Question
C++ Lab -- Generic Containers * Please note that referenced code has been included at the bottom for your convenience. ********************************************************************************************************************************************************************************************************************* // simpleStack.cc #include simpleStack.h
C++ Lab -- Generic Containers
* Please note that referenced code has been included at the bottom for your convenience.
*********************************************************************************************************************************************************************************************************************
// simpleStack.cc
#include "simpleStack.h"
void Stack::push(const StackType &d) throw (ContainerFullException) {
if (count == STACK_SIZE) throw ContainerFullException("SimpleStack");
data[count] = d;
count++;
// data[count++] = d; }
StackType Stack::pop(void) throw (ContainerEmptyException) {
if (!count) throw ContainerEmptyException("SimpleStack");
count--;
return data[count];
// return data[--count]; }
StackType Stack::peek(void) throw (ContainerEmptyException) {
if (!count) throw ContainerEmptyException("SimpleStack");
return data[count-1]; }
*********************************************************************************************************************************************************************************************************************
// simpleStack.h
#ifndef _SIMPLESTACK_H
#define _SIMPLESTACK_H
#include
const int STACK_SIZE = 10; typedef int StackType;
class Stack { public: Stack(void) { count = 0; } ~Stack(void) { }
void clear(void) { count = 0; } int size(void) { return count; } bool isEmpty(void) { return count == 0; }
void push(const StackType &) throw (ContainerFullException); StackType pop(void) throw (ContainerEmptyException); StackType peek(void) throw (ContainerEmptyException);
private: StackType data[STACK_SIZE]; int count; };
#endif
*********************************************************************************************************************************************************************************************************************
// zenStack.cc
#include "zenStack.h"
void ZenStack::push(void *p) throw (ContainerFullException) {
if (count == STACK_SIZE) throw ContainerFullException("ZenStack");
memcpy(data+count*dSize,p,dSize);
count++; }
void ZenStack::pop(void *p) throw (ContainerEmptyException) {
if (count == 0) throw ContainerEmptyException("ZenStack");
count--;
memcpy(p,data+count*dSize,dSize); }
void ZenStack::peek(void *p) throw (ContainerEmptyException) {
if (count == 0) throw ContainerEmptyException("ZenStack");
memcpy(p,data+(count-1)*dSize,dSize); }
*********************************************************************************************************************************************************************************************************************
// zenStack.h
#ifndef _ZENSTACK_H #define _ZENSTACK_H
#include
using namespace std;
const int STACK_SIZE = 1024;
class ZenStack { public: ZenStack(int s) { count = 0; dSize = s; } ~ZenStack(void) { }
void clear(void) { count = 0; } int size(void) { return count; } bool isEmpty(void) { return count == 0; }
void push(void *) throw (ContainerFullException); void pop(void *) throw (ContainerEmptyException); void peek(void *) throw (ContainerEmptyException);
private: uint8_t data[STACK_SIZE]; uint32_t count, dSize; };
#endif
*********************************************************************************************************************************************************************************************************************
// stackTest1.cc
#include
#include "simpleStack.h"
using namespace std;
int main(void) { int x,d;
Stack myStack;
cout > x;
while (x != 0) { d = x % 10; x /= 10;
myStack.push(d); }
while (!myStack.isEmpty()) { d = myStack.pop(); cout
return 0; }
*********************************************************************************************************************************************************************************************************************
// stackTest2.cc
#include
#include "zenStack.h"
using namespace std;
int main(void) { int x,d;
Stack myStack;
cout > x;
while (x != 0) { d = x % 10; x /= 10;
myStack.push(d); }
while (!myStack.isEmpty()) { d = myStack.pop(); cout
return 0; }
*********************************************************************************************************************************************************************************************************************
// stackTest3.cc
#include
using namespace std;
int main(void) { int x,d;
Stack myStack;
cout > x;
while (x != 0) { d = x % 10; x /= 10;
myStack.push(d); }
while (!myStack.isEmpty()) { d = myStack.pop(); cout
return 0; }
*********************************************************************************************************************************************************************************************************************
// stack.h
#ifndef _STACK_H #define _STACK_H
#include
const int STACK_SIZE = 10; typedef int StackType;
class Stack { public: Stack(void) { count = 0; } ~Stack(void) { }
void clear(void) { count = 0; } int size(void) { return count; } bool isEmpty(void) { return count == 0; }
void push(const StackType &d) throw (ContainerFullException) {
if (count == STACK_SIZE) throw ContainerFullException("Stack");
data[count] = d;
count++; }
StackType pop(void) throw (ContainerEmptyException) {
if (!count) throw ContainerEmptyException("Stack");
count--;
return data[count];
}
StackType peek(void) throw (ContainerEmptyException) {
if (!count) throw ContainerEmptyException("Stack");
return data[count-1]; }
private: StackType data[STACK_SIZE]; int count; };
#endif
Experiment with various methods for creating c the same program. rs that can hold multiple types of data within So far, you have seen a class-a collection of data and the code that manlpulates the data. You may have also seen a struct, which ls Just the collection of data without the code. A union is simllar to a struct. The dlfference ls, whlle a struct can hold many values Inside of Itself simultaneously, a union can only hold one value at a time. Conslder the following struct 1 struct 2 int foo; 3 double bar This struct can hold an Integer foo value and a double-preclsion value bar simultaneously. Now, conslder the following union: 1 union f 2 int foo; 3 double bar This can hold elther foo or bar, but not both slmultaneously. The struct has enough space to hold both-12 bytes on most systems-while the union only has enough space for the largest of its tems-the double. which Is 8 bytes. To try this method out, you wll need to modify the following files: simpleStack.h simpleStack.cc . stackTestl.cc First, modify simpleStack.h, replacing the typedef line with the following: 1 union UItem f 2 int iVal; 3 double dVal; 6 typedef union UItem StackType; Experiment with various methods for creating c the same program. rs that can hold multiple types of data within So far, you have seen a class-a collection of data and the code that manlpulates the data. You may have also seen a struct, which ls Just the collection of data without the code. A union is simllar to a struct. The dlfference ls, whlle a struct can hold many values Inside of Itself simultaneously, a union can only hold one value at a time. Conslder the following struct 1 struct 2 int foo; 3 double bar This struct can hold an Integer foo value and a double-preclsion value bar simultaneously. Now, conslder the following union: 1 union f 2 int foo; 3 double bar This can hold elther foo or bar, but not both slmultaneously. The struct has enough space to hold both-12 bytes on most systems-while the union only has enough space for the largest of its tems-the double. which Is 8 bytes. To try this method out, you wll need to modify the following files: simpleStack.h simpleStack.cc . stackTestl.cc First, modify simpleStack.h, replacing the typedef line with the following: 1 union UItem f 2 int iVal; 3 double dVal; 6 typedef union UItem StackTypeStep by Step Solution
There are 3 Steps involved in it
Step: 1
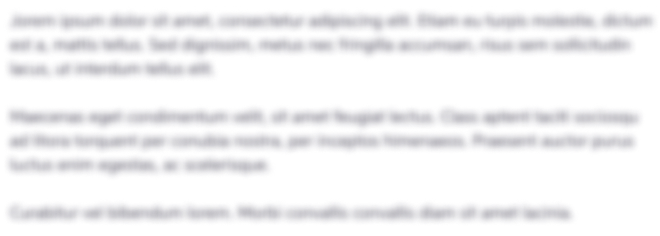
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started