is 1.
Your main program must call searchNames to find the index where the name occurs in the girls and boys vectors. Since searchNames only takes one vector, you will need to call it twice: once for the boys and once for the girls. The index returned by searchNames can be used to look up the frequency of the name in the frequency vectors. (you may NOT write 2 search functions )
Your main program will need to use a loop to repeatedly read names from stdin, locate the name in the girls and boys vectors using searchNames, and then print the information for that name, formatted as specified above. The output for this part will be in addition to the output from Part 1 but the output for part 2 will be stdout.
Case 6 Code:
#include #include #include
#define MAX 10000 // maximum entries in the file could be.
using namespace std;
void printTopNamesToFile( ofstream &outFile, // argument to print output to file string girlsNames[MAX], string boysNames[MAX], int girlsFreq[MAX], int boysFreq[MAX], int nGirls, int nBoys, int n) { // width as 10 and left align; outFile.width(10); outFile< // width as 10 and right align; outFile.width(10); outFile< outFile<<" "; outFile.width(10); outFile< outFile.width(10); outFile<
// the exit condition is either the size or n must be 0 // this way even if user enter number greater than the actual entries in // file we will display all the data present in file. int i; for(i = 0;i < nGirls && i outFile.width(10); outFile< outFile.width(10); outFile< outFile<<" "; outFile.width(10); outFile< outFile.width(10); outFile< n--;// we need only n-1 names. hence decreasing }
// case in which the number of girls are more than number of boys. if( n!=0 && i != nGirls) { while(i < nGirls && n!=0) { outFile.width(10); outFile< outFile.width(10); outFile< i++; n--; } }
// case in which the number of boys are more than number of girsl. if( n!=0 && i != nBoys) { while(i < nBoys && n!=0) { outFile.width(10); outFile< outFile.width(10); outFile< outFile<<" "; outFile.width(10); outFile< outFile.width(10); outFile< i++; n--; } }
outFile.close(); // closing the file to print the output. }
int main(int argc, char*argv[]) {
// checking if the number of arguments to be equal to 4 if(argc != 4) { cout<<"Wrong number of arguments Correct usage: ifilename ofilename #namesToPrint "; exit(0); //exiting by 0 as mentioned in question. }
ifstream inFile; ofstream outFile;
inFile.open(argv[1]);
// if file is not present we must show error if(!inFile) { cout<<"Error opening file "; exit(1); }
outFile.open(argv[2]);
// if file is not present we must show error if(!outFile.is_open()) { cout<<"Error opening file "; exit(1); }
int n = atoi(argv[3]);
string na,g; int c;
// arrays to store information of names string girlNames[MAX], boysNames[MAX]; // arrays to store frequency int girlFreq[MAX], boysFreq[MAX]; // count of number of boys and girls int nBoys=0, nGirls = 0;
//reading till the end of file. // looking if reading of name is end of file. // if not then we will read the gender and count. // else we will come out of loop. while(inFile >> na) { inFile>>g; inFile>>c;
// creating object and inserting into vector; if(g == "F") { girlNames[nGirls] = na; girlFreq[nGirls++] = c; } else { boysNames[nBoys] = na; boysFreq[nBoys++] = c; }
}
//calling function with 7 parameters as stated in question. // passing out stream to the function. printTopNamesToFile(outFile, girlNames, boysNames, girlFreq, boysFreq,nGirls, nBoys, n);
return 0;
}
:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
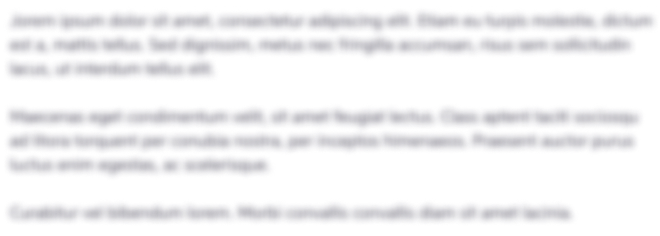
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started