Question
C++ program. I'm trying to run this code for the following project but I get 13 errors( the error list is at bottom). This program
C++ program. I'm trying to run this code for the following project but I get 13 errors( the error list is at bottom).
This program will have names and addresses saved in a linked list. In addition, a birthday and anniversary date will be saved with each record.
When the program is run, it will search for a birthday or an anniversary using the current date to compare with the saved date. It will then generate the appropriate card message.
Because this will be an interactive system, your program should begin by displaying a menu.
Items on the menu should include:
Enter a new name into the address book
Delete a name from the address book
Change a name or date in the address book
Display the whole address book
Generate birthday cards
Generate anniversary cards
Exit the card program
Each of these sections will call individual functions to perform their appropriate task.
This address book is to be sorted in alphabetical order. Be aware of this when you are entering, deleting, or changing the name, and plan your code accordingly. Use classes and objects where appropriate. Be sure to comment your code and use appropriate variable, function, and class names so that it is clear how the program flows.
The user gets the menu and creates information to be stored in your address book. (To save sanity in testing, you may fill in 5 or 6 records within the code or written to a file to start your address book.) The user can add a new record to your existing ones, delete a record, modify a record or print the whole address book on the screen. For each of the options, make sure you give suitable prompts on the screen so that the user knows what is expected by the program.
Expect that the user will enter both a birthday and anniversary date. (If youd like, you can handle the situation where the user chooses not to enter an anniversary date.) A year may be entered or omitted in birthday and anniversary dates. Also be sure to redisplay the menu any time a function has concluded, until the user presses the Exit option.
Create and display the card created on the screen (you do not need to print it). You may design
the layout of the card as you wish. For example, it could be:
Dear
Hope your birthday is really wonderful and this coming year is the best yet!
Love,
Joanne
Generate Birthday Cards and Generate Anniversary Cards will use the system date (today) as the date to match. Using this date, display the card below it on the screen. Be ready to print
multiple cards if more than one birthday or anniversary falls on the same day.
Display the Whole Address Book will be done on the screen. Output should look something like:
Wilson, Fred
123 Main Street
Anytown, NJ 00000
Birthday: May 7
Anniversary: June 25
Or include the structure variable names youve used:
lname: Wilson fname: Fred
addr: 123 Main Street
city: Anytown state: NJ zip: 00000
bday: May 7
aday: June 25
2. School calendar program.
3. Program that capitalizes words that begin with a.
4. Convert function to template.
5. Cylinder and circle derived class question.
//main.cpp
#include
#include
#include "LinkedList.h"
#include "AddressBook.h"
using namespace std;
void mainMenu(AddressBook& addresses);
void enterName(AddressBook& addresses);
void deleteName(AddressBook& addresses);
void changeName(AddressBook& addresses);
void generateBirthdayCards(AddressBook& addresses);
void generateAnniversaryCards(AddressBook& addresses);
void resetInput();
int main()
{
// Load the address book
AddressBook addresses;
addresses.load();
// Start the mainMenu loop
mainMenu(addresses);
return 0;
}
void mainMenu(AddressBook& addresses)
{
// Display main menu
cout << "------------------Main menu------------------" << endl;
cout << "[1]: Enter a new name into the address book" << endl;
cout << "[2]: Delete a name from the address book" << endl;
cout << "[3]: Change a name or date in the address book" << endl;
cout << "[4]: Generate birthday cards" << endl;
cout << "[5]: Generate anniversary cards" << endl;
cout << "[6]: Save and exit the card program" << endl;
cout << "[7]: Print address book." << endl;
// Prompt for user input.
cout << "Enter code here: ";
// Cleanup to make sure cin is working before gathering user input
resetInput();
int code;
cin >> code;
cout << endl;
// If their input failed, as they entered a word instead of a number
while (cin.fail())
{
// Cleanup to make sure cin is working before gathering user input
resetInput();
// Prompts that input failed, trys to recollect input
cout << "Input failed. Enter code here: ";
cin >> code;
cout << endl;
}
// Start the corresponding function the code that was entered.
switch (code)
{
case 1:
cout << "-----------------Enter Name------------------" << endl;
enterName(addresses);
break;
case 2:
cout << "-----------------Delete Name-----------------" << endl;
deleteName(addresses);
break;
case 3:
cout << "-----------------Change Name-----------------" << endl;
changeName(addresses);
break;
case 4:
cout << "---------------Birthday Cards----------------" << endl;
generateBirthdayCards(addresses);
break;
case 5:
cout << "-------------Anniversary Cards---------------" << endl;
generateAnniversaryCards(addresses);
break;
// Saves and exits the program
case 6:
cout << "Now closing..." << endl;
addresses.save();// Saves the addresses to the text file
exit(0);// Exits the program
break;
case 7:
cout << "----------------Address List-----------------" << endl;
addresses.print();
break;
default:
// The default operation will print wrong number.
