Question
C++ Help We will define a ring to be sorted bi-directional circular list that is implemented using links. Each node in the ring will have
C++ Help
We will define a ring to be sorted bi-directional circular list that is implemented using links. Each node in the ring will have a backward and forward link and a data entry. The ring will be sorted in ascending order following the forward links. Of course, since the ring is circular, there is a discontinuity in the sort at the head (the start/end point) of the ring. Create a templated ring class along with functions for inserting and deleting data, and a function that displays the contents of the ring. Will need to update a few portions of the code so that it supports bi-directional linked lists. Write a driver that inserts 50 unique random ints into a ring, displays the ring in both forward and backward directions by following the links, and then deletes all of the odd values that were inserted. Perform the deletions by saving a list of the odd values (say, in a vector or array) and then using your delete function dont write a special function that traverses the ring, deleting odd values as it goes. Should your delete function follow the forward or backward links when deleting? Display the ring again in both forward and backwards directions after performing the deletions.
********************************************************************************
LinkedSortedList.h
********************************************************************************
/** ADT sorted list: Link-based implementation.
@file LinkedSortedList.h */
#pragma warning(disable : 4290)
#pragma once
#include "SortedListInterface.h"
#include "Node.h"
#include "PrecondViolatedExcep.h"
template
class LinkedSortedList : public SortedListInterface
{
private:
Node
int itemCount; // Current count of list items
// Locates the node that is before the node that should or does
// contain the given entry.
// @param anEntry The entry to find.
// @return Either a pointer to the node before the node that contains
// or should contain the given entry, or nullptr if no prior node exists.
Node
// Locates the node at a given position within the chain.
Node
// Returns a pointer to a copy of the chain to which origChainPtr points.
Node
public:
LinkedSortedList();
LinkedSortedList(const LinkedSortedList
virtual ~LinkedSortedList();
void insertSorted(const ItemType& newEntry);
bool removeSorted(const ItemType& anEntry);
int getPosition(const ItemType& newEntry) const;
// The following methods are the same as given in ListInterface:
bool isEmpty() const;
int getLength() const;
bool remove(int position);
void clear();
ItemType getEntry(int position) const throw(PrecondViolatedExcep);
}; // end LinkedSortedList
#include "LinkedSortedList.cpp"
********************************************************************************
Node.h
********************************************************************************
/** @file Node.h
Listing 4-1 */
#pragma once
template
class Node
{
private:
ItemType item; // A data item
Node
public:
Node();
Node(const ItemType& anItem);
Node(const ItemType& anItem, Node
void setItem(const ItemType& anItem);
void setNext(Node
ItemType getItem() const;
Node
}; // end Node
#include "Node.cpp"
********************************************************************************
PrecondViolatedExcep.h
********************************************************************************
/** Listing 7-5.
@file PrecondViolatedExcep.h */
#pragma once
#include
#include
using namespace std;
class PrecondViolatedExcep : public logic_error
{
public:
PrecondViolatedExcep(const string& message = "");
}; // end PrecondViolatedExcep
********************************************************************************
SortedListInterface.h
********************************************************************************
/** Interface for the ADT sorted list
@file SortedListInterface.h */
#pragma once
template
class SortedListInterface
{
public:
/** Inserts an entry into this sorted list in its proper order
so that the list remains sorted.
@pre None.
@post newEntry is in the list, and the list is sorted.
@param newEntry The entry to insert into the sorted list. */
virtual void insertSorted(const ItemType& newEntry) = 0;
/** Removes the first or only occurrence of the given entry from this
sorted list.
@pre None.
@post If the removal is successful, the first occurrence of the
given entry is no longer in the sorted list, and the returned
value is true. Otherwise, the sorted list is unchanged and the
returned value is false.
@param anEntry The entry to remove.
@return True if removal is successful, or false if not. */
virtual bool removeSorted(const ItemType& anEntry) = 0;
/** Gets the position of the first or only occurrence of the given
entry in this sorted list. In case the entry is not in the list,
determines where it should be if it were added to the list.
@pre None.
@post The position where the given entry is or belongs is returned.
The sorted list is unchanged.
@param anEntry The entry to locate.
@return Either the position of the given entry, if it occurs in the
sorted list, or the position where the entry would occur, but as a
negative integer. */
virtual int getPosition(const ItemType& anEntry) const = 0;
// The following methods are the same as those given in ListInterface
// in Listing 8-1 of Chapter 8 and are completely specified there.
/** Sees whether this list is empty. */
virtual bool isEmpty() const = 0;
/** Gets the current number of entries in this list. */
virtual int getLength() const = 0;
/** Removes the entry at a given position from this list. */
virtual bool remove(int position) = 0;
/** Removes all entries from this list. */
virtual void clear() = 0;
/** Gets the entry at the given position in this list. */
virtual ItemType getEntry(int position) const = 0;
}; // end SortedListInterface
********************************************************************************
LinkedSortedList.cpp
********************************************************************************
/** Implementation file for the class LinkedSortedList.
