Question
C++ Needs to be documented. Example code: #include #include #include #include #include using namespace std; class Person { private: string firstName; string lastName; string address;
C++ Needs to be documented.
Example code:
#include
#include
#include
#include
#include
using namespace std;
class Person
{
private:
string firstName;
string lastName;
string address;
public:
Person()
{
setFirstName("");
setLastName("");
setAddress("");
}
Person(string firstName, string lastName)
{
setFirstName(firstName);
setLastName(lastName);
// set the address to blank
setAddress("");
}
Person(string firstName, string lastName, string address)
{
setFirstName(firstName);
setLastName(lastName);
// set the address to blank
setAddress(address);
}
string getFirstName() { return firstName; }
string getLastName() { return lastName; }
string getAddress() { return address; }
void setFirstName(string firstName) { this->firstName = firstName; }
void setLastName(string lastName) { this->lastName = lastName; }
void setAddress(string address) { this->address = address; }
virtual void print()
{
cout << firstName << " " << lastName << ", " << address << endl;
}
};
class Player : public Person
{
private:
double average;
double onBasePercentage;
double sluggingPercentage;
public:
Player()
{
// set all the players to 0
setAverage(0);
setOBP(0);
setSlugging(0);
}
Player(string first, string last, string address, double average, double slugging, double onBase)
{
setFirstName(first);
setLastName(last);
setAddress(address);
setAverage(average);
setOBP(onBase);
setSlugging(slugging);
}
// setter for the values
void setAverage(double average) { this->average = average; }
void setSlugging(double slug) { this->sluggingPercentage = slug; }
void setOBP(double OnBase) { this->onBasePercentage = OnBase; } // the print void print() { cout << getFirstName() << " " << getLastName() << ", " << getAddress() << ", " << average << ", " << sluggingPercentage << "%, " << onBasePercentage << "%" << endl; }
};
class AddressBook : public vector
{
private:
int index = 0;
public:
AddressBook() {}
AddressBook(string filename) {
ifstream in(filename);
if (!in) {
cout << "File not found!!! ";
exit(0);
}
string line;
while (getline(in, line)) {
stringstream ss(line);
string token, fName, lName, address;
int i = 0;
while (getline(ss, token, ',')) {
if (i == 0) {
fName = token;
i++;
}
else if (i == 1) {
lName = token;
i++;
}
else {
address = token;
i = 0;
}
}
setPerson(fName, lName, address);
}
in.close();
}
AddressBook(string first, string last) { push_back(new Person(first, last, "")); }
AddressBook(string first, string last, string address) { push_back(new Person(first, last, address)); }
void setPerson(string first, string last, string address) { push_back(new Person(first, last, address)); }
Person* getPerson()
{
if (size() == 0)
return NULL;
Person* nextPerson = at(index);
index = (index + 1) % size();
return nextPerson;
}
Person* findPerson(string last)
{
for (int i = 0; i < size(); i++)
{
if (at(i)->getLastName() == last)
return at(i);
}
return NULL;
}
Person* findPerson(string first, string last)
{
// find the person with this last name
for (int i = 0; i < size(); i++)
{
if (at(i)->getLastName() == last && at(i)->getFirstName() == first)
return at(i);
}
return NULL;
}
void print()
{
for (int i = 0; i < size(); i++)
{
at(i)->print();
}
}
void writeToFile(string filename) {
ofstream out(filename);
for (int i = 0; i < size(); i++)
{
out << at(i)->getFirstName() << "," << at(i)->getLastName() << "," << at(i)->getAddress() << endl;
}
out.close();
}
};
int main()
{
AddressBook addressBook1("address.txt");
if (addressBook1.findPerson("Wayne") != NULL)
cout << "Error: Address Book must be empty!" << endl;
else
cout << "Pass: Address Book is empty when created!" << endl;
addressBook1.setPerson("Bruce", "Wayne", "Wayne Tower");
addressBook1.setPerson("Clark", "Kent", "Daily Mail");
addressBook1.setPerson("Thor", "Son of Odin", "Asgard");
if (addressBook1.findPerson("Wayne") != NULL)
cout << "Pass: Person with last name found!" << endl;
else
cout << "Error: Person with last name not found!" << endl;
if (addressBook1.findPerson("Clark", "Kent") != NULL)
cout << "Pass: Person with last name and first name found!" << endl;
else
cout << "Error: Person with last name and first name not found!" << endl;
if (addressBook1.findPerson("Bruce", "Banner") == NULL)
cout << "Pass: Person with last name and first name not found!" << endl;
else
cout << "Error: Person with last name and first name found!" << endl;
cout << endl;
// print the address book
addressBook1.print();
cout << endl;
//Call function to write into file
addressBook1.writeToFile("address.txt");
}
Problem:
This assignment is intended as a means for you to practice file IO. You will be required to have an understanding of opening a file, closing a file, reading a file a line at a time and writing to a text file a line at a time. You will also be required to do some simple parsing of information read from a text file and formatting output to be written to a text file.
Create an AddressBookException class that you can use to throw exceptions in your address book. Your class should have a constructor that will allow you to set the particular message that corresponds to the error.
You should use your custom exception class to throw an error in the findPerson method if the person being searched for cannot be found, in the getPerson method if the AddressBook is empty. You should also throw an error any time that the addressbook file that you are attempting to read or write from cannot be opened.
In main test your AddressBook exception class by placing a try - catch around any code that could possibly throw an error. Supply a catch block to catch the AddressBook exception and provide a catch all block just in case an exception is thrown the you may have missed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
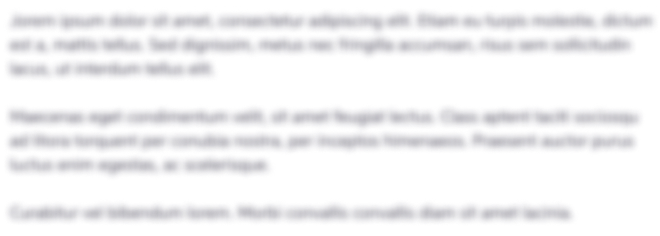
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started