Question
C++ help please: 13. a. Define the class bankAccount to store a bank customers account number and balance. Suppose that account number is of type
C++ help please:
13. a. Define the class bankAccount to store a bank customers account number and balance. Suppose that account number is of type int, and balance is of type double. Your class should, at least, provide the following operations: set the account number, retrieve the account number, retrieve the balance, deposit and withdraw money, and print account information. Add appropriate constructors.
b. Every bank offers a checking account. Derive the class checkingAccount from the class bankAccount (designed in part (a)). This class inherits members to store the account number and the balance from the base class. A customer with a checking account typically receives interest, maintains a minimum balance, and pays service charges if the balance falls below the minimum balance. Add member variables to store this additional information. In addition to the operations inherited from the base class, this class should provide the following operations: set interest rate, retrieve interest rate, set minimum balance, retrieve minimum balance, set service charges, retrieve service charges, post interest, verify if the balance is less than the minimum balance, write a check, withdraw (override the method of the base class), and print account information. Add appropriate constructors.
c. Every bank offers a savings account. Derive the class savingsAccount from the class bankAccount (designed in part (a)). This class inherits members to store the account number and the balance from the base class. A customer with a savings account typically receives interest, makes deposits, and withdraws money. In addition to the operations inherited from the base class, this class should provide the following operations: set interest rate, retrieve interest rate, post interest, withdraw (override the method of the base class), and print account information. Add appropriate constructors.
d. Write a program to test your classes designed in parts (b) and (c).
Follow this template exactly and fill in where it says "TODO" please:
------------------------------------------------------------------------------------------------
bankAccount.h
//Class bankAccount
#ifndef H_bankAccount
#define H_bankAccount
class bankAccount
{
public:
void setAccountNumber(int acct);
int getAccountNumber() const;
double getBalance() const;
void withdraw(double amount);
void deposit(double amount);
void print() const;
bankAccount(int acctNumber = -1, double bal = 0);
protected:
int accountNumber;
double balance;
};
#endif
---------------------------------------------------------------
bankAccountImp.cpp
//Implementation file bankAccountImp.cpp
#include
#include
#include "bankAccount.h"
using namespace std;
bankAccount::bankAccount(int acctNumber, double bal)
{
// TODO
}
// TODO: add the class implementation here!
----------------------------------------------------------------------
checkingAccount.h
//Class checkingAccount
#ifndef H_checkingAccount
#define H_checkingAccount
#include "bankAccount.h"
const double DEFAULT_INTEREST_RATE_CHECKING = 0.04;
const double DEFAULT_MIN_BALANCE = 500.00;
const double DEFAULT_SERVICE_CHARGE = 20;
class checkingAccount: public bankAccount
{
public:
double getMinimumBalance() const;
void setMinimumBalance(double minBalance);
double getInterestRate() const;
void setInterestRate(double intRate);
double getServiceCharge() const;
void setServiceCharge(double intRate);
void postInterest();
bool verifyMinimumumBalance(double amount);
void writeCheck(double amount);
void withdraw(double amount);
void print() const;
checkingAccount(int acctNumber, double bal,
double minBal = DEFAULT_MIN_BALANCE,
double intRate = DEFAULT_INTEREST_RATE_CHECKING,
double servC = DEFAULT_SERVICE_CHARGE);
protected:
double interestRate;
double minimumBalance;
double serviceCharge;
};
#endif
---------------------------------------------------------------------
checkingAccountImp.cpp
//Implementation file checkingAccountImp.cpp
#include
#include
#include "checkingAccount.h"
using namespace std;
checkingAccount::checkingAccount(int acctNumber, double bal,
double minBal, double intRate, double servC)
: bankAccount(acctNumber, bal)
{
// TODO
}
// TODO: Add the class implementation here (See the class definition in checking account.h)!
------------------------------------------------------------------------------------
savingsAccount.h
//Class savingsAccount
#ifndef H_savingsAccount
#define H_savingsAccount
#include "bankAccount.h"
const double DEFAULT_INTEREST_RATE_SAVINGS = 0.06;
class savingsAccount: public bankAccount
{
public:
double getInterestRate() const;
void setInterestRate(double rate);
void withdraw(double amount);
void postInterest();
void print() const;
savingsAccount(int acctNumber, double bal,
double intRate = DEFAULT_INTEREST_RATE_SAVINGS);
protected:
double interestRate;
};
#endif
------------------------------------------------------------------------
savingsAccountImp.cpp
//Implementation File savingsAccountImp.cpp
#include
#include
#include "savingsAccount.h"
using namespace std;
savingsAccount::savingsAccount(int acctNumber, double bal, double intRate)
:bankAccount(acctNumber, bal)
{ // TODO
}
double savingsAccount::getInterestRate() const
{// TODO
}
void savingsAccount::setInterestRate(double rate)
{ // TODO
}
void savingsAccount::withdraw(double amount)
{ // TODO
}
void savingsAccount::postInterest()
{ // TODO
}
void savingsAccount::print() const
{ // TODO
}
-----------------------------------------------------------------------------
main.cpp
#include
#include
#include "savingsAccount.h"
#include "checkingAccount.h"
using namespace std;
int main()
{
int accountNumber = 1000;
checkingAccount jackAccount(accountNumber++,1000);
checkingAccount lisaAccount(accountNumber++, 450);
savingsAccount samirAccount(accountNumber++, 9300);
savingsAccount ritaAccount(accountNumber++, 32);
jackAccount.deposit(1000);
lisaAccount.deposit(2300);
samirAccount.deposit(800);
ritaAccount.deposit(500);
jackAccount.postInterest();
lisaAccount.postInterest();
samirAccount.postInterest();
ritaAccount.postInterest();
cout << "***********************************" << endl;
jackAccount.print();
lisaAccount.print();
samirAccount.print();
ritaAccount.print();
cout << "***********************************" << endl << endl;
jackAccount.writeCheck(250);
lisaAccount.writeCheck(350);
samirAccount.withdraw(120);
ritaAccount.withdraw(290);
cout << "********After withdrawals ***************" << endl;
jackAccount.print();
lisaAccount.print();
samirAccount.print();
ritaAccount.print();
cout << "***********************************" << endl << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
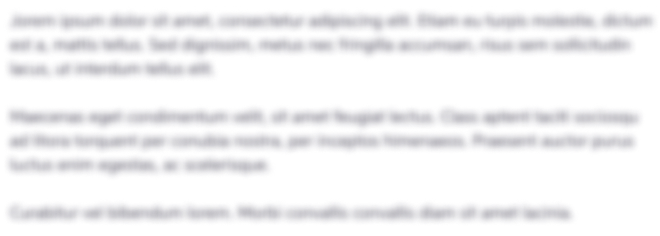
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started