@file LinkedSortedList.cpp */
#include "LinkedSortedList.h" // Header file
#include
template
LinkedSortedList
{
} // end default constructor
template
LinkedSortedList
{
headPtr = copyChain(aList.headPtr);
itemCount = aList.itemCount;
} // end copy constructor
template
Node
{
Node
if (origChainPtr != nullptr)
{
// Build new chain from given one
copiedChainPtr = new Node
copiedChainPtr->setNext(copyChain(origChainPtr->getNext()));
} // end if
return copiedChainPtr;
} // end copyChain
template
LinkedSortedList
{
clear();
} // end destructor
template
void LinkedSortedList
{
Node
Node
if (isEmpty() || (prevPtr == nullptr)) // Add at beginning
{
newNodePtr->setNext(headPtr);
headPtr = newNodePtr;
}
else // Add after node before
{
Node
newNodePtr->setNext(aftPtr);
prevPtr->setNext(newNodePtr);
} // end if
itemCount++;
} // end insertSorted
template
bool LinkedSortedList
{
bool ableToDelete = false;
if (!isEmpty())
{
Node
Node
if (prevPtr != nullptr)
nodeToRemovePtr = prevPtr->getNext();
ableToDelete = (nodeToRemovePtr != nullptr) && (anEntry == nodeToRemovePtr->getItem());
if (ableToDelete)
{
Node
if (nodeToRemovePtr == headPtr)
{
// Delete the first node in the chain
headPtr = aftPtr;
}
else
{
// Disconnect indicated node from chain by connecting the
// prior node with the one after
prevPtr->setNext(aftPtr);
} // end if
// Return deleted node to system
nodeToRemovePtr->setNext(nullptr);
delete nodeToRemovePtr;
nodeToRemovePtr = nullptr;
itemCount--; // Decrease count of entries
} // end if
} // end if
return ableToDelete;
} // end removeSorted
template
int LinkedSortedList
{
int position = 1;
Node
while ( (curPtr != nullptr) && (anEntry > curPtr->getItem()) )
{
curPtr = curPtr->getNext();
position++;
} // end while
if ( (curPtr == nullptr) || (anEntry != curPtr->getItem()) )
position = -position;
return position;
} // end getPosition
//=====================
// List operations:
template
bool LinkedSortedList
{
bool ableToDelete = (position >= 1) && (position <= itemCount);
if (ableToDelete)
{
Node
if (position == 1)
{
// Delete the first node in the chain
curPtr = headPtr; // save pointer to node
headPtr = headPtr->getNext();
}
else
{
// Find node that is before the one to delete
Node
// Point to node to delete
curPtr = prevPtr->getNext();
// Disconnect indicated node from chain by connecting the
// prior node with the one after
prevPtr->setNext(curPtr->getNext());
} // end if
// Return deleted node to system
curPtr->setNext(nullptr);
delete curPtr;
curPtr = nullptr;
itemCount--; // Decrease count of entries
} // end if
return ableToDelete;
} // end remove
template
void LinkedSortedList
{
while (!isEmpty())
remove(1);
} // end clear
template
ItemType LinkedSortedList
{
// Enforce precondition
bool ableToGet = (position >= 1) && (position <= itemCount);
if (ableToGet)
{
Node
return nodePtr->getItem();
}
else
{
string message = "getEntry() called with an empty list or ";
message = message + "invalid position.";
throw(PrecondViolatedExcep(message));
} // end if
} // end getEntry
template
bool LinkedSortedList
{
return itemCount == 0;
} // end isEmpty
template
int LinkedSortedList
{
return itemCount;
} // end getLength
template
Node
{
Node
Node
while ( (curPtr != nullptr) && (anEntry > curPtr->getItem()) )
{
prevPtr = curPtr;
curPtr = curPtr->getNext();
} // end while
return prevPtr;
} // end getNodeBefore
template
Node
{
assert( (position >= 1) && (position <= itemCount) );
// Count from the beginning of the chain
Node
for (int skip = 1; skip < position; skip++)
curPtr = curPtr->getNext();
return curPtr;
} // end getNodeAt
// End of implementation file.
