Question
C++ program, No input is needed. Program should output the following: #ENROLL A B C D F AVG-GPA 102 nn nn.n% nn.n% nn.n% nn.n% nn.n%
C++ program,
No input is needed.
Program should output the following:
#ENROLL A B C D F AVG-GPA 102 nn nn.n% nn.n% nn.n% nn.n% nn.n% n.nn 104 nn nn.n% nn.n% nn.n% nn.n% nn.n% n.nn 204 nn nn.n% nn.n% nn.n% nn.n% nn.n% n.nn ...
Generate 300 students, and each student should have randomly generated first name, last name, ssid, and should have a bag of courses, with 3-6 courses, and each course has randomly generated midterm1, midterm2, and final exam grade.
The following set of courses are offered: 102, 104, 204, 210, 212, 217, 220, 221, 301, 304, 317, 318, 322, 332, 335, 340, 412, 420, 450, 470, 480, 599
Student info should be retained in student class object,
Course info (m1, m2, finalexam, course id ) should be retained in course class object
Suppose also that every course has grading scheme of two midterm exams and a final exam. Instructor of each course will assign letter grades { A, B, C, D, F } based on the total score of these exams A, B, C, D, and F should be assigned respectively to the top 15%, 16-35%, 36-60%, 61-80%, and the rest.:
Use the following bag template class:
[code]
using namespace std; templateclass Bag { public: // TYPEDEFS and MEMBER CONSTANTS typedef T value_type; typedef std::size_t size_type; static const size_type DEFAULT_CAPACITY = 30; // CONSTRUCTORS and DESTRUCTOR Bag(size_type initial_capacity = DEFAULT_CAPACITY); Bag(const Bag& source); void operator =(const Bag& source); ~Bag() { delete [] data; } // MODIFICATION MEMBER FUNCTIONS void reserve(size_type new_capacity); bool erase_one(const value_type& target); size_type erase(const value_type& target); void insert(const value_type& entry); void operator +=(const Bag& addend); // CONSTANT MEMBER FUNCTIONS size_type size( ) const { return used; } size_type count(const value_type& target) const; // SIMPLE ITERATOR void begin() { current = 0; } bool end() const { return current >= used; } int operator++(int) { assert(!end()); current++; } T& get() { assert(!end()); return data[current]; } private: value_type *data; // Pointer to partially filled dynamic array size_type used; // How much of array is being used size_type capacity; // Current capacity of the Bag size_type current; // Iterator's current position. }; // NONMEMBER FUNCTIONS for the Bag class template Bag operator +(const Bag & b1, const Bag & b2); template const typename Bag ::size_type Bag ::DEFAULT_CAPACITY; template Bag :: Bag(size_type initial_capacity) { data = new T[initial_capacity]; capacity = initial_capacity; used = 0; } template Bag ::Bag(const Bag & source) // Library facilities used: algorithm { data = new T[source.capacity]; capacity = source.capacity; used = source.used; copy(source.data, source.data + used, data); } template void Bag ::reserve(size_type new_capacity) // Library facilities used: algorithm { value_type *larger_array; if (new_capacity == capacity) return; // The allocated memory is already the right size. if (new_capacity < used) new_capacity = used; // Cant allocate less than we are using. larger_array = new value_type[new_capacity]; copy(data, data + used, larger_array); delete [ ] data; data = larger_array; capacity = new_capacity; } template typename Bag ::size_type Bag ::erase(const value_type& target) { size_type index = 0; size_type many_removed = 0; while (index < used) { if (data[index] == target) { --used; data[index] = data[used]; ++many_removed; } else ++index; } return many_removed; } template bool Bag ::erase_one(const value_type& target) { size_type index; // The location of target in the data array // First, set index to the location of target in the data array, // which could be as small as 0 or as large as used-1. // If target is not in the array, then index will be set equal to used. index = 0; while ((index < used) && (data[index] != target)) ++index; if (index == used) // target isn't in the Bag, so no work to do return false; // When execution reaches here, target is in the Bag at data[index]. // So, reduce used by 1 and copy the last item onto data[index]. --used; data[index] = data[used]; return true; } template void Bag ::insert(const value_type& entry) { if (used == capacity) reserve(used+1); data[used] = entry; ++used; } template void Bag ::operator +=(const Bag & addend) // Library facilities used: algorithm { if (used + addend.used > capacity) reserve(used + addend.used); copy(addend.data, addend.data + addend.used, data + used); used += addend.used; } template void Bag ::operator =(const Bag & source) // Library facilities used: algorithm { value_type *new_data; // Check for possible self-assignment: if (this == &source) return; // If needed, allocate an array with a different size: if (capacity != source.capacity) { new_data = new value_type[source.capacity]; delete [ ] data; data = new_data; capacity = source.capacity; } // Copy the data from the source array: used = source.used; copy(source.data, source.data + used, data); } template typename Bag ::size_type Bag ::count(const value_type& target) const { size_type answer; size_type i; answer = 0; for (i = 0; i < used; ++i) if (target == data[i]) ++answer; return answer; } template Bag operator +(const Bag & b1, const Bag & b2) { Bag answer(b1.size( ) + b2.size( )); answer += b1; answer += b2; return answer; }
[/code]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
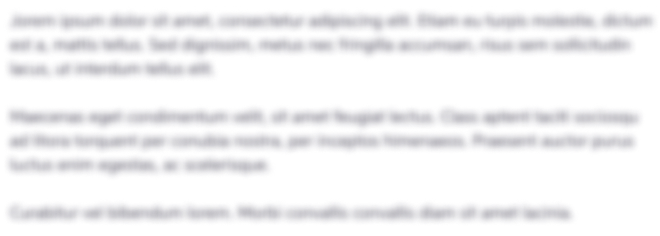
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started