cerr << "Sorry, wrong number." << endl;
break;
};
mainMenu(addresses);
}
void enterName(AddressBook& addresses)
{
// Cleanup to make sure cin is working before gathering user input
resetInput();
// Fill out form, for name, address, anniversary date, and birthday
Address cur;
cout << "Enter name:";
getline(cin, cur.name);
cout << "Enter Address:";
getline(cin, cur.streetAddress);
cout << "Enter Anniversary Date:";
getline(cin, cur.anniversaryDate);
cout << "Enter Birthday Date:";
getline(cin, cur.birthdayDate);
cout << endl;
// We then add the newly created address to the address book
addresses.add(cur);
}
void deleteName(AddressBook& addresses)
{
// Cleanup to make sure cin is working before gathering user input
resetInput();
// Get name to delete
string name;
cout << "Enter name to delete: ";
getline(cin, name);
cout << endl;
// Remove it from the address book
addresses.remove(name);
}
void changeName(AddressBook& addresses)
{
// Cleanup to make sure cin is working before gathering user input
resetInput();
// Get name to rename
string name;
cout << "Enter name to rename: ";
getline(cin, name);
// Get the address with the specific name
Address addressToBeDeleted = addresses.get(name);
// If the address is NULL, the entered name doesn't exist. Exit the function.
if (addressToBeDeleted.name == "NULL")
{
cerr << "Entered name does not exist!" << endl;
return;
}
// New Address that is being formed
Address* changeAddress = new Address;
changeAddress->streetAddress = addressToBeDeleted.streetAddress;
changeAddress->anniversaryDate = addressToBeDeleted.anniversaryDate;
changeAddress->birthdayDate = addressToBeDeleted.birthdayDate;
// Remove the old address
addresses.remove(name);
// Get the new name
cout << "Enter new name: ";
getline(cin, name);
cout << endl;
changeAddress->name = name;
// Add the new address to the address book
addresses.add(*changeAddress);
}
void generateBirthdayCards(AddressBook& addresses)
{
// Cleanup to make sure cin is working before gathering user input
resetInput();
// Get date
string date;
cout << "Enter date: ";
cin >> date;
// Generate birthday cards for the date
addresses.generateBirthdayCards(date);
}
void generateAnniversaryCards(AddressBook& addresses)
{
// Cleanup to make sure cin is working before gathering user input
resetInput();
// Get date
string date;
cout << "Enter date: ";
cin >> date;
// Generate anniversary cards for the date
addresses.generateAnniversaryCards(date);
}
// Cleanup to make sure cin is working before gathering user input
void resetInput()
{
// If it's failed, ignore the whole line
if (cin.fail())
{
// Removes any information user entered.
cin.clear();
// cin.ignore(std::numeric_limits::max(), ' ');
}
// If the next character is a newline, ignore it.
if (cin.peek() == ' ')
{
cin.ignore();
}
}
//Address.h
#ifndef ADDRESS_H
#define ADDRESS_H
#include
class Address
{
public:
// Create an Address using these 4 params
Address(std::string name = "Not Entered",
std::string streetAddress = "Not Entered",
std::string anniversaryDate = "XX-XX-XXXX",
std::string birthdayDate = "XX-XX-XXXX");
virtual ~Address();
// Let other files print out addresses
friend std::ostream& operator<<(std::ostream& os, const Address& curAddress);
std::string name;
std::string streetAddress;
std::string anniversaryDate;
std::string birthdayDate;
protected:
private:
};
#endif // ADDRESS_H
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
//Address.cpp
#include "Address.h"
// Can be created with name, address, aniv date, and bday
Address::Address(std::string name, std::string streetAddress, std::string anniversaryDate, std::string birthdayDate)
{
this->name = name;
this->streetAddress = streetAddress;
this->anniversaryDate = anniversaryDate;
this->birthdayDate = birthdayDate;
}
Address::~Address()
{
//dtor
}
// Can be printed out by an overloaded operator.
std::ostream& operator<<(std::ostream& outStream, const Address& curAddress)
{
outStream.clear();
outStream << "Name: " << curAddress.name << std::endl
<< "Address: " << curAddress.streetAddress << std::endl
<< "Anniversary Date: " << curAddress.anniversaryDate << std::endl
<< "Birthday Date: " << curAddress.birthdayDate << std::endl;
return outStream;
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
#pragma once
//LinkedList.h
#ifndef LINKEDLIST_H
#define LINKEDLIST_H
#include
#include
template
class LinkedList
{
public:
LinkedList();
virtual ~LinkedList();
int getLength();
virtual void print();
void add(Type value);
void remove(Type value);
protected:
int length;
struct Node
{
Type val;
Node* next;
};
Node* head, *tail;
};
/**
* Sets head and tail to null, sets length to be empty.
