Question
C++ inheritance Define and implement a class named Hunter . A Hunter object represents an Animal that hunts for its food in the wild. The
C++ inheritance
Define and implement a class namedHunter. AHunterobject represents anAnimalthat hunts for its food in the wild. TheHunterclass must be defined by inheriting from theAnimalclass. TheHunterclass has the following public constructors and behaviours:
Hunter(string aSpecies);// create a hunter of the given species
void record_kill(string kill);// add a new kill to the end of the hunter's list of kills
int numberOfKills();// how many kills have been recorded
// implement ONE of the following
string * get_kills();// return an array of all kills by this hunter
vector
Your main program should create aHunterobject to represent a "Cheetah" and record kills of 1 "Mouse", 2 "Gazelle", 1 "Hyena" and 2 "Rabbit". TheHunterobject initially has no kills. Your main program must print all the kills recorded by theHunterobject.
a class namedAnimalthat has the following public constructors and behaviours:
Animal(string aSpecies) ;// animals are allocated a unique ID on creation,
// the first animal has ID 1, the second animal is 2 and so on
void set_name(string aName);// change the animal's name
string get_species();
string get_name();
int get_ID();// the animal's unique ID
This should be straight forward except for the ID.How do we keep track of what ID number we are up to?At the moment we have no way to keep track of the number of animals that have been created.The solution to this problem is to declare aclass variable.A class variable as its name implies is a variable that belongs to theclass itself, not object instances.Itis declared in the class declaration (just like other state variable) but is identified as a class variable by the keywordstatic.There is onlyonecopy of this variable no matter how many object instances are created.
Class variables are declared in the
static int currentID ;// the next id number to give out
and theAnimal.cppfile must contain (outside of any of the method implementations):
int Animal::currentID = 0 ;
You can access the currentID variable when you are creating objects of class animal.Think carefully about this use.currentID should be 1 when you create your first animal, 2 when you create your second animal, and so on.What will you need to do to currentID whenever you create an animal?Each animal must have their own unique ID.Do they need their own state variable to hold this unique ID or can they use currentID?
Your main program should create twoAnimalobjects, an "Elephant" and a "Cheetah", then display their details. Your main program should demonstrate that the name can be changed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
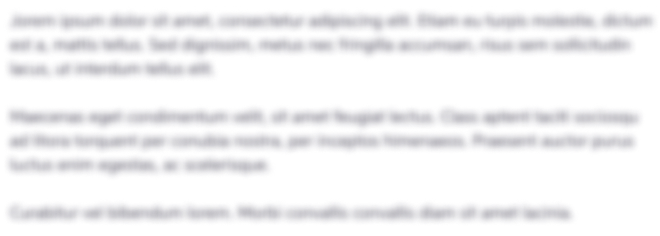
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started