Question
C# Exercise Create a collection class to manage students using the give Solution below. Your collection class should inherit from the collection base class. Extra
C# Exercise
Create a collection class to manage students using the give Solution below. Your collection class should inherit from the collection base class.
Extra Credit: 3 points
Create a procedures plan for your collection class.
StudentInfoFrom.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
namespace Exercise7Solution { public partial class StudentInfoForm : Form { public StudentInfoForm() { InitializeComponent(); }
private void ClearForm() { txtFirstName.Focus(); txtFirstName.Clear(); txtLastName.Clear(); mtbSID.Clear(); mtbAcceptDate.Clear(); mtbAppDate.Clear(); txtCredits.Clear(); txtGPA.Clear(); errorProvider1.Clear(); }
private bool IsValidEntry() { bool answer = true; if (!Student.IsValidFirstName(txtFirstName.Text)) { answer = false; errorProvider1.SetError(txtFirstName, "First name is required"); }
if (!Student.IsValidLastName(txtLastName.Text)) { answer = false; errorProvider1.SetError(txtLastName, "Last name is required"); }
if (!Student.IsValidStudentID(mtbSID.Text)) { answer = false; errorProvider1.SetError(mtbSID, "Student ID is required, 9 digits"); } DateTime appDate; if (DateTime.TryParse(mtbAppDate.Text, out appDate)) { if (!Student.IsValidApplicationDate(appDate)) { answer = false; errorProvider1.SetError(mtbAppDate, "Application date is required, must be today or before"); } } else { answer = false; errorProvider1.SetError(mtbAppDate, "Application date is required, must be today or before"); } int credits; if (int.TryParse(txtCredits.Text, out credits)) { if (!Student.IsValidCredits(credits)) { answer = false; errorProvider1.SetError(txtCredits, "Credits is required, must be zero or more"); } } else { answer = false; errorProvider1.SetError(txtCredits, "Credits is required, must be zero or more"); } decimal gpa; if (decimal.TryParse(txtGPA.Text, out gpa)) { if (!Student.IsValidGPA(gpa)) { answer = false; errorProvider1.SetError(txtGPA, "GPA is required, must be zero or more"); } } else { answer = false; errorProvider1.SetError(txtGPA, "GPA is required, must be zero or more"); } return answer; }
private void btnAdd_Click(object sender, EventArgs e) { errorProvider1.Clear(); if (IsValidEntry()) { Student myStudent = new Student(txtFirstName.Text, txtLastName.Text, mtbSID.Text, DateTime.Parse(mtbAppDate.Text), int.Parse(txtCredits.Text), decimal.Parse(txtGPA.Text)); DateTime accept; if (DateTime.TryParse(mtbAcceptDate.Text, out accept)) myStudent.AcceptanceDate = accept; lstStudents.Items.Add(myStudent); } }
private void btnEdit_Click(object sender, EventArgs e) { errorProvider1.Clear(); int index = lstStudents.SelectedIndex; if (index != -1) { if (IsValidEntry()) { Student myStudent = new Student(txtFirstName.Text, txtLastName.Text, mtbSID.Text, DateTime.Parse(mtbAppDate.Text), int.Parse(txtCredits.Text), decimal.Parse(txtGPA.Text)); DateTime accept; if (DateTime.TryParse(mtbAcceptDate.Text, out accept)) myStudent.AcceptanceDate = accept; lstStudents.Items.RemoveAt(index); lstStudents.Items.Insert(index, myStudent); } } else { errorProvider1.SetError(lstStudents, "Select student, make entries, then click edit to update that student"); } }
private void btnClear_Click(object sender, EventArgs e) { ClearForm(); }
private void btnExit_Click(object sender, EventArgs e) { Close(); }
private void lstStudents_SelectedIndexChanged(object sender, EventArgs e) { errorProvider1.Clear(); } } }
StudentInfoForm.Designer.cs
namespace Exercise7Solution { partial class StudentInfoForm { ///
///
#region Windows Form Designer generated code
///
}
#endregion
private System.Windows.Forms.Label label1; private System.Windows.Forms.TextBox txtFirstName; private System.Windows.Forms.Label label2; private System.Windows.Forms.TextBox txtLastName; private System.Windows.Forms.Label label3; private System.Windows.Forms.Label label4; private System.Windows.Forms.MaskedTextBox mtbAppDate; private System.Windows.Forms.Label label5; private System.Windows.Forms.MaskedTextBox mtbAcceptDate; private System.Windows.Forms.Label label6; private System.Windows.Forms.TextBox txtCredits; private System.Windows.Forms.Label label7; private System.Windows.Forms.TextBox txtGPA; private System.Windows.Forms.ListBox lstStudents; private System.Windows.Forms.MaskedTextBox mtbSID; private System.Windows.Forms.Button btnAdd; private System.Windows.Forms.Button btnEdit; private System.Windows.Forms.Button btnClear; private System.Windows.Forms.Button btnExit; private System.Windows.Forms.ToolTip toolTip1; private System.Windows.Forms.ErrorProvider errorProvider1; } }