*/
template
LinkedList::LinkedList()
{
head = tail = NULL;
length = 0;
}
/**
* Destructor for the Linked List.
*/
template
LinkedList::~LinkedList()
{
Node* prev = NULL;
Node* it = head;
while (it != NULL)
{
prev = it;
it = it->next;
delete prev;
}
}
/**
* Prints the whole linked list.
*/
template
void LinkedList::print()
{
int index = 0;
Node* it = head;
while (it != NULL)
{
std::cout << "{" << index << ":" << it->val << "}";
if (it->next != NULL)
{
std::cout << ", ";
}
it = it->next;
}
}
/**
* @return the number of nodes in the linked list.
*/
template
int LinkedList::getLength()
{
return length;
}
template
void LinkedList::add(Type value)
{
Node* newNode = new Node;
newNode->val = value;
newNode->next = NULL;
if (head == NULL)
{
head = tail = newNode;
}
else
{
tail->next = newNode;
tail = newNode;
}
length++;
}
/**
* Removes all nodes that have the value passed in.
* @param value The value you want to remove.
*/
template
void LinkedList::remove(Type value)
{
Node* it = head;
Node* prev = NULL;
while (it->next != NULL)
{
if (it->val == value)
{
if (prev == NULL)
{
head = it->next;
delete it;
}
else
{
prev->next = it->next;
delete it;
}
}
prev = it;
it = it->next;
}
length--;
}
#endif // LINKEDLIST_H
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
#pragma once
//AddressBook.h
#ifndef ADDRESSBOOK_H
#define ADDRESSBOOK_H
#include "LinkedList.h"
#include "Address.h"
class AddressBook : public LinkedList
{
public:
AddressBook();
virtual ~AddressBook();
void print();// Print the Address Book
void add(Address curAddress);// Add an address
void remove(std::string name);// Remove an address associated with the name passed in
Address get(std::string name);// Get an address by passing in the name of that address
void generateBirthdayCards(std::string date);// Generate bday cards
void generateAnniversaryCards(std::string date);// Generate anniversary cards
void load();// Load the address book
void save();// Save the address book
protected:
private:
void printBirthdayCard(Address currAdress);
void printAnniversaryCard(Address currAdress);
};
#endif // ADDRESSBOOK_H
/////////////////////////////////////////////////////////////////////////////////////////////////////////////
//AddressBook.cpp
#include "AddressBook.h"
#include
#include
#include
AddressBook::AddressBook()
{
//ctor
}
AddressBook::~AddressBook()
{
//dtor
}
// Print all of the elements in the address book
void AddressBook::print()
{
Node* it = head;
while (it != NULL)
{
std::cout << it->val << std::endl;
it = it->next;
}
}
// Removes the passed in name
void AddressBook::remove(std::string name)
{
// start the iterator at the head
Node* it = head;
Node* prev = NULL;
// While our iterator isnt null
while (it != NULL)
{
// Get the current name out of the node
std::string curName = ((Address)it->val).name;
// If the current name and nameToBeDeleted are the same
if (curName == name)
{
// If we are at the head, set it's next node to the iterators next value
if (prev == NULL)
{
head = it->next;
delete it;
}
// Else set the previous values next value to the iterators next value
else
{
prev->next = it->next;
delete it;
}
// Decrease the length on removal
length--;
}
// Set the previous node to be the iterator
prev = it;
// Set the iterator to the next value
it = it->next;
}
}
// Returns the address at the name passed in, or an address whose name is NULL if the name isn't in the address book
Address AddressBook::get(std::string name)
{
// Set the iterator to the head of the linked list
Node* it = head;
// While our name isn't found, and we haven't gone through the whole list
while (it != NULL)
{
// Extract the iterators name
std::string curName = ((Address)it->val).name;
// If the iterators name is equal to the wanted name, then the value is found and returned
if (curName == name)
{
return it->val;
}
it = it->next;
}
// If the address wasn't found, return the address with the name of NULL
Address nullAdd;
nullAdd.name = "NULL";
return nullAdd;
}
// Go through every address, print out birthday cards with the same date as string passed in
void AddressBook::generateBirthdayCards(std::string date)
{
// Go through every address
Node* it = head;
while (it != NULL)
{
// If it's birthday is equal to the date passed in, print it's birthday card
Address curAddress = ((Address)it->val);
if (date == curAddress.birthdayDate)
{
printBirthdayCard(curAddress);
}
it = it->next;
}
}
