Question
C Progaming Purpose: Use bit operations to extract information Demonstrate understanding of number formats and binary representations Assignment: Computers sometimes use a format called binary
C Progaming
Purpose:
- Use bit operations to extract information
- Demonstrate understanding of number formats and binary representations
Assignment:
Computers sometimes use a format called binary coded decimal or BCD. Good background information is available from https://en.wikipedia.org/wiki/Binary-coded_decimal , but it is far deeper than you need for this assignment. (read at your own risk, the section marked Basics covers all you need to know at this point in time).
BCD encodes a decimal number into 4 binary digits. A byte holds 2 BCD digits. A 32 bit word can contain 8 packed BCD digits.
Decimal digit | BCD | |||
8 | 4 | 2 | 1 | |
0 | 0 | 0 | 0 | 0 |
1 | 0 | 0 | 0 | 1 |
2 | 0 | 0 | 1 | 0 |
3 | 0 | 0 | 1 | 1 |
4 | 0 | 1 | 0 | 0 |
5 | 0 | 1 | 0 | 1 |
6 | 0 | 1 | 1 | 0 |
7 | 0 | 1 | 1 | 1 |
8 | 1 | 0 | 0 | 0 |
9 | 1 | 0 | 0 | 1 |
Write a program that accepts an integer input (base 10) to stdin. This input value is in packed BCD format. Note that not every possible decimal input value will map to a corresponding BCD number, some bit patterns are not valid BCD. Using the packed BCD format, display the number as a sequence of characters to stdout. The sequence of characters is a simulation of a calculators display (5 characters wide, 5 characters tall, with a space between each).
- Display any BCD digit as a 0 if it is an invalid encoding.
- All numbers are positive.
- Note that the number provided as input data is in packed BCD representation.
- Process input until a -1 is entered. Do not process the -1, just finish without printing anything out.
- Output must match the example exactly. Dont try and make the calculator display more readable.
- Use bit operations to extract the digits.
- The program must work for any valid input values; provided they do not overflow an integer. Suggest you test with 10 or 20 different values prior to turning it in.
- A two dimensional array is useful, but not essential for completing this program.
- Including comments, this assignment can be completed in less than 100 lines of code. If you are writing much more than this, you are on the wrong track. Ask for help.
- Every function must have comments that describe its purpose,implementation, and interface.
- Any pre and post conditions for a function must be described in the comments, and checked at runtime using assert ().
- As in previous assignments, suggest you use bash input redirection to make a test file to verify you program works with many inputs.
- Compile with -std=c99
- Turn into blackboard a single C file.
Examples:
Consider an input value of 4660 base 10.
This number is 0x1234 expressed in hex.
Or
0000 0000 0000 0001 0010 0011 0100
In packed BCD, this number is
0001234
Another examples:
Input value of 39321 base 10.
This number is 0x9999 expressed in hex.
Or
1001 1001 1001 1001
In packed BCD, this number is
9999
Here is an example of a decimal number that is not a valid representation of a BCD number
Input value of 298 base 10.
This number is 012A hex.
Or
0000 0001 0010 1010
This is not a valid packed BCD number, as the last digit is 1010, which is not a valid BCD digit.
For the purpose of making test cases for this assignment:
- Determine the BCD value you want to display on the calculator display
- Write down the bit pattern for this number
- Convert the bit pattern to hex. (note no hex digit is > 9 for valid bcd)
- Convert that hex number into decimal. This is the input to the program.
Grading Criteria:
1. Correct output, observed by running the code against several input files; each input file containing several input values.
2. Indentation, commenting of code
3. Compliance with the assignments requirements
4. Compiles without warnings using -Wall
Example Output:
$ gcc -Wall -std=c99 -g hw4.c
$ ./a.out
4660 (reads 00001234)
--- --- --- --- ---- ----
| | | | | | | | | / | |
| | | | | | | | | / ---- ----
| | | | | | | | | / | ^
--- --- --- --- ---- ---- ^
305419896 (reads 12345678)
---- ---- ---- ---- ---
| / | | | | / | |
| / ---- ---- ---- --- / ---
| / | ^ | | | / | |
---- ---- ^ ---- --- ---
39321 (reads 00009999)
--- --- --- --- --- --- --- ---
| | | | | | | | | | | | | | | |
| | | | | | | | --- --- --- ---
| | | | | | | | | | | |
--- --- --- ---
-1 (ends program)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
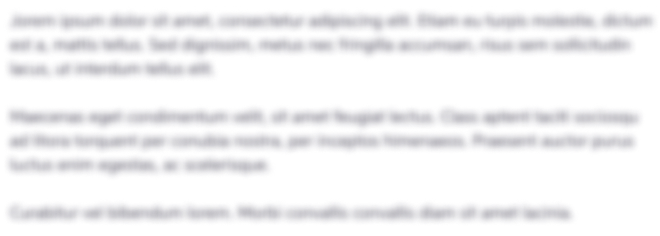
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started