********************************************************************************
main.cpp
********************************************************************************
#include
#include
#include "LinkedSortedList.h" // ADT list operations
using namespace std;
void displayList(SortedListInterface
{
cout << "The sorted list contains " << endl;
for (int pos = 1; pos <= listPtr->getLength(); pos++)
{
cout << listPtr->getEntry(pos) << " ";
} // end for
cout << endl << endl;
} // end displayList
void copyConstructorTester()
{
LinkedSortedList
string items[] = { "zero", "one", "two", "three", "four", "five" };
for (int i = 0; i < 6; i++)
{
cout << "Adding " << items[i] << endl;
list.insertSorted(items[i]);
}
displayList(&list);
LinkedSortedList
cout << "Copy of list: ";
displayList(©OfList);
cout << "The copied list: ";
displayList(&list);
} // end copyConstructorTester
void sortedListTester(SortedListInterface
{
string luke = "Luke";
string brent = "Brent";
string donna = "Donna";
string tom = "Tom";
string sue = "Sue";
string jerry = "Jerry";
cout << " Test isEmpty with an empty list:" << endl;
if (listPtr->isEmpty())
cout << "OK" << endl;
else
cout << "isEmpty() error" << endl;
listPtr->insertSorted(luke);
listPtr->insertSorted(brent);
listPtr->insertSorted(donna);
listPtr->insertSorted(tom);
listPtr->insertSorted(sue);
listPtr->insertSorted(jerry);
// display the list
cout << "List should contain Brent, Donna, " <<
"Jerry, Luke, Sue, Tom" << endl;
cout << " List actually contains:" << endl;
displayList(listPtr);
cout << endl;
// test getPosition
cout << " Test getPosition: " << endl;
// These names are in the list
cout << "Brent is at position " << listPtr->getPosition(brent) << endl;
cout << "Donna is at position " << listPtr->getPosition(donna) << endl;
cout << "Jerry is at position " << listPtr->getPosition(jerry) << endl;
cout << "Luke is at position " << listPtr->getPosition(luke) << endl;
cout << "Sue is at position " << listPtr->getPosition(sue) << endl;
cout << "Tom is at position " << listPtr->getPosition(tom) << endl;
// These names are not in the list
string missy = "Missy";
cout << "Missy belongs at position " << listPtr->getPosition(missy) << endl;
string zeke = "Zeke";
cout << "Zeke belongs at position " << listPtr->getPosition(zeke) << endl;
string aaron = "Aaron";
cout << "Aaron belongs at position " << listPtr->getPosition(aaron) << endl;
// test getLength and getEntry
cout << " Test getLength and getEntry: " << endl;
cout << " List has " << listPtr->getLength() << " entries, as follows: " << endl;
for (int i = 1; i <= listPtr->getLength(); i++)
cout << i << ": " << listPtr->getEntry(i) << endl;
// test remove
cout << " Test remove: " << endl;
// remove first entry
cout << " Removing first item (Brent): " << listPtr->removeSorted(brent) << "; should be 1 (true)" << endl;
cout << " After removing Brent, list contains " << listPtr->getLength()
<< " items, as follows:" << endl;
displayList(listPtr);
// remove interior
cout << " Removing interior item (Luke): " << listPtr->removeSorted(luke) << "; should be 1 (true)" << endl;
cout << " After removing Luke, list contains " << listPtr->getLength()
<< " items, as follows:" << endl;
displayList(listPtr);
// remove last
cout << &" Removing last item (Tom): "[listPtr->removeSorted(tom)] << "; should be 1 (true)" << endl;
cout << " After removing last item, list contains " << listPtr->getLength()
<< " items, as follows:" << endl;
displayList(listPtr);
cout << " Removing a missing item (Brent): " << listPtr->removeSorted(brent) << "; should be 0 (false)" << endl;
cout << " Removing a missing item (Luke): " << listPtr->removeSorted(luke) << "; should be 0 (false)" << endl;
cout << " Removing a missing item (Tom): " << listPtr->removeSorted(tom) << "; should be 0 (false)" << endl;
cout << " The list contains " << listPtr->getLength()
<< " items, as follows:" << endl;
displayList(listPtr);
// test clear()
cout << " Test clear(): " << endl;
listPtr->clear();
if (listPtr->isEmpty())
cout << " The list is empty after invoking clear()." << endl;
else
cout << " clear() error" << endl;
} // end sortedListTester
void listOpsTester(SortedListInterface
{
string luke = "Luke";
string brent = "Brent";
string donna = "Donna";
string tom = "Tom";
string sue = "Sue";
string jerry = "Jerry";
listPtr->insertSorted(luke);
listPtr->insertSorted(brent);
listPtr->insertSorted(donna);
listPtr->insertSorted(tom);
listPtr->insertSorted(sue);
listPtr->insertSorted(jerry);
displayList(listPtr);
cout << "isEmpty: returns " << listPtr->isEmpty() << "; should be 0 (false)" << endl;
