Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
c + + #ifndef _ HEAP _ H _ #define _ HEAP _ H _ #include #include using std::vector; using std::cout; using std::cin; using std::endl;
c #ifndef HEAPH #define HEAPH #include #include using std::vector; using std::cout; using std::cin; using std::endl; class BinaryHeap private: vector array; The heap array int currentSize; void buildHeap; void percolateDown int pos ; void percolateUp int pos ; public: explicit BinaryHeap int capacity ; explicit BinaryHeap const vector & items ; int size const; bool isEmpty const; void print const; int getMin const; void insert const int & x ; void deleteMin; void deleteMin int & minItem ; void decreaseKey int pos, int amountToDecrease ; void increaseKey int pos, int amountToIncrease ; ; Constructor @param capacity the initial capacity of the heap BinaryHeap::BinaryHeapint capacity array vectorcapacity; currentSize ; delete the minimum from the heap @return bool whether the heap is empty bool BinaryHeap::isEmpty const return currentSize ; delete the minimum from the heap @return bool whether the heap is empty int BinaryHeap::size const return currentSize; Print the heap in a linear array void BinaryHeap::print const TODO get the minimum value in heap @return x the minimum value int BinaryHeap::getMin const TODO just a place holder return ; insert an item to the heap @param x the value to be inserted to the heap void BinaryHeap::insert const int & x TODO delete the minimum from the heap void BinaryHeap::deleteMin TODO delete the minimum from the heap @param minItem the value of the minimium item will be assigned to minItem void BinaryHeap::deleteMin int & minItem TODO Percolate down an item @param pos the array position of the item to be percolated down. void BinaryHeap::percolateDown int pos TODO Percolate up an item @param pos the array position of the item to be percolated up void BinaryHeap::percolateUpint pos TODO Constructor to create a Heap from a vector of integers @param items a vector of integers to construct the heap BinaryHeap::BinaryHeap const vector & items TODO use buildheap not allowed to use insert Establish heap order property from an arbitrary arrangement of items. Runs in linear time. void BinaryHeap::buildHeap TODO Increase the value of a node Thus decrease the priority @param pos the array position of the item to increase @param amountToIncrease the positive value to be ADDED to the item void BinaryHeap::increaseKeyint pos, int amountToIncrease TODO Decrease the value of a node Thus increase the priority @param pos the array position of the item to decrease @param amountToDecrease the positive value to be SUBTRACTED from the item void BinaryHeap::decreaseKeyint pos, int amountToDecrease TODO #endif HEAPH
c #ifndef HEAPH
#define HEAPH
#include
#include
using std::vector;
using std::cout;
using std::cin;
using std::endl;
class BinaryHeap
private:
vector array; The heap array
int currentSize;
void buildHeap;
void percolateDown int pos ;
void percolateUp int pos ;
public:
explicit BinaryHeap int capacity ;
explicit BinaryHeap const vector & items ;
int size const;
bool isEmpty const;
void print const;
int getMin const;
void insert const int & x ;
void deleteMin;
void deleteMin int & minItem ;
void decreaseKey int pos, int amountToDecrease ;
void increaseKey int pos, int amountToIncrease ;
;
Constructor
@param capacity the initial capacity of the heap
BinaryHeap::BinaryHeapint capacity
array vectorcapacity;
currentSize ;
delete the minimum from the heap
@return bool whether the heap is empty
bool BinaryHeap::isEmpty const
return currentSize ;
delete the minimum from the heap
@return bool whether the heap is empty
int BinaryHeap::size const
return currentSize;
Print the heap in a linear array
void BinaryHeap::print const
TODO
get the minimum value in heap
@return x the minimum value
int BinaryHeap::getMin const
TODO
just a place holder
return ;
insert an item to the heap
@param x the value to be inserted to the heap
void BinaryHeap::insert const int & x
TODO
delete the minimum from the heap
void BinaryHeap::deleteMin
TODO
delete the minimum from the heap
@param minItem the value of the minimium item will be assigned to minItem
void BinaryHeap::deleteMin int & minItem
TODO
Percolate down an item
@param pos the array position of the item to be percolated down.
void BinaryHeap::percolateDown int pos
TODO
Percolate up an item
@param pos the array position of the item to be percolated up
void BinaryHeap::percolateUpint pos
TODO
Constructor to create a Heap from a vector of integers
@param items a vector of integers to construct the heap
BinaryHeap::BinaryHeap const vector & items
TODO
use buildheap not allowed to use insert
Establish heap order property from an arbitrary
arrangement of items. Runs in linear time.
void BinaryHeap::buildHeap
TODO
Increase the value of a node
Thus decrease the priority
@param pos the array position of the item to increase
@param amountToIncrease the positive value to be ADDED to the item
void BinaryHeap::increaseKeyint pos, int amountToIncrease
TODO
Decrease the value of a node
Thus increase the priority
@param pos the array position of the item to decrease
@param amountToDecrease the positive value to be SUBTRACTED from the item
void BinaryHeap::decreaseKeyint pos, int amountToDecrease
TODO
#endif HEAPH
Step by Step Solution
There are 3 Steps involved in it
Step: 1
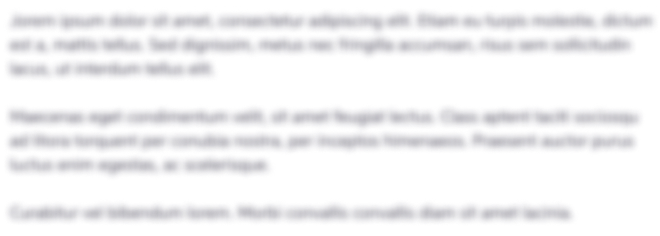
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started