Question
C++ Program: In this lab, you will be examining a set of temperatures collected over a twenty four hour period. Be sure to make use
C++ Program:
In this lab, you will be examining a set of temperatures collected over a twenty four hour period. Be sure to make use of an array to store these temperatures. You will be required to read in the data points from a file. After reading in the data, you will need to write a segment of code that will find the average of the data set. Make sure to print out the average in main. You will also need to write code to display the temperatures in the table format (on the screen) shown below.
Table Format:
Hour Temperature Difference from the Average 0 35 +/- ??? 1 32 +/- ??? |
After you finish printing out the average and the data in the format shown above, you need to write additional code that will find the lowest temperature in the array. You could sort the data, and grab the piece of data in the first position. However, this will result in no credit. (We will cover sorting the array in two more weeks.) You will have to use a linear search to find the lowest temperature. Once you find it, print out the value you found. In addition to printing out the value, please print out the position where the value was found. This can be done by having a for loop run through the array and search for the value using an if statement such as:
int low = tempArray[0];
int lowPosition = 0;
for (int i = 0; i < sizeOfArray; ++i)
if (tempArray[i] < low) { low = tempArray[i]; lowPosition = i; } |
Once you have written this code, write additional code that does the same thing to find the highest value and its position in the array. Print both the lowest and highest value and the position where it was found in the array. Put this output on the screen after the table that you have already printed on the screen.
Data:
Below is the set of data that you should include in your text file. Remember! Your input text file must be in the same folder as your cpp file.
35 32 31 28 25 22 23 25 26 30 32 38 41 43 45 48 52 55 49 44 43 42 49 37
Step by Step Solution
There are 3 Steps involved in it
Step: 1
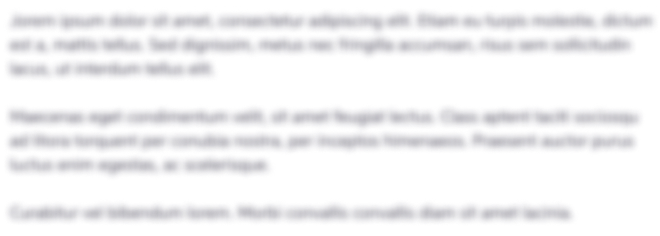
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started