Question
C++, please solve it step by step. thank you Biguint: Sometimes you need to store reaaaaaally big integers. Unsigned long ints only store numbers up
C++, please solve it step by step. thank you
Biguint: Sometimes you need to store reaaaaaally big integers. Unsigned long ints only store numbers up to 10 decimal digits. So were going to create a class biguint (for Big Unsigned Integer) to (eventually) allow us to store arbitrarily large unsigned integers. For now, they will store numbers with up to 20 decimal digits. The class will have a private member integer array, where each spot in the array stores one digit of the number. You will store the 1s digit in spot 0, the 10s digit in spot 1, the 100s digit in spot 2, etc, with zeros filling in any unused leading spots (do you notice a relationship between the spot index and the digit stored there? That is, betwee 1 and 0, 10 and 1, 100 and 2, ). So the number 1,472 would be stored in the array like this: 2 7 4 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 Weve used an array of unsigned shorts, because they are the smallest int type, and we will never need to store a value larger than 9.
1. Write the default constructor. It should simply fill in the array with 0s. Call it from main (though you wont be able to see whether it was created correctly).
2. Explain how you plan to write the constructor that takes in a string. How can you convert the character 5 to the number 5? Recall your character arithmetic.
3. Write the constructor that takes in a string. Be sure to fill all the extra spots with 0. Call it from main (though you wont be able to see whether it was created correctly).
4. Overload the [] operator to return the ith digit in the biguint (use the array indexing; so if they do [0] you will give the digit in the ones place). What should your function return if the value in a is 1472 and a[900] is called? Call [] from main; use it to see whether the constructors worked.
5. Overload << as a non-member function. Its fine to print out the leading 0s. Call it from main.
6. Explain how you plan to overload +=. Think about how you learned to add in elementary school.
7. Overload += as a member function. Call it from main.
Start from the header file you can find here:
#ifndef BIGUINT_H #define BIGUINT_H #include#include #include // WANT: integers with CAPACITY digits, only non-negative // // support: // 2 constructors: int, string // member functions: [] returns individual digits given position // += // -= // compare: return +1, 0, -1, depending on // whether this biguint >, ==, < than given biguint // +, - (binary), - (unary), <, <=, ==, !=, >=, > // <<, >> class biguint { public: // CONSTANTS & TYPES static const std::size_t CAPACITY = 20; // CONSTRUCTORS // pre: none // post: creates a biguint with value 0 biguint(); // pre: s contains only decimal digits // post: creates a biguint whose value corresponds to given string of digits biguint(const std::string &); // CONSTANT MEMBER FUNCTIONS // pre: pos < CAPACITY // post: returns the digit at position pos // 0 is the least significant (units) position unsigned short operator [](std::size_t pos) const; // pre: none // post: returns 1 if this biguint > b // 0 if this biguint == b // -1 if this biguint < b int compare(const biguint & b) const; // MODIFICATION MEMBER FUNCTIONS // pre: none // post: b is added to this biguint; ignore last carry bit if any void operator += (const biguint & b); void operator -= (const biguint & b); private: unsigned short data_[CAPACITY]; // INVARIANTS: // data_[i] holds the i^th digit of this biguint or 0 if not used // data_[0] holds the least significant (units) digit }; // nonmember functions biguint operator + (const biguint &, const biguint &); biguint operator - (const biguint &, const biguint &); bool operator < (const biguint &, const biguint &); bool operator <= (const biguint &, const biguint &); bool operator != (const biguint &, const biguint &); bool operator == (const biguint &, const biguint &); bool operator >= (const biguint &, const biguint &); bool operator > (const biguint &, const biguint &); #endif // BIGUINT_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
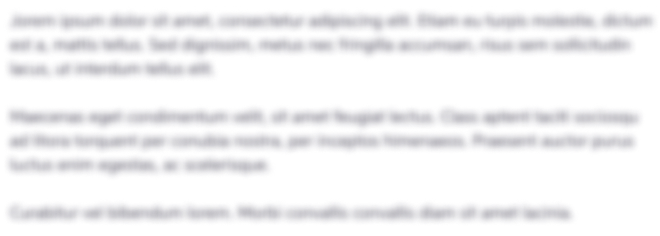
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started