Question
C++ For this project, we turn our attention to arrays, in particular 2D arrays, or grids. Were going to use the grid as a board
C++
For this project, we turn our attention to arrays, in particular 2D arrays, or grids. Were going to use the grid as a board for a game we are going to play. So I put those words in quotes because we wont really be playing the game, well be loading items on the grid and then well let the computer actually control the game. While were doing this well be checking to make sure that the players on the board dont go off the board and dont step on any of the other items on the board.
So the game well be playing a defense game of sorts. Therell be two castles placed on the board and additionally therell be rocks and water on the board. After the board is loaded, the castles will begin to issue and move soldiers from their castle to the opposing players castle. Once the soldiers get next to the opposing teams castle, theyll begin to do a point of damage against the castle. Once one of the castles health reaches 0, that castle has lost. If both castles health get to 0 on the same turn, then it will be considered a draw, and neither castle will have won.
Details
The main storage array or grid will be a fixed 20 rows by 40 columns. I would make it an array of strings, since we will be storing two character data in the array. It is possible to use other data types so if you have another idea, you may use another data type, for example, int.
Some things to note, the location 0, 0 is the top left corner and 19, 39 is the bottom right corner. So when the row numbers increase you are moving down the grid and when the column numbers increase you are moving to the right. This is important because if you reverse this your grid will be flipped.
Another major emphasis of this project is to check the row and column you are about to use to make sure it is at least 0 and not greater than the size of the grid. There are times when the items will always be on the grid, but if you dont check, you wont know. There will be, or I think there should be, large parts of the code that are simply check the row and column to make sure you know how many directions you can go.
Input
The input for this program is a board file that contains a set of information about what is on the board. The order is not guaranteed except that the castles health and the number of soldiers will always be the last 3 lines in the file. The castles may not be the first items in the file and sometimes one or the other, or maybe both, will be missing. The row and column that are given may be too large and need to be checked before you attempt to place the item on the grid. Also, you will only place an item on the grid is the space is empty. This is not a problem if you attempt to place a rock on top of a water, or vice versa, however this is an error if you attempt to place a castle on an already occupied grid square.
Sample Board.txt
C1 9, 5 C2 9, 35 Rock 9, 20 Water 8, 20 Water 10, 20 Rock 9, 34 Rock 10, 30 Rock 8, 34 Rock 7, 30 Rock 11, 30 C1H 90 C2H 100 NumSoldiers 5
So in this input, we have castle 1, C1, being placed at row 9 and column 5. Castle 2, C2, is being placed at row 9 and column 35. The rocks and water are being placed at the coordinates indicated after the words. A rock is, or can be, stored as **, and water is, or can be stored as, ~~. An empty space can be indicated using -. In this way, if a grid space contains a -, then its empty and available to be used. If it doesnt contain a -, then it is occupied. We can use this later when we attempt to issue a soldier and move a soldier.
Errors during loading
There are 3 errors that might occur during loading. If an error occurs, the game is not played. Do not use exit() to stop the game. You will not get a grade from Web-CAT if you use exit().
A castle is missing from the input file.
A castles row/column would place it off the board.
A castle is given a row, column that is already occupied.
Each of these errors has a distinct error message. See the output section below for those messages.
Playing the Game
Assuming no error has occurred in loading the board file, then the computer takes over and plays the game. This will take a very prescribed algorithm, meaning you have to follow the steps correctly to be correct.
After loading, you will display the board as it is loaded.
While either of the castles health is greater than 0 and the step count is less than 1000
If castle 1 has fewer than the number of soldiers its allowed, issue a soldier
If castle 2 has fewer than the number of soldiers its allowed, issue a soldier
For each of the soldiers that castle 1 has issued, move a soldier starting with the first one that was issued and going to the last one issued.
For each of the soldiers that castle 2 has issued, move a soldier starting with the first one that was issued and going to the last one issued.
Display the step count, each iteration is a step, starting with step 1.
