Question
c program Introduction Steganography refers to hiding information such as text/images/data within another file/message/data with the intent of concealing the existence of the hidden information.
c program
Introduction
Steganography refers to hiding information such as text/images/data within another file/message/data with the intent of concealing the existence of the hidden information. A common method for this is hiding data within the least significant bits of the bytes representing colours in an image. The LSB can be represented as 0b00000001, 0x01 or 1 and the MSB can be represented as 0b10000000, 0x80 or 128.
You are to write a program that can be used to hide data from a supplied file in the low bits of a supplied image file. The same program will also be able to check the size of hidden data, extract the data and verify, using a checksum, the integrity of the hidden data in an image.
Encoding
Bitmap files (*.bmp) are a relatively simple file format. The file consists of a header containing metadata about the pixel format and image size and is m bytes long. The pixel data follows in the remaining l bytes, the size of which can be calculated from the information in the header. The basic specification only requires you handle these files and a bitmap module is supplied to assist in getting the length of the header in bytes and the length of the pixel data in bytes.
The program shall only modify the data relating to pixel bytes and shall copy the header information required for the image file format without modification. Concealing a single byte within the pixel data is depicted in the above diagram. The MSB of concealed data shall be placed in lower index pixel bytes and the LSB in higher index pixel bytes. Where multiple bits are concealed per byte of pixel data (as in a following diagram) the more significant bits of concealed data shall be placed in more significant bits of the pixel byte. Where there are insufficient bits of the current byte of concealed data to fill the required number of bits in the pixel byte the most significant bit(s) of the next byte of concealed data shall be utilised.
The format of the concealed data consists of a 32 bit unsigned integer giving the length of the data payload, the data payload and a 32 bit unsigned integer checksum of the data payload. The data length and checksum parameters are always encoded as 1 concealed bit per byte of pixel data. The concealed data is encoded as the minimum number of concealed bits per byte of pixel data to completely encode the payload. This may require 2, 3 or even 8 bits concealed per byte of pixel data. Where multiple bits are required per byte more significant bits of concealed data are encoded in the more significant bits of the pixel data as shown in a following diagram where concealing 3 bits per byte of pixel data is depicted. Once all the bytes of the data payload have been concealed remaining bits in the current pixel byte shall be set to 0 and remaining pixel bytes (until the last 32 bytes for the checksum) shall be copied unmodified.
Given o bytes of data to conceal the output image file that has l bytes of pixel data shall have:
m bytes of header data directly copied from the input source image file.
32 bytes of pixel data with the length of the data payload concealed 1 bit per byte.
p bytes of modified pixel data
r bits of concealed data per pixel byte shall be used where,
r is the minimum number of bits per pixel such that the concealed data can fit within the available pixel values.
r is an integer value such that the following is true (r - 1) * (l - 64) = (o * 8)
If (r * p) != (o * 8) the 'concealed' bits will be append with s '0' bits such that (r * p) == (s + o * 8).
q bytes of unmodified pixel data.
32 bytes of pixel data with the checksum of the data payload concealed 1 bit per byte.
Once all the concealed data is encoded the remaining bytes are copied unmodified until the checksum. If at the end of the concealed data there are insufficient bits to fill the last pixel byte (as in the above diagram) the remaining bits shall be set to 0.
Basic Specification (75%)
The program shall comply with the following specification:
1. Compilation & Code
The program shall be written in C and compiled with GCC using the C11 standard; the compiled executable shall be named steg. Compilation flags shall include "-Wall -Wextra -Wpedantic -fsanitize=address -std=c11". Additional flags that do not remove/undo the effects of these flags shall be permitted.
Compilation shall be through a Makefile that has steg and clean as targets.
All comments in the source code shall be written in English.
2. Error Handling
All error messages, which are messages starting with "Error:", shall be printed to the terminal using stderr.
Where the specification states "exit immediately with error code n" this is to mean no further text shall be printed to the terminal and the return statement in main shall return n. All allocated memory shall be properly freed.
Errors shall be responded to in the following order:
Left to right for command line options.
Missing inputs before existing outputs.
Payload size relative to image size.
Checksum verification.
If no errors occur the program shall return an error code of 0.
3. Command Line Options
The program will accept 1, 2 or 3 non-option arguments (arguments after and including the first argument that doesn't start with -). The following points specify the differing behaviour of the program with each number of arguments.
Optional arguments may modify the behaviour of the program and shall be given before any non-option arguments.
If the program is given zero non-option arguments it shall print Error: No file name provided. and exit immediately with error code 7.
If the program is given more than three non-option arguments it shall print Error: Too many file names provided. and exit immediately with error code 6.
