Question
C++ code..use the built-in Queue from the C++ STL(Probe class will use the queue). Planet Class(Planet.cpp and Planet.h) The Planet class should have the following
C++ code..use the built-in Queue from the C++ STL(Probe class will use the queue).
Planet Class(Planet.cpp and Planet.h)
The Planet class should have the following public interface:
- Planet(int)
- Sets the planet's distance from the Star to a random value between 0-3000
- You should still randomly select a planet type between rocky, habitable, and gaseous. Add a new private variable, char type that represents the planet type with a character: 'h'=habitable, 'r'=rocky, 'g'=gaseous.
- unsigned long getID()
- returns the planet's unique id
- unsigned int getDistance()
- returns the Planet's distance from the star
- char getType()
- returns the Planet's type
Star Class(Star.h and (Star.cpp)
The star class should have the following public interface:
- unsigned long addPlanet()
- Creates and adds a Planet to the Star's orbit
- bool removePlanet(unsigned long)
- Removes a Planet based on the PLanet's ID
- Returns true if found, false otherwise
- Planet * getPlanet(unsigned long)
- Returns a pointer to the Planet object.
- nullptr if not found
- vector
& getPlanets() - return a vector containing the planets orbiting the star
Probe Class (Probe.h and Probe.cpp)
The Probe class is going to travel from Star to Star finding habitable planets. At each star, you are going to 'scan' each planet for habitability. You will then add all Habitable planets to a queue for later exploration. Your Probe should have the following public interface:
- vector
getHabitable(Star s) - Takes a Star as a parameter, and inspect all planets orbiting the star to see if they are habitable
- A planet must meet both conditions for habitability to be considered habitable:
- It must be marked habitable type, 'h'
- It's distance from the star is within the Goldilocks Zone: 60-140
- A planet must meet both conditions for habitability to be considered habitable:
- returns a vector containing only habitable planets around that star
- Takes a Star as a parameter, and inspect all planets orbiting the star to see if they are habitable
- void addToQueue(Planet p)
- Adds the planet object to an internal queue
- Planet getNext()
- dequeues the next planet on the Queue, and returns the object
- calling getNext() on a Probe with no planets is undefined
- queue
& getQueue() - return the internal Queue
- This is just for testing purposes
- void shuffle();
- The shuffle method should (surprise) shuffle your Planet queue.
- Your shuffle must be a randomized shuffle
- You can use additional methods or anything else you find useful, as long as you are not violating encapsulation principles (i.e. changing the values of a Planet instead of moving the objects themselves around).
Would appreciate any help. I got most of this done.. Just kinda confused on the Probe class.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
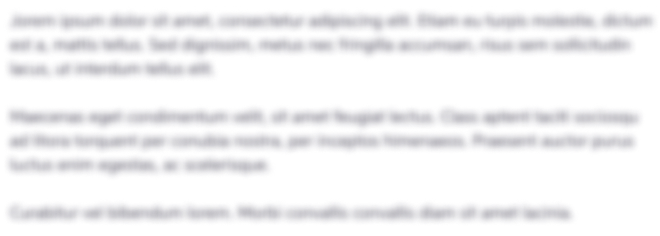
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started