Question
C++ Problem: 1. Write a program in C++ that asks the user to enter an integer at the console and then analyze the number for
C++ Problem:
1. Write a program in C++ that asks the user to enter an integer at the console and then analyze the number for the following properties:
Is the number positive.
Is the number negative.
Is the number even.
Is the number a perfect square. A perfect square is a positive integer that is the square of two equal integers.
Is the number prime. Remember negative numbers, zero and one are all considered to not be prime and two is considered to be prime. Any number greater than two is prime if it is divisible by any positive integer other than one and itself.
For each of these properties, write a separate function that takes a number as the argument and returns a boolean result. Inside of your main function once you receive the number from the user, call each of these functions and print the result to the console.
Expected Output:
> Enter a number: 17 > Is the number positive: true > Is the number negative: false > Is the number even: false > Is the number square: false > Is the number prime: true
2. The provided code sets up an array with 20 random numbers in the range [0, 100]. Ask the user for a number at the console and then print out whether or not that value is found in the array.
Starter Code:
#include#include #include constexpr size_t LENGTH = 20; static void populate(int numbers[]); int main() { int numbers[LENGTH]; populate(numbers); for (auto i = 0; i < LENGTH; i++) { std::cout << numbers[i] << ", "; } std::cout << " "; // TODO: ask the user for a number and print out true if // it is in the array, and false otherwise } static void populate(int numbers[]) { static std::mt19937 generator{ std::random_device{}() }; static std::uniform_int_distribution range{ 0, 100 }; for (auto i = 0; i < LENGTH; i++) { numbers[i] = range(generator); } }
Expected Output:
> 75, 68, 51, 39, 49, 1, 28, 53, 76, 56, 46, 8, 87, 54, 36, 26, 6, 42, 20, 66, > Enter a number: 49 > Found: true
Step by Step Solution
There are 3 Steps involved in it
Step: 1
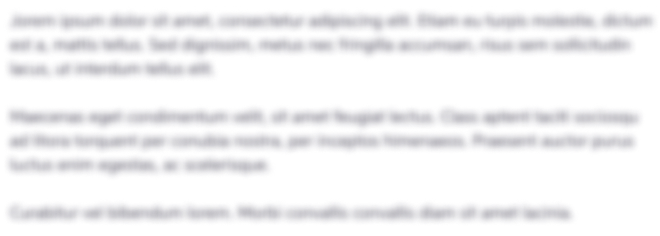
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started