Program.cs
using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using System.Windows.Forms;
namespace Exercise7Solution { static class Program { ///
Student.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace Exercise7Solution {
public enum NameOrder { FirstLast, LastFirst}
public class Student { private string firstName, lastName, studentID; private DateTime applicationDate, acceptanceDate; private int credits; private decimal gpa;
public Student(string First, string Last, string SID, DateTime Application, int Credits, decimal Gpa) { this.FirstName = First; this.LastName = lastName; this.StudentID = SID; this.ApplicationDate = Application; this.Credits = Credits; this.GPA = Gpa; }
public Student(string First, string Last, string SID, DateTime Application, DateTime Accept, int Credits, decimal Gpa) { this.FirstName = First; this.LastName = lastName; this.StudentID = SID; this.ApplicationDate = Application; this.AcceptanceDate = Accept; this.Credits = Credits; this.GPA = Gpa; }
public string FirstName { get { return firstName; } set { if (IsValidFirstName(value)) firstName = value; else throw new ApplicationException("First name can't be empty"); } }
public string LastName { get { return lastName; } set { if (IsValidLastName(value)) lastName = value; else throw new ApplicationException("Last name can't be empty"); } }
public string StudentID { get { return studentID; } set { if (IsValidStudentID(value)) studentID = value; else throw new ApplicationException("Invalid Student ID must be 9 digits"); } }
public DateTime ApplicationDate { get { return applicationDate; } set { if (IsValidApplicationDate(value)) applicationDate = value; else throw new ApplicationException("Application date must be today or before"); } }
public DateTime AcceptanceDate { get { return acceptanceDate; } set { acceptanceDate = value; } }
public int Credits { get { return credits; } set { if (IsValidCredits(value)) credits = value; else throw new ApplicationException("Credits must be zero or more"); } }
public decimal GPA { get { return gpa; } set { if (IsValidGPA(value)) gpa = value; else throw new ApplicationException("GPA must be zero or more"); } }
public static bool IsValidFirstName(string value) { return !IsEmptyString(value); }
public static bool IsValidLastName(string value) { return !IsEmptyString(value); }
private static bool IsEmptyString(string value) { return value == ""; }
public static bool IsValidStudentID(string value) { bool answer = true; if (value.Length == 9) { foreach (char c in value) { if (!int.TryParse(c.ToString(), out int number)) { answer = false; break; } } } else { answer = false; } return answer; }
public static bool IsValidApplicationDate(DateTime value) { return value.Date == DateTime.Today; }
public static bool IsValidCredits(int value) { return value >= 0; }
public static bool IsValidGPA(decimal value) { return value >= 0; }
public string StudentName(NameOrder format) { string answer=""; switch (format) { case NameOrder.FirstLast: answer = string.Format("{0} {1}", FirstName, LastName); break; case NameOrder.LastFirst: answer = string.Format("{0}, {1}", LastName, FirstName); break; } return answer; }
public override string ToString() { return string.Format("{0} - {1}", StudentName(NameOrder.LastFirst), StudentID); } } }
Solution Explorer Search Solution Explorer (Ctrl+) -Solution Exercise7Solution' (1 project) Exercise7Solution Properties References App.config .; ClassDiagram1.cd DProgram.cS C Student.cs StudentlnfoForm.cs Student!nfoForm.Designer.cs StudentInfoForm.resx Solution Explorer Team Explorer Solution Explorer Search Solution Explorer (Ctrl+) -Solution Exercise7Solution' (1 project) Exercise7Solution Properties References App.config .; ClassDiagram1.cd DProgram.cS C Student.cs StudentlnfoForm.cs Student!nfoForm.Designer.cs StudentInfoForm.resx Solution Explorer Team ExplorerStep by Step Solution
There are 3 Steps involved in it
Step: 1
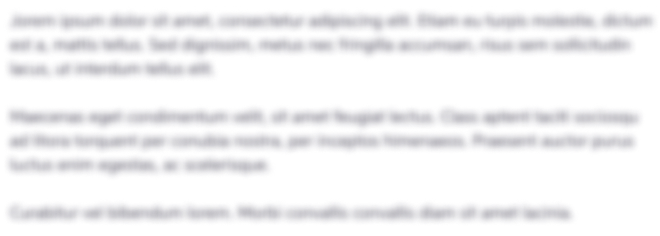
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started