Question
C Code: Please complete in C Description: The purpose of this assignment is to practice writing code that calls functions, and contains loops and branches.
C Code:
Please complete in C
Description: The purpose of this assignment is to practice writing code that calls functions, and contains loops and branches. You will create a C program that prints a menu and takes user choices as input. The user will make choices regarding different "triangles" that will be printed to the screen.
Your code must contain at least one of all of the following control types:
- nested for() loops
- a while() or a do-while() loop
- a switch() statement
- an if-else statement
- functions (see below)
Important! Consider which control structures will work best for which aspect of the assignment. For example, which would be the best to use for a menu? The first thing your program will do is print a menu of choices for the user. You may choose your own version of the wording or order of choices presented, but each choice given in the menu must match the following:
Menu Choice | Valid User Input Choices |
Enter/Change Character | 'C' or 'c' |
Enter/Change Number | 'N' or 'n' |
Print Triangle Type 1 (Left Justified) | '1' |
Print Triangle Type 2 (Right Justified) | '2' |
Quit Program | 'Q' or 'q' |
A prompt is presented to the user to enter a choice from the menu. If the user enters a choice that is not a valid input, a message stating the choice is invalid is displayed and the menu is displayed again. Your executable file will be named Lab3_
Your program must have at least five functions (not including main()) including:
- A function that prints the menu of choices for the user, prompts the user to enter a choice, and retrieves that choice. The return value of this function must be void. The function will have one pass-by-reference parameter of type char. On the function's return, the parameter will contain the user's menu choice.
- A function that prompts the user to enter a single character. The return value of the function be a char and will return the character value entered by the user. This return value will be stored in a local variable, C, in main(). The initial default value of this character will be ' ' (blank or space character).
- A function that prompts the user to enter a numerical value between 1 and 15 (inclusive). If the user enters a value outside this range, the user is prompted to re-enter a value until a proper value is entered. The return value of the function be an int and will return the value entered by the user. This return value will be stored in a local variable, N, in main(). The initial default value of this character will be 0.
- Two "Print Triangle" functions. Each function will take the previously entered integer value N and character value C as input parameters (You will need to ensure that these values are valid before entering these functions). The return values of these functions will be void. The functions will print triangles of N lines containing the input character C. The Left Justified function will print the triangle with the characters Left-Justified. The Right Justified function will print the triangles with the characters "Right-Justified." For example, if the integer value N = 6, and the character value C = '*' and the Right Justified Triangle type is called, the following triangle will be printed:
* ** *** **** ***** ****** If the Left-Justified triangle is to be printed, then the following triangle is printed: * ** *** **** ***** ******
Step by Step Solution
There are 3 Steps involved in it
Step: 1
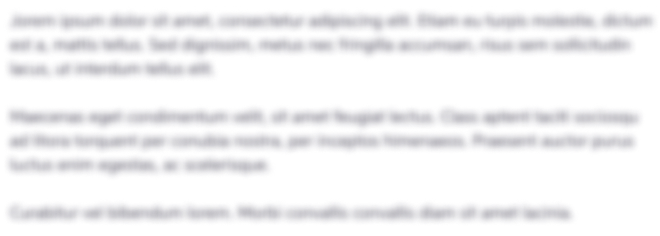
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started