cout << "getLength returns : " << listPtr->getLength() << "; should be 6" << endl;
cout << "remove(2): returns " << listPtr->remove(2) << "; should be 1 (true)" << endl;
cout << "remove(1): returns " << listPtr->remove(1) << "; should be 1 (true)" << endl;
cout << "remove(6): returns " << listPtr->remove(6) << "; should be 0 (false)" << endl;
displayList(listPtr);
cout << "getLength returns : " << listPtr->getLength() << "; should be 4" << endl;
cout << "clear: " << endl;
listPtr->clear();
cout << "isEmpty: returns " << listPtr->isEmpty() << "; should be 1 (true)" << endl;
try
{
cout << "Attempt an illegal retrieval from position 6: " << endl;
listPtr->getEntry(6);
}
catch (PrecondViolatedExcep e)
{
cout << e.what() << endl;
} // end try/catch
} // end listOpsTester
int main()
{
cout << " Linked Sorted List ";
copyConstructorTester();
SortedListInterface
cout << "Testing the Link-Based Sorted List:" << endl;
sortedListTester(listPtr);
cout << "=========================================" << endl;
cout << " Testing the List Operations:" << endl;
listOpsTester(listPtr);
cout << " Fin ";
return 0;
} // end main
/*
Linked Sorted List
Adding zero
Adding one
Adding two
Adding three
Adding four
Adding five
The sorted list contains
five four one three two zero
Copy of list: The sorted list contains
five four one three two zero
The copied list: The sorted list contains
five four one three two zero
Testing the Link-Based Sorted List:
Test isEmpty with an empty list:
OK
List should contain
Brent, Donna, Jerry, Luke, Sue, Tom
List actually contains:
The sorted list contains
Brent Donna Jerry Luke Sue Tom
Test getPosition:
Brent is at position 1
Donna is at position 2
Jerry is at position 3
Luke is at position 4
Sue is at position 5
Tom is at position 6
Missy belongs at position -5
Zeke belongs at position -7
Aaron belongs at position -1
Test getLength and getEntry:
List has 6 entries, as follows:
1: Brent
2: Donna
3: Jerry
4: Luke
5: Sue
6: Tom
Test remove:
Removing first item (Brent): 1; should be 1 (true)
After removing Brent, list contains 5 items, as follows:
The sorted list contains
Donna Jerry Luke Sue Tom
Removing interior item (Luke): 1; should be 1 (true)
After removing Luke, list contains 4 items, as follows:
The sorted list contains
Donna Jerry Sue Tom
Removing last item (Tom): ; should be 1 (true)
After removing last item, list contains 3 items, as follows:
The sorted list contains
Donna Jerry Sue
Removing a missing item (Brent): 0; should be 0 (false)
Removing a missing item (Luke): 0; should be 0 (false)
Removing a missing item (Tom): 0; should be 0 (false)
The list contains 3 items, as follows:
The sorted list contains
Donna Jerry Sue
Test clear():
The list is empty after invoking clear().
=========================================
Testing the List Operations:
The sorted list contains
Brent Donna Jerry Luke Sue Tom
isEmpty: returns 0; should be 0 (false)
getLength returns : 6; should be 6
remove(2): returns 1; should be 1 (true)
remove(1): returns 1; should be 1 (true)
remove(6): returns 0; should be 0 (false)
The sorted list contains
Jerry Luke Sue Tom
getLength returns : 4; should be 4
clear:
isEmpty: returns 1; should be 1 (true)
Attempt an illegal retrieval from position 6:
Precondition Violated Exception: getEntry() called with an empty list or invalid
position.
Fin
*/
********************************************************************************
Node.cpp
********************************************************************************
/** @file Node.cpp
Listing 4-2 */
#include
#include "Node.h"
template
Node
{
} // end default constructor
template
Node
{
} // end constructor
template
Node
item(anItem), next(nextNodePtr)
{
} // end constructor
template
void Node
{
item = anItem;
} // end setItem
template
void Node
{
next = nextNodePtr;
} // end setNext
template
ItemType Node
{
return item;
} // end getItem
template
Node
{
return next;
} // end getNext
********************************************************************************
PrecondViolatedExcep.cpp
********************************************************************************
/** Listing 7-6.
@file PrecondViolatedExcep.cpp */
#include "PrecondViolatedExcep.h"
PrecondViolatedExcep::PrecondViolatedExcep(const string& message) : logic_error("Precondition Violated Exception: " + message)
{
} // end constructor
// End of implementation file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
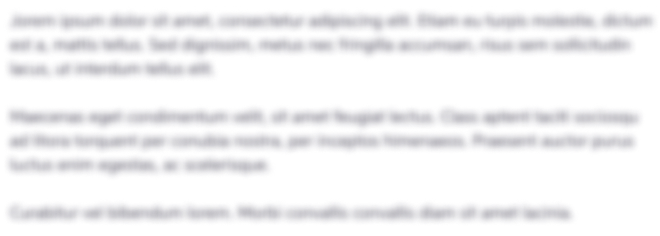
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started