Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// C++ homework // The homework is to complete below in the stack.h : // 1. the copy constructor // 2. the assignment operator //
// C++ homework
// The homework is to complete below in the stack.h : // 1. the copy constructor // 2. the assignment operator // 3. the destructor // 4. Write a test program (mytest.cpp) to test copy and assignment // 5. Verify destructor by running the test program in Valgrind // This is the main.cpp #include#include "stack.h" using namespace std; int main() { Stack intStack; cout << " Push integers on stack and dump contents: "; for (int i = 1; i <= 10; i++) { intStack.push(i); } intStack.dump(); cout << " Read contents using top() and pop(): "; while ( not intStack.empty() ) { cout << intStack.top() << endl; intStack.pop(); } cout << " Attempt pop() of empty stack: "; try { intStack.pop(); } catch (exception &e) { cout << "Caught exception: " << e.what() << endl; } return 0; }
// This is stack.h #ifndef _STACK_H #define _STACK_H #include#include using std::ostream; using std::cout; using std::endl; using std::range_error; // Forward declarations template class Stack; template class Node; template ostream& operator<<(ostream&, const Node &); // Node class for linked list template class Node { friend Stack ; public: Node(T data = T(), Node *next = nullptr) { _data = data; _next = next; } friend ostream& operator<< (ostream &sout, const Node &x); private: T _data; Node *_next; }; // Overloaded insertion operator. Must be overloaded for the template // class T, or this won't work! template ostream& operator<<(ostream &sout, const Node &x) { sout << "Data: " << x._data; return sout; } // Stack class. Linked-list implementation of a stack. Uses the Node // class. template class Stack { public: // Constructor Stack(); // Copy constructor, assignment operator, and destructor // DO NOT IMPLEMENT HERE. SEE BELOW. Stack(const Stack &rhs); const Stack& operator=(const Stack& rhs); ~Stack(); // Stack operations: push(), top(), and pop() void push(const T& data); const T& top() const; void pop(); // Helpful functions bool empty() const; // Returns 'true' if stack is empty void dump() const; // Dump contents of the linked list private: Node *_head; // *************************************************** // Any private helper functions must be delared here! // *************************************************** }; template Stack ::Stack() { _head = nullptr; } template Stack ::Stack(const Stack & rhs) { // ******************************** // Implement the copy constructor // ******************************** } template const Stack & Stack ::operator=(const Stack & rhs) { // ********************************** // Implement the assignment operator // ********************************** } template Stack ::~Stack() { // ************************* // Implement the destructor // ************************* } template void Stack ::push(const T& data) { Node *tmpPtr = new Node (data); tmpPtr->_next = _head; _head = tmpPtr; } template const T& Stack ::top() const { if ( empty() ) { throw range_error("Stack ::top(): attempt to read empty stack."); } return _head->_data; } template void Stack ::pop() { if ( empty() ) { throw range_error("Stack ::pop(): attempt to pop from an empty stack."); } Node *tmpPtr = _head->_next; delete _head; _head = tmpPtr; } template bool Stack ::empty() const { return _head == nullptr; } template void Stack ::dump() const { Node *nodePtr = _head; while ( nodePtr != nullptr ) { cout << nodePtr->_data << endl; nodePtr = nodePtr->_next; } } #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
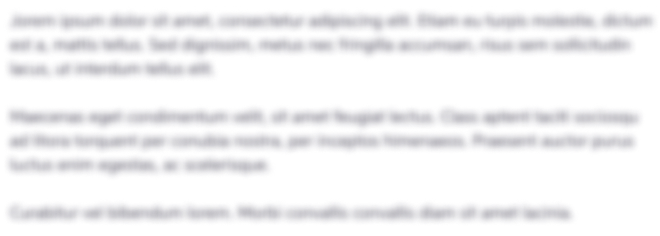
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started