Question
C++ For this assignment you will implement four functions that are defined in myarrayfile.cpp which is provided with this assignment. The description of each of
C++
For this assignment you will implement four functions that are defined in myarrayfile.cpp which is provided with this assignment. The description of each of the functions is defined
in the myarrayfile.cpp file. For this assignment, a supporting myarrayfile.h file must be created. The definition of the functions in myarrayfile.cpp cannot be changed. The four functions in the myarrayfile.cpp file must be implemented. Do not change the getRandomInteger() function in the myarrayfile.cpp. The function prototype for getRandomInteger() needs to be added to the myarrayfile.h file along with the 4 other functions that are being implemented. A Main.cpp file is also provided which contains test cases for the four functions in myarrayfile.cpp.
To receive full credit, the test cases must all pass. A project will be created that contains 3 files (myarrayfile.h, myarrayfile.cpp, and Main.cpp). The myarrayfile.h file and the myarrayfile.cpp file need to be completed to successfully compile with the Main.cpp file. The Main.cpp file will compile without errors or warnings with correctly implemented myarrayfile.h and myarrayfile.cpp files. No other changes should be made to the Main.cpp file.
myarrayfile.cpp
#include "myarrayfile.h"
#include // For time()
#include // For srand() and rand()
// This function will perform a linear search of the input myArray[].
// arrayLength is the number of elements to search for in myArray[].
// If itemToFind is contained in myArray[] at least once then return true.
// If itemToFind is not contained in myArray[] then return false.
// If arrayLength is zero or negative then return false.
// You can only access the bounds of myArray, that is you only access
between
// index 0 and index arrayLength-1.
bool isItemInArray(int myArray[], int arrayLength, int itemToFind)
{
}
// This function will add all the even positive numbers in myArray.
// The length of myArray is defined by arrayLength.
// The value returned is the sum of all the even positive numbers in the
array.
// If there are no positive even integers in the array then return 0.
// If arrayLength is zero or negative then return 0.
// You can only access the bounds of myArray, that is you only access
between
// index 0 and index arrayLength-1.
int addEvenPositive(int myArray[], int arrayLength)
{
}
// This function will find the highest value in myArray.
// The length of myArray is defined by arrayLength.
// The value returned is the highest value in myArray.
// If arrayLength is zero or negative then return 0.
// You can only access the bounds of myArray, that is you only access
between
// index 0 and index arrayLength-1.
int getHighest(int myArray[], int arrayLength)
{
}
// Implement a function that performs a quick pick function for the
Florida Lottery.
// You are to fill myArray with six unique random numbers from 1 to 53.
// All the six numbers must be different.
// You should use getRandomInteger(int) function which is provided to you.
// You can also use isItemInArray() function which is implemented above.
void quickPick(int myArray[])
{
}
// do not make any changes to this function !!!
// getRandomInteger will return a random integer number between 0 and max
int getRandomInteger(int max)
{
static bool initialized = false;
if (initialized == false)
{ // if the first time through then
srand((unsigned)time(0)); // Initialize random number
generator.
initialized = true;
}
int rv = rand() % (max + 1);
return rv;
}
Main.cpp
#include "myarrayfile.h"
#include
bool checkSame(int array1[], int array2[]); // function prototypes for
this file
int checkArray(int array[]); // function prototypes for this file
int main(void)
{
// Put your name in the statement below
std::cout << "Homework 4 test case results by Jane Doe" <<
std::endl;
int testCount = 1; // used to track test numbers
// test cases for the isItemInArray() function
int testArray1[] = {-1, 3, 0, 2, 6};
if (isItemInArray(testArray1, 4, -1) == true)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, 4, 3) == true)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, 4, 0) == true)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, 4, 2) == true)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, 4, 6) == false)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, 4, -2) == false)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, 0, -1) == false)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (isItemInArray(testArray1, -1, -1) == false)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
// test cases for the addEvenPositive() function
int testArray2[] = { -2, 3, 4, 2, 6, 10 };
if (addEvenPositive(testArray2, 5) == 12)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (addEvenPositive(testArray2, 6) == 22)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (addEvenPositive(testArray2, 0) == 0)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (addEvenPositive(testArray2, -1) == 0)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
int testArray9[] = { -2, 3, 5, 1, 7, 10 };
if (addEvenPositive(testArray9, 5) == 0)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
// test cases for the getHighest() function
int testArray3[] = { -3, -4, -14, -10, -2, -1 };
if (getHighest(testArray3, 6) == -1)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (getHighest(testArray3, 5) == -2)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (getHighest(testArray3, 4) == -3)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (getHighest(testArray3, 0) == 0)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
if (getHighest(testArray3, -1) == 0)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
// test cases for the quickPick() function
int myArray1[] = {0,0,0,0,0,0};
int myArray2[] = { 0, 0, 0, 0, 0, 0 };
quickPick(myArray1);
quickPick(myArray2);
if (checkSame(myArray1, myArray2) == false)
std::cout << "Test Case " << testCount << " Passed" <<
std::endl;
else
std::cout << "Test Case " << testCount << " Failed" <<
std::endl;
testCount++;
int error;
for (int i = 0; i < 100; i++)
{
quickPick(myArray1);
error = checkArray(myArray1);
if (error != 0)
{
break;
}
}
if (error == 0)
std::cout << "QuickPick Test Passed " << std::endl;
else if (error == -1)
std::cout << "QuickPick Test Failed - Out of range number
found " << myArray1[0] << " " << myArray1[1] << " " << myArray1[2] << " "
<< myArray1[3] << " " << myArray1[4] << " " << myArray1[5] << std::endl;
else if (error == -2)
std::cout << "QuickPick Test Failed - Duplicate number
found " << myArray1[0] << " " << myArray1[1] << " " << myArray1[2] << " "
<< myArray1[3] << " " << myArray1[4] << " " << myArray1[5] << std::endl;
return 0;
}
bool checkSame(int array1[], int array2[])
{
bool rv = true;
for (int i = 0; i < 6; i++)
{
if (array1[i] != array2[i])
{
rv = false;
}
}
return rv;
}
int checkArray(int array[])
{
int rv = 0;
for (int i = 0; i < 6; i++)
{
if (array[i] < 1 || array[i] > 53)
rv = -1;
}
if (rv == 0)
{
for (int i = 0; i < 6; i++)
{
int count = 0;
for (int j = 0; j < 6; j++)
{
if (array[i] == array[j])
{
count++;
}
}
if (count > 1)
{
rv = -2;
}
}
}
return rv;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
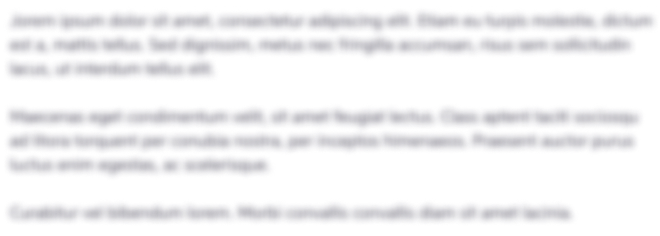
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started