Display the current board.
Issuing A Soldier
Each castle will issue a soldier in the direction of the other castle.
Castle 1 will want to issue their soldiers to the right first. Castle 1 will not issue any soldier in a left direction. If right is blocked then it will issue into the next available in a clockwise direction. For example, if a castle 1 is directly to the left of a rock, so soldiers cant issue to the right, then it will try to issue a soldier down and to the right. If that is blocked, then it will attempt to issue a soldier down. If that direction is also blocked, then it will attempt to issue a soldier up. If that were also blocked then finally it would attempt to issue in an up and right direction. See picture below.
4 5 ^ ^ | / C1 -> 1 | \ v v 3 2
Castle 2 will follow a similar pattern but instead will issue soldiers to the left and never to the right.
2 3 ^ ^ \ | 1 <- C2 / | v v 5 4
Theres no guarantee but generally, castle 1 will be on the left half of the grid and castle 2 will be on the right half of the grid. It is not an error if this isnt true.
Additional things to consider, a castle might be on an edge, so in those cases, it cant issue soldiers in that direction at all. For example, if castle 1 were in the top left corner, it could only issue soldiers right, down right and down. If castle 2 is in the top left corner, it could only issue soldiers in a left, down, and down left directions. The order doesnt change, just the potential places where it might be able to issue. This is why its important to know where an item is an to know which ways its allowed to go. If a way is blocked, then its simply out of the running as a potential place to go.
If a soldier cannot issue, this isnt an error, it simply doesnt issue.
Moving a soldier
Moving a soldier is similar to issuing a soldier except, the grid location that is chosen it based on which grid is closet to the other castle. Castle 1 soldiers will never move in a left direction and castle 2 soldiers will never move in a right direction. I did this for simplicity and to reduce the amount of code you are writing. It does cause some weird movements if a soldier gets stuck, we are simply going to ignore this.
So from where a soldier is, you need to figure out the distance from the grids around it to the other castle, then choose the grid that is closest. We will use the same pattern to choose as we did for issuing. If a grid is blocked by a rock, water, soldier, or edge, then it is out of the running, and we must choose another grid cell instead. If a soldier gets stuck, then it simply doesnt move.
I suggest you use another 2D array to store the row and column of each soldier. For example, you can make a 2D array of size, 5x2 of type int. So row 0 is the first soldier and column 0 might be its row, then row 0 and column 1 would be the soldiers column. There are other ways to store this and this is one reason Ive limited the max number of soldiers to 5.
Output
If there are errors during loading, this is what you would say for each condition:
Error: during loading, one of the castles was missing from the input file.
Error: during loading, one of the castles was placed on a covered cell.
Error: during loading, one of the castles was off the board.
Ill leave it up to you to decide which message goes with which error. If you have questions, please ask, but lets use some context clues to decide which one makes the most sense to go with which error.
Displaying the board
Each time you display the board it will look something like this:
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 0 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 1 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 2 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 3 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 4 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 5 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 6 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 7 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ** - - - - - - - - - 8 - - - - - - - - - - - - - - - - - - - - ~~ - - - - - - - - - - - - - ** - - - - - 9 - - - - - C1 - - - - - - - - - - - - - - ** - - - - - - - - - - - - - ** C2 - - - - 10 - - - - - - - - - - - - - - - - - - - - ~~ - - - - - - - - - ** - - - - - - - - - 11 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ** - - - - - - - - - 12 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 13 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 14 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 15 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 16 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 17 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 18 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 19 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Castle 1 >> Health: 90 >> Soldiers: 0 ~~**~~ Castle 2 >> Health: 100 >> Soldiers: 0
This is the initial display after loading the board from the sample in the input. The bottom line is a status line of sorts that indicates the castle, its health, and the number of soldiers it currently has on the board.
