Question
C++ Game of life (basically) need help with last 3 functions in the implementation file. Code and instructions below. Can't change the .h file. Function:
C++ Game of life (basically) need help with last 3 functions in the implementation file. Code and instructions below. Can't change the .h file.
Function: getLiveNeighbors This function has two int parameters, a row and a column. Each cell in the grid has from three to eight neighbors (adjacent cells). This function must return the number of neighbors that contain the value LIVE. Remember that many cells don't have all eight neighbors. For example, the cell in row 0, column 0, has only three neighbors. Many of its possible neighbors ((row-1, column-1), (row-1, column), (row-1 column+1), (row, column-1), (row+1, column-1)) do not exist because those coordinates are outside the bounds of the grid. You can use the isValidCell function to test for that.
Function: nextGeneration This function has no parameters. This function returns an int. The purpose of this function is to modify the grid to represent the next generation. Here's how you are supposed to do that for this assignment: Set each element of the count array to the number of live neighbors that the corresponding element in the grid array has. For each element in the grid array, set it according to the following rules: If the cell was LIVE and has less than two or more than three live neighbors, then change it to DEAD. If the cell was DEAD and has exactly three live neighbors, then change it to LIVE. Add one to the generation. Return the value of the generation instance variable.
Function: operator<< This function has two parameters, a reference to an ostream, and a reference to an automata object. Display the grid to the ostream using one row of the grid per output line. Return the ostream reference passed to this function.
Notes Once compiled, you can run the program using: ./auto2d The count array would normally be declared as a local variable inside the nextGeneration method since that is the only method that uses it. Its elevation in this case to an instance variable is poor coding style.
************************code*************************
#include "io.h" #include "automata.h"
automata::automata() { reset(); }
void automata::reset() { for (int r = 0; r < ROWS; r++) { for ( int c = 0; c < COLS; c++) { grid[r][c] = DEAD; } } generation == 0; }
void automata::setCell(const int r, const int col) { if (isValidCell = true) { setCell[r][col] = LIVE; } }
void automata::clearCell(const int r, const int col) { if (isValidCell = true) { setCell[r][col] = DEAD; } }
bool automata::isValidCell(const int r, const int c) const { return 0 <= r && r <= ROWS-1 && 0 <= c && c <= COLS-1; }
int automata::getGeneration() const { return generation; }
int automata::getLiveNeighbors(const int r, const int c) const {
}
int automata::nextGeneration() {
}
ostream& operator<<(ostream& strm, const automata& brd) {
}
*****************.h file for ref.*******************
#ifndef __AUTOMATA_H__ #define __AUTOMATA_H__ #includeusing std::ostream; class automata { private: static const char LIVE = '*'; static const char DEAD = ' '; static const int ROWS = 20; static const int COLS= 60; int generation; char grid[ROWS][COLS]; int count[ROWS][COLS]; public: automata(); void reset(); void setCell(const int r, const int col); void clearCell(const int r, const int col); bool isValidCell(const int r, const int c) const; int getLiveNeighbors(const int r, const int c) const; int getGeneration() const; int nextGeneration(); friend ostream& operator<<(ostream& strm, const automata& brd); }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
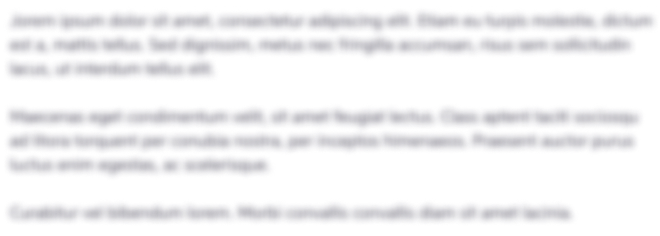
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started