If the program is given three non-option arguments it shall treat the first non-option argument as the name of an input source image file, the second non-option argument as the name of an input source data file and the third non-option argument as the name of an output destination image file. It shall then embed the contents of the input source data file in the input source image pixels and output to the destination image file the modified pixel values and unmodified file format/header information.
If the program is given two non-option arguments it shall treat the first non-option argument as an input image file with encoded data and the second non-option argument as an output data file. It shall then extract the contents of the input image file to the output data file.
If the program is given one non-option argument it shall treat it as an input image file with encoded data and only decode if another option is specified.
The program shall accept the optional argument -h which shall cause the program to print the specified help message and immediately exit with error code 1. The help message is:
Usage: steg [-vncp] source_image [[data_file] [output_image]]
Steganographically conceal/extract data from an image.
With three arguments will conceal data from data_file within source_image and
output to output_image. One and two argument versions inspect (and optionally save)
data from an image with concealed data.
-c Print the checksum of the concealed data.
-h Print this help message.
-n Print the number of concealed bytes.
-p Print the contents of a concealed image/data file when concealing. Assuming text data.
-v Verify checksum of an image with concealed data. Invalid if an output image is specified.
The program shall accept the optional argument -n which shall cause the program to print the length of hidden data in bytes, in the format Hidden data length: 45643 bytes. .
The program shall accept the optional argument -c which shall cause the program to print the checksum of the hidden data as a 32-bit hex number in the format Checksum: 0x0400F50F. . When the input image contains hidden data, as signified by a command line containing 1 or 2 non-option arguments, this checksum value shall be read from the stored checksum. When creating an output image containing hidden data the checksum value printed shall be checksum calculated from the input data.
The program shall accept the option argument -p which shall cause the program to print the hidden data (treating it as a text file/string) between two lines that each consist of 80 hyphens:
--------------------------------------------------------------------------------
This is the contents of a text file. The first line was very long and did not end at 80 characters.
This file ended with a new line character and means there is a blank line before the hyphens.
--------------------------------------------------------------------------------
The program shall accept the optional argument -v which shall cause the program to verify the checksum with the data. The -v optional argument shall be valid only with one and two non-option argument invocations of the program. If the optional argument -vIis used with the three non-option argument invocation of the program the program shall print Error: -v invalid when concealing data. and exit immediately with error code 11. If the calculated checksum differs from the stored checksum the program shall print Error: Checksum mismatch. and exit immediately with error code 12. If the calculated checksum matches the stored checksum the program shall print Checksums match. .
If the program finds an optional argument that it is not known it shall print Error: Unknown option -%s. and immediately exit with error code 8.
Output/effects from processing optional arguments shall occur after any output files have been written and will occur in the order -p, -n, -c, -v.
4. File Handling
If a nominated input file does not exist the program shall print an error message Error: Input file %s was not found. and exit immediately with error code 2. The token %s shall be replaced with the name of the file that was nominated.
If a nominated output file exists the program shall inquire of the user Output file %s already exists. Overwrite (y)? If an affirmative answer (y, yes - case insensitive) is received, the program shall continue and overwrite the file. If the answer is negative (n, no - case insensitive) the program shall emit no further messages and exit immediately with error code 3. If any other value is received the program shall ask the question again.
If the data to be encoded cannot fit within the source image pixel values (see encoding details above) the program shall print Error: Input data too large to hide within source image. and exit immediately with error code 5.
If when attempting to decode an input image file the decoded data size is too large to be concealed within the image file the program shall print an error message Error: Image file too small to conceal %i bytes of data. and exit immediately with error code 9.
Checksum
A checksum is a method to check if data has been modified or changed. It is a function of the data and is generally sensitive to changes of a single bit. As we are storing data within an image we need a way to verify that it has not been corrupted in an image editing program. To this end we have devised a relatively simple checksum to use.
The data payload shall be broken into 16-bit unsigned short integers using little endian ordering.
Each short shall be squared (multiplied by itself) and the result stored in an unsigned integer (32-bit).
The result of the squaring operation shall be successively XOR'd (^ operator) to get the final checksum.
If the data payload has an odd number of bytes an additional zero byte shall be appended onto the end for the purposes of calculating the checksum but shall not be stored/concealed within the pixel bytes.
hih2 di TIL hih2 di TILStep by Step Solution
There are 3 Steps involved in it
Step: 1
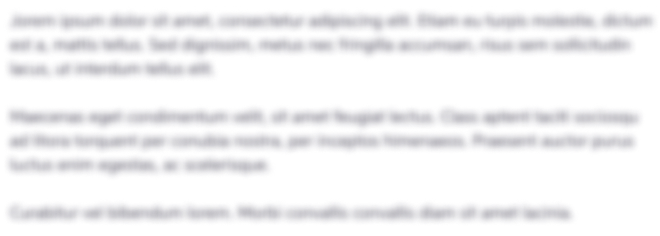
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started