All subsequent outputs will have the step first and then the display of the board. For example, this is step 1 of the game after the loading:
Step: 1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 0 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 1 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 2 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 3 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 4 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 5 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 6 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 7 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ** - - - 2S - - - - - 8 - - - - - - - - - - - - - - - - - - - - ~~ - - - - - - - - - - - - - ** - - - - - 9 - - - - - C1 - 1S - - - - - - - - - - - - ** - - - - - - - - - - - - - ** C2 - - - - 10 - - - - - - - - - - - - - - - - - - - - ~~ - - - - - - - - - ** - - - - - - - - - 11 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ** - - - - - - - - - 12 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 13 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 14 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 15 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 16 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 17 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 18 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 19 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Castle 1 >> Health: 90 >> Soldiers: 1 ~~**~~ Castle 2 >> Health: 100 >> Soldiers: 1
So in this case, it might look like each soldier moved 2 spaces, but what happened is a soldier was issued, and then it moved. So when a soldier is issued, it is allowed to move on the same turn or step. So castle 1 issued a soldier to 9, 6 and then it moved to 9, 7 since that was the grid that was closest to castle 2. Castle 2 issue a soldier to 8, 35, since 9, 34 and 8, 34 were blocked, then it moved to 7, 34 since it was the closest grid to castle 1. Had 8, 35 been blocked, then the soldier would have tried 10, 35.
This game would continue until either 1000 steps are taken or one of the castles health reaches 0. Below is the final step output:
Step: 55 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 0 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 1 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 2 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 3 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 4 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 5 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 6 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 7 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ** - - - - - - - - - 8 - - - - - 2S 2S - - - - - - - - - - - - - ~~ - - - - - - - - - - - - - ** - - - - - 9 - - - - - C1 2S 2S - - - - - - - - - - - - ** - - - - - - - - - - - - - ** C2 - 1S - - 10 - - - - - - 2S - - - - - - - - - - - - - ~~ - - - - - - - - - ** - - 1S 1S 1S 1S - - - 11 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ** - - - - - - - - - 12 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 13 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 14 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 15 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 16 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 17 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 18 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 19 - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Castle 1 >> Health: 0 >> Soldiers: 5 ~~**~~ Castle 2 >> Health: 30 >> Soldiers: 5 Castle 2 won. It's health was: 30
So this game ended on step 55. The last line of the output will indicate which castle won and the remaining health of the winning castle.
In the case of the draw, then you will simply say Draw followed by castle 1s health and castle 2s health. In the case when the steps reaches 1000, then this is also the output that would happen, since neither castle won.
Requirements
The following requirements will be assessed by the TAs and I for full credit.
You must declare the function void defense( string input, string output ); in a file named castle.h
You must implement the function from requirement 1, in a separate cpp file. This is the primary function that plays the game.
You must use other functions are you see fit. You must have at a minimum 3 more functions other than the function from requirement 1.
For example, I have a functions for loading the board, issuing a soldier, moving a soldier, displaying the board, computing the distance, finding items on the grid. These are not required functions but 6 examples of functions.
You may not use a vector, list, or other storage container from the STL.
You must use a 2D array of size 20 by 40 to store the grid. It should probably be a string, but other data types are okay. It may also be a 3D array if you want.
An additional 2D array of 5 by 2 is suggested to store the soldiers locations, but this is not required.
You must follow the code style guidelines as outlined in Canvas.
No global variable.
No classes, structs, pointers.
Grading
Zip up your castle.h and your cpp file(s) and submit them to Web-CAT for grading.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
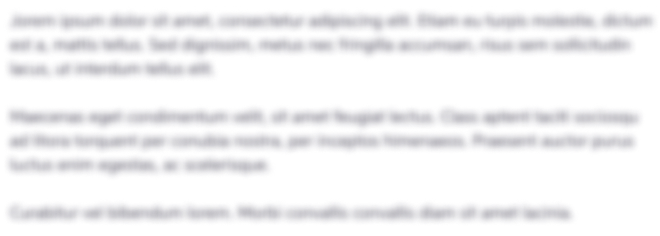
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started