Question
c++ Derive a new subclass of MyClass, name it MySubClass and define a private int type data member named subClassData in this new class. What
c++
Derive a new subclass of MyClass, name it "MySubClass" and define a private "int" type data member named "subClassData" in this new class. What do you need to do is to ensure that the correct copy constructor and assignment operators are called. Verify that your new class works correctly.
Take Away: When to and how to call super class constructors/methods
These are the codes of MyClass.h, MyClass.cpp, and CopyAssignTest.cpp
/*
* MyClass.h
*/
#pragma once
#include
using namespace std;
class MyClass
{
public:
// default constructor
MyClass();
// copy constructor
MyClass(const MyClass& orig);
// destructor
~MyClass(void);
// assignment operator
MyClass& operator=(const MyClass& rhs);
// setters
void setI(int newI);
void setD(double newD);
void setS(string newS);
void setIp(int newIp);
// getters
int getI(void) const;
double getD(void) const;
string getS(void) const;
int getIp(void) const;
private:
// a couple useful utility functions
void copy(const MyClass& other);
void clear(void);
// an assortment of test data members
int i; // primitive
double d; // primitive
string s; // object
int* ip; // primitive, pointer
};
/*
* MyClass.cpp
*/
#include "MyClass.h"
#include
using namespace std;
MyClass::MyClass() : i(0), d(0.0)
{
ip = new int;
*ip = 0;
}
MyClass::MyClass(const MyClass& orig) : ip(nullptr)
{
// Note that this is a new object; no dynamic memory has been allocated
copy(orig);
}
MyClass& MyClass::operator=(const MyClass& rhs)
{
// we have seen this before: a = a is a legal assignment, and shouldn't do anything
if (this != &rhs) {
copy(rhs);
}
return *this;
}
MyClass::~MyClass()
{
clear();
}
void MyClass::setI(int newI)
{
i = newI;
}
void MyClass::setD(double newD)
{
d = newD;
}
void MyClass::setS(string newS)
{
s = newS;
}
//3. Coding: Is MyClass::setIp() implemented correctly? What exactly does it do?
void MyClass::setIp(int newIp)
{
*ip = newIp;
}
int MyClass::getI() const
{
return i;
}
double MyClass::getD() const
{
return d;
}
string MyClass::getS() const
{
return s;
}
int MyClass::getIp() const
{
return *ip;
}
void MyClass::copy(const MyClass& other)
{
i = other.i;
d = other.d;
s = other.s;
// assert(ip == nullptr);
ip = new int;
*ip = *(other.ip);
}
void MyClass::clear()
{
i = 0;
d = 0.0;
s = "";
assert(ip != nullptr);
*ip = 0;
delete ip;
ip = nullptr;
}
/*
* CopyAssignTest
*/
#include
#include "MyClass.h"
#include "MySubClass.h"
using namespace std;
int main(int argc, char** argv)
{
// create object m1 using default constructor
MyClass m1;
// update data members
m1.setD(3.14159);
m1.setI(42);
m1.setS("This is a test");
m1.setIp(7);
cout << "m1 values:" << endl;
cout << '\t' << m1.getD() << ", " << m1.getI() << ", " << m1.getS()
<< ", " << m1.getIp() << endl;
// create object m2 from m1 using copy constructor
MyClass m2(m1);
cout << "m2 values:" << endl;
cout << '\t' << m2.getD() << ", " << m2.getI() << ", " << m2.getS()
<< ", " << m2.getIp() << endl;
// create object m3 from m1 using assignment operator
MyClass m3 = m1;
cout << "m3 values:" << endl;
cout << '\t' << m3.getD() << ", " << m3.getI() << ", " << m3.getS()
<< ", " << m3.getIp() << endl;
// update m2's data
m2.setD(1.7172);
m2.setI(100);
m2.setS("This is a NEW test");
m2.setIp(8);
// copy m2 to m1
m1 = m2;
cout << "m1 values:" << endl;
cout << '\t' << m1.getD() << ", " << m1.getI() << ", " << m1.getS()
<< ", " << m1.getIp() << endl;
// only update m2's data IP which is using dynamically allocated memory
m2.setIp(23);
cout << "m1 values:" << endl;
cout << '\t' << m1.getD() << ", " << m1.getI() << ", " << m1.getS()
<< ", " << m1.getIp() << endl;
cout << "m2 values; last should be different:" << endl;
cout << '\t' << m2.getD() << ", " << m2.getI() << ", " << m2.getS()
<< ", " << m2.getIp() << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
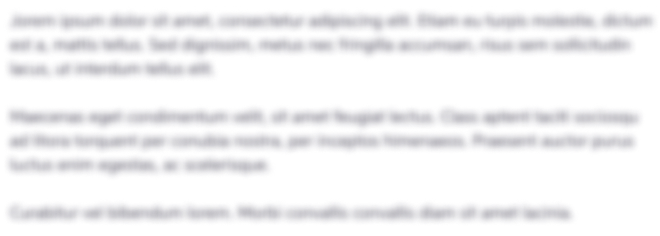
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started