Question
C Programming: Define a basic HealthProfile structure for a school by using C programming . Use the keyword typedef in the declaration of the structure
C Programming:
Define a basic HealthProfile structure for a school by using C programming. Use the keyword typedef in the declaration of the structure for simplicity. The structures members should include the name (e.g, Jane Doe), students ID (e.g., 10001), gender (a letter M or F), month of birth (1~12), day of birth (1~31), and year of birth (1900~2020). This structure must contain a member variable that is a pointer to the structure type itself.
Tasks:
- In the main() function, declare a pointer to the Profile structure type, and initialize this pointer using the malloc() function in the C standard library. Declare other variables if needed
- Have the first function that prompts for the name, and receives the data and uses it to set the corresponding member of the Profile structure created in step 1 through a pointer
- A second function prompts for the gender, and receives the data and uses it to set the corresponding member of the Profile structure created in step 1 through a pointer
- Third function prompts for the ID, and receives the data and uses it to set the corresponding member of the Profile structure created in step 1 through a pointer
- Fourth function that prompts for the patients date of birth, receives the month of birth (1~12), day of birth (1~31), and year of birth (2019) in pointer parameters. The return type of this function must be void.
- All the above functions should be called at least once in the main() function. At the end of the main() function, prints all the information of a patient by using the pointer to the Profile structure type (created in step 1)
Copyable code:
#include
//A structure to hold health profile information typedef struct{ char lastName[30]; char firstName[30]; int id; char gender[10]; int monthOfBirth; int dayOfBirth; int yearOfBirth; } HealthProfile;
//Name in char and recieves first and last name of the patient void setName(HealthProfile *HPP){ char lastName; char firstName; printf("Enter patient's first and last name: ") scanf("%c", %c) }
//ID is an int and HPP is a pointer used to assign variable void setID(HealthProfile *HPP){ int id; printf("Enter patient's ID: "); scanf("%d", %id); }
//Gender receives gender information for patient's profile void setGender(HealthProfile *HPP){ char gender; printf("Enter patient's gender: "); scanf("%c", %c); }
void setBD(int*month, int*day, int*year){ printf("Enter patient's DOB: ")
}
//Main function prints out of my patient's information int main(){ // HealthProfile *HPP = malloc(sizeof(HealthProfile)); printf(" Name:\t %s ", ); printf("ID:\t %d ", ); printf("Gender:\t %c ", ); printf("DOB:\t %d-%d-%d ", ); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
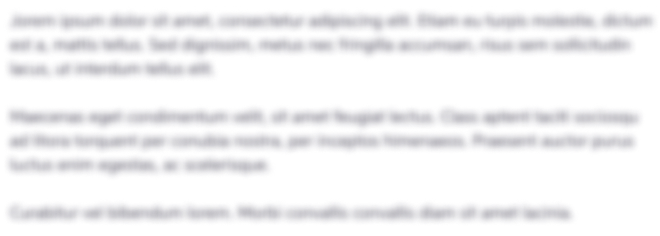
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started