void AddressBook::printBirthdayCard(Address curAddress)
{
std::cout << "-------------------------------------" << std::endl;
std::cout << "Dear " << curAddress.name << "," << std::endl << std::endl;
std::cout << "Hope your birthday is really wonderful and this coming year is the best yet!" << std::endl;
std::cout << "Love," << std::endl << std::endl;
std::cout << "John Doe" << std::endl;
}
// Go through every address, print out anniversary cards with the same date as string passed in
void AddressBook::generateAnniversaryCards(std::string date)
{
// Go through every address
Node* it = head;
while (it != NULL)
{
// If it's anniversary is equal to the date passed in, print it's birthday card
Address curAddress = ((Address)it->val);
if (date == curAddress.anniversaryDate)
{
printAnniversaryCard(curAddress);
}
it = it->next;
}
}
// Add an address to the book, make sure it is sorted alphabetically
void AddressBook::add(Address newAddress)
{
// Create a new node with our address
Node* newNode = new Node;
newNode->val = newAddress;
newNode->next = NULL;
// IF the head is null, the head and tail are both the new node
if (head == NULL)
{
head = tail = newNode;
}
else
{
// Create an iterator, and previous iterator
Node* prev = NULL;
Node* it = head;
// Go through each element
while (it != NULL)
{
// If the current name is greater than the new name,
std::string curName = ((Address)it->val).name;
if (curName > newAddress.name)
{
// Add the new node at the last location, then rewire the list
if (prev == NULL)
{
head = newNode;
newNode->next = it;
}
else
{
prev->next = newNode;
newNode->next = it;
}
return;
}
else
{
// Else we go to the next node
if (it->next == NULL)
{
it->next = newNode;
it = newNode->next;
}
else
{
prev = it;
it = it->next;
}
}
}
}
// Increment the length when an item is added
length++;
}
void AddressBook::printAnniversaryCard(Address curAddress)
{
std::cout << "-------------------------------------" << std::endl;
std::cout << "Dear " << curAddress.name << "," << std::endl << std::endl;
std::cout << "Hope your anniversary is really wonderful and this coming year is the best yet!" << std::endl;
std::cout << "Love," << std::endl << std::endl;
std::cout << "John Doe" << std::endl;
}
// Loads the address book from a properties file
void AddressBook::load()
{
// Attempt to open the address file
std::ifstream in;
in.open("addresses.properties");
// If the file didn't open, print an error message
if (!in.good())
{
std::cerr << "Cannot open addresses.properties" << std::endl;
return;
}
// While we can read without an error, keep adding addresses to our book
while (in.good())
{
// Create new address, get all 4 elements
Address* curAddress = new Address;
std::string temp;
getline(in, temp);
curAddress->name = temp;
getline(in, temp);
curAddress->streetAddress = temp;
getline(in, temp);
curAddress->anniversaryDate = temp;
getline(in, temp);
curAddress->birthdayDate = temp;
// If our input is still good after adding those 4 elements, add the new address.
if (in.good())
{
add(*curAddress);
}
}
// Close the file stream
in.close();
}
// Save the address book
void AddressBook::save()
{
// Open the properties file for our address book
std::ofstream out;
out.open("addresses.properties");
// If it failed to open, print an error and end.
if (!out.good())
{
std::cerr << "Cannot open addresses.properties" << std::endl;
return;
}
// For every address
Node* it = head;
while (it != NULL)
{
// Print it's information to a new line of the text file
Address curAddress = (Address)it->val;
out << curAddress.name << std::endl;
out << curAddress.streetAddress << std::endl;
out << curAddress.anniversaryDate << std::endl;
out << curAddress.birthdayDate << std::endl;
// Go to the next node
it = it->next;
}
// Close the outstream
out.close();
}
Errors:
1)I get 13 errors.... most of them about the "getline" identifier: error C3861: 'getline': identifier not found.
2)error C2679: binary '>>': no operator found which takes a right-hand operand of type 'std::string' (or there is no acceptable conversion) Severity Code Description Project File Line Suppression State Warning.
3)C4717 'operator<<': recursive on all control paths, function will cause runtime stack overflow borra_last ast\address.cpp 24
Step by Step Solution
There are 3 Steps involved in it
Step: 1
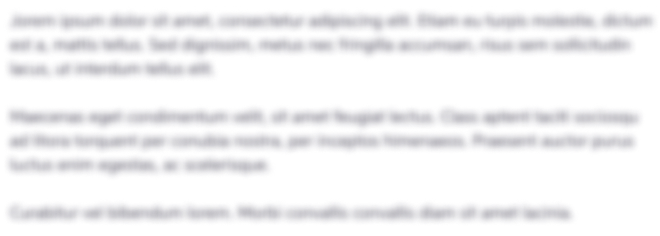
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started