Question
C program: General Game Description: The G a me of the Goose (https://en.wikipedia.org/wiki/Game_of_the_Goose) is a centuries old board game that consists of spiral path of
C program:
General Game Description:
The Game of the Goose (https://en.wikipedia.org/wiki/Game_of_the_Goose) is a centuries old board game that consists of spiral path of 63 spaces on which two or more players take turns moving their pieces. On each player's turn, they roll two dice, and then move their piece by the sum of the values shown. The objective is to reach the last space (space 63) with an exact roll before the other players. For example, suppose that a player is n spaces away from space 63. If the player rolls m with the dice, and m>n, he must move towards 63 (n spaces) and then back m - n spaces until the piece has been moved m steps. However if the dice sum is exactly n, then the player wins.
Special spaces
There are some special spaces that modify the player's move in case they land on them. In some spaces the goose is depicted. When a player's piece lands on a goose, it is moved farther along by the same distance (or from goose to goose in other variants of the game). A space with a bridge, moves the piece forward to another specified position. On most versions of the game, the bridge is from space 6 to space 12. There are also some "Hazard spaces" which usually make the player lose his turn until certain conditions are met. If the player lands on the maze, they must return to the space where they started that turn. If the player lands on the skull (or death), they must return their piece to the beginning and start again.
Project Description:
In this Project, you will write a C program to create a simple version of the Game of the Goose using 24 spaces and a two-player game mode. Player 1 will be the human player, player 2 will be the computer.
When running the program, the user will be asked to enter a positive integer number, which will be used as a seed for the generation of random numbers when rolling the dice. Then, your program prints a welcome menu displaying two options:
1) Press 'P' or 'p' to play or
2) Press 'Q' or 'q' to quit.
If the selected option is to play the game, the players should roll the dice (press enter) to determine which player should go first. The player who gets the highest value will go first in the game. Here is an example:
HUMAN PLAYER, Press
6 and 2 for a 8
COMPUTER PLAYER, Press
3 and 2 for a 5
HUMAN PLAYER goes first
If there is a tie determining who goes first (i.e. both the Human and Computer roll the same value), a message is printed stating so, and the process is repeated until two different values are rolled. The highest roll then goes first.
After determining which player goes first, 24 spaces are displayed in two lines, each represented by a number enclosed in square brackets (12 numbers in each line) and separated by tabs. The only exception is the last space which doesn't use bracket but instead is displayed as <24>. A character before a number indicate that it is a special space. A goose is represented by '+', the bridge by '*', a maze by '-' and the skull by '!'. The following table indicates the special spaces:
Symbol | Spaces | Action |
goose | 7, 11, 15 | move your piece again by the same distance |
bridge | 6 | go to space 12 |
maze | 13, 20 | go back to your previous space |
skull | 23 | return to the beginning |
The characters '$' and '%' are used to represent the pieces of human and computer player, respectively. When a player lands on a space, the space number is NOT displayed but only the player instead. If both players are in the same space, human's character is always shown first.
Playing the game:
-
After selecting play in the main menu the goose board is displayed with both players' pieces in the start position.
-
Below the board, a message is displayed indicating which player will next roll the dice (assuming a player didn't win the previous roll). This information should be shown in a manner similar to:
[HUMAN/COMPUTER] PLAYER'S TURN, [current space]... Press
-
After the player presses
(the human player should also "roll" for the computer) the value of each die along with the sum of both dice is shown. The next line shows a summary of the main movements performed in that roll. If more than one movement is carried out, a comma separates each movement. The main possible movements are: -
go to space #
- come back to space #
- return to start
-
-
After the main movements are performed, the new board status is shown
-
If the new board status shows that the player won during the roll, a message is displayed indicating that the game is over, and stating which player (Human or Computer) wins:
"-GAME OVER-" Player # won!
-
Then the following message is displayed, allowing the user to return to the main menu:
Press
Implementation:
You must create at least two functions (in addition to main()) for this project. If desired you can create additional functions as you see fit. Make sure that your code and the number of lines per function follows the CS 262 Style Guide.
Rolling the dice
A "roll" function will be used to roll the dice. It will return an int value and contain no parameters. This function will declare two integer variables (each represents a single die). Use a random number generator for each variable, so that the possible values range from one to six. The function will print the result of each dice and the sum of both. and return the sum. Example: "5 and 2 for a 7". Use the following function header:
int roll_Dice(void)
To read an explanation how to use random( ) to generate random numbers click here.
