Question
C) Define a Square class that extends Rectangle. The class contains: 1. One double data field named side to denote the side of the square.
C) Define a Square class that extends Rectangle. The class contains:
1. One double data field named side to denote the side of the square.
2. A constructor that creates a Square with the specified side. Pass the value of the side to Rectangles constructor: Rectangle(double side1, double side2). In other words, you have to call super and pass side for both arguments: side1 and side2.
3. A method getArea() that returns the area of the square.
4. A method getPerimeter() that returns the perimeter of the square.
Using the code below:
public abstract class GeometricObject {
public static void main(String[] args)
private String color = "white";
private boolean filled;
private java.util.Date dateCreated;
/** Construct a default geometric object */
protected GeometricObject() {
dateCreated = new java.util.Date();
}
/** Construct a geometric object with color and filled
value */
protected GeometricObject(String color, boolean filled) {
dateCreated = new java.util.Date();
this.color = color;
this.filled = filled;
}
/** Return color */
public String getColor() {
return color;
}
/** Set a new color */
public void setColor(String color) {
this.color = color;
}
/** Return filled. Since filled is boolean,
* the get method is named isFilled */
public boolean isFilled() {
return filled;
}
/** Set a new filled */
public void setFilled(boolean filled) {
this.filled = filled;
}
/** Get dateCreated */
public java.util.Date getDateCreated() {
return dateCreated;
}
@Override
public String toString() {
return "created on " + dateCreated + " color: " +
color +
" and filled: " + filled;
}
/** Abstract method getArea */
public abstract double getArea();
/** Abstract method getPerimeter */
public abstract double getPerimeter();
}
class Triangle extends GeometricObject {
private double side1 = 1.0, side2 = 1.0, side3 = 1.0;
/** Constructor */
public Triangle() {
}
/** Constructor */
public Triangle(double side1, double side2, double
side3) {
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
/** Override method findArea in GeometricObject */
public double getArea() {
double s = (side1 + side2 + side3) / 2;
return Math.sqrt(s * (s - side1) * (s - side2) * (s -
side3));
}
/** Override method findPerimeter in GeometricObject */
public double getPerimeter() {
return side1 + side2 + side3;
}
/** Override the toString method */
public String toString() {
// Implement it to return the three sides
return "Triangle: side1 = " + side1 + " side2 = " +
side2 +
" side3 = " + side3;
}
}
class Test {
public static void main(String[] args) {
GeometricObject gObjectArray [] = new GeometricObject [5];
//Complete your code here
gObjectArray[0] = new Circle(5,5,5);
gObjectArray[1]=new EquilateralTriangle(5);
gObjectArray[2]=new Triangle(5,5,5);
gObjectArray[3]=new Rectangle(5,5);
gObjectArray[4]=new Square(5);
//using loop calling the below print method
for(int i=0;i System.out.println(gObjectArray[i]); printAreaAndPerimeter(gObjectArray[i]); } } private static void printAreaAndPerimeter(GeometricObject gObject) { //Complete your code here System.out.println("Area : "+gObject.getArea()); System.out.println("Perimeter : "+gObject.getPerimeter()); System.out.println(); } } -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- public class Rectangle extends GeometricObject { private double width; private double height; //no argument constructor public Rectangle() { this.width=1; this.height=1; } //parameter argument constructor that takes width and height public Rectangle(double width, double height) { this.width = width; this.height = height; } public Rectangle( double width, double height, boolean filled) { this.width = width; this.height = height; setFilled(filled); } //Return WIdth public double getWidth() { return width; } //Set a new width public void setWidth(double width) { this.width = width; } //Return height public double getHeight() { return height; } //Set height public void setHeight(double height) { this.height = height; } //getArrea return public double getArea() { return width * height; } //Return perimeter public double getPerimeter() { return 2 * (width + height); } public String toString() { return "Rectangel: Width = " + width + " Height = " + height ; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
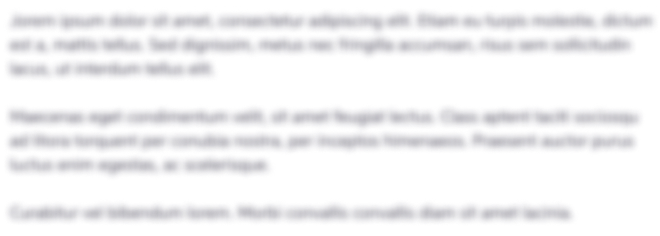
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started