Question
c program linked list #include #include // struct ndoe *previous; } Node; Node* insert(Node* listHead, int dataToInsert) { // we need to return a new
c program linked list
#include struct ndoe *previous; } Node; Node* insert(Node* listHead, int dataToInsert) { // we need to return a new head of linkedlist because // the head may change after insertion if (listHead == NULL) { // list is emtpy, let's insert our first element! printf("inserting first element with data %d ",dataToInsert); Node *newNode = malloc(sizeof(Node)); newNode->data = dataToInsert; newNode->next = NULL; return newNode; } else { // list is not empty, let's insert at the end printf("inserting element with data %d ",dataToInsert); Node *current = listHead; // CAUTION! while (current != NULL) { if (current->next == NULL) { // if it's end of list... Node *newNode = malloc(sizeof(Node)); newNode->data = dataToInsert; newNode->next = NULL; current->next = newNode; return listHead; } else { // otherwise... current = current->next; } } } } void printList(Node* listHead) { printf("printing list: "); Node *current = listHead; while (current != NULL) { printf("%d ",current->data); current = current->next; } printf(" "); } Node* deleteSpecific(Node *listHead, int dataValue) { // assume data to delete always exist and is always unique in // this exercise printf("deleting %d from list ", dataValue); struct node *ptr,*preptr; ptr = listHead; if(ptr ->data ==dataValue) { listHead = deleteFirst(listHead); return listHead; } else { while (ptr->data != dataValue) { preptr = ptr; ptr = ptr->next; } preptr->next = ptr->next; free(ptr); return listHead; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
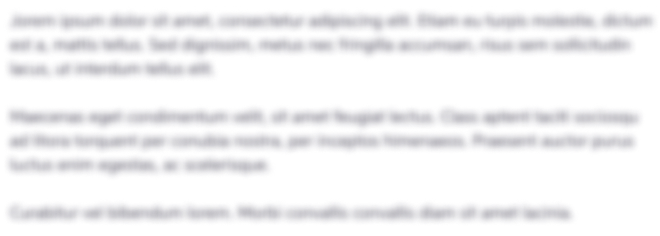
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started