Print board
You will implement a function to print and show the status of the board. It will have two parameters (the positions of both players) and will display the board with the symbols of the special spaces and the spaces where are the players. The function will not have a return value (i.e. it will be a void function).Use the following function header:
void print_Board(int playerPos1, int playerPos2 )
Other considerations
The board size and positions of special spaces will be denoted using global constants and symbolic constants (#define). Because all of the various symbols could potentially be placed on one or more spaces on the game board, you should make symbolic constants to reflect the number of each type of symbol, and a global array of constants for each symbol type denoting the space that those symbols are found. Using the Goose spaces as an example:
#define NUM_GOOSE_SPACES 3
const int gooseSpaces[NUM_GOOSE_SPACES] = {7, 11, 15};
The idea is that your program can be modified to create different board sizes (such as the original 63 spaces), and number of and positions of special spaces merely by changing values at the top of the program (and perhaps some modification to the display board function) and recompiling. It is acceptable to declare an array containing a single element (which you would need to do for the bridge and the skull). You will not actually make such modifications for this project (i.e. generate programs with different board sizes), but you need to write your code so that it is easy to do if it was desired to do so in the future.
Don't forget to comment your code (including the standard information at the top of the file-name, section, etc.).
Example Output:
This is an example of some of the output that might be displayed on the screen based on the inputs and result of the dice sum:
Enter a seed for the random number generator: 10
Welcome to the game of goose, please select an option:
Press 'P' or 'p' to play
Press 'Q' or 'q' to quit
p
HUMAN PLAYER, Press
6 and 2 for a 8
COMPUTER PLAYER, Press
3 and 2 for a 5
HUMAN PLAYER goes first
[$%] [2] [3] [4] [5] *[6] +[7] [8] [9] [10] +[11] [12]
-[13] [14] +[15] [16] [17] [18] [19] -[20] [21] [22] ![23] <24>
HUMAN PLAYER'S TURN, [1]... Press
6 and 3 for a 9
go to space 10
[%] [2] [3] [4] [5] *[6] +[7] [8] [9] [$] +[11] [12]
-[13] [14] +[15] [16] [17] [18] [19] -[20] [21] [22] ![23] <24>
COMPUTER PLAYER'S TURN, [1]... Press
4 and 6 for a 10
go to space 11, go to space 21
[1] [2] [3] [4] [5] *[6] +[7] [8] [9] [$] +[11] [12]
-[13] [14] +[15] [16] [17] [18] [19] -[20] [%] [22] ![23] <24>
HUMAN PLAYER'S TURN, [10]... Press
3 and 2 for 5
go to space 15, go to space 20, come back to space 10
[1] [2] [3] [4] [5] *[6] +[7] [8] [9] [$] +[11] [12]
-[13] [14] +[15] [16] [17] [18] [19] -[20] [%] [22] ![23] <24>
COMPUTER PLAYER'S TURN, [21]... Press
1 and 2 for a 3
go to space 24
[1] [2] [3] [4] [5] *[6] +[7] [8] [9] [$] +[11] [12]
-[13] [14] +[15] [16] [17] [18] [19] -[20] [21] [22] ![23] <%>
Game over! Computer Won!
Press
Makefile for Compilation:
Copy your most recent Makefile and include as comments at the top:
#
Make sure gcc is set as the compiler for the the variable CC
Use CFLAGS = -Wall -g -O2 -std=c89 -D_XOPEN_SOURCE=600 -pedantic-errors
Include the targets clean: and all: as was indicated in previous lab Makefiles
Submission:
-
Create a directory named Project1,copy your source file and makefile to this directory
-
Move on your directory Project1
-
Create a typescript file named Project1_typescript_
-
Show that you are logged onto Zeus. Type: uname -a
-
Show that Project1 is your current work path. Type: pwd
-
Show a detailed listing of the current directory. Type: ls -l
-
Show the content of your code using cat.
-
Remove any executable versions of Project1_
that may appear. -
Compile the code with your makefile
-
Show that the executable file was created from make
-
End the typescript by typing Control-d.
Remove any executables from your directory. Create a tarfile of your directory containing your typescript, source file and makefile. Submit your tarfile to Blackboard as Project1.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
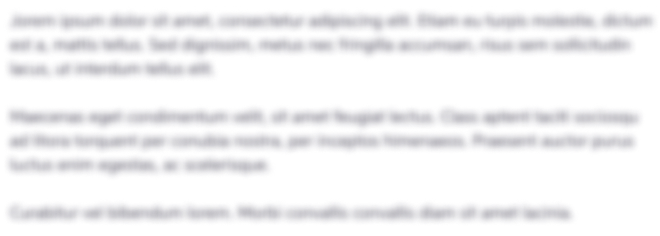
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started