Question
C++ Programming From Problem Analysis to Program Design pages 813-814 #6 In Programming Exercise 2, the class dateType was designed and implemented to keep track
C++ Programming From Problem Analysis to Program Design
pages 813-814 #6
In Programming Exercise 2, the class dateType was designed and implemented to keep track of a date, but it has very limited operations. Redefine the class dateType so that it can perform the following operations on a date, in addition to the operations already defined:
a. Set the month.
b. Set the day.
c. Set the year.
d. Return the month.
e. Return the day.
f. Return the year.
g. Test whether the year is a leap year.
h. Return the number of days in the month. For example, if the date is 3-12-2019, the number of days to be returned is 31 because there are 31 days in March.
i. Return the number of days passed in the year. For example, if the date is 3-18-2019, the number of days passed in the year is 77. Note that the number of days returned also includes the current day.
j. Return the number of days remaining in the year. For example, if the date is 3-18-2019, the number of days remaining in the year is 288.
k. Calculate the new date by adding a fixed number of days to the date. For example, if the date is 3-18-2019 and the days to be added are 25, the new date is 4-12-2019.
I've taken a stab at it and still come up with some problems in my code and would like some help in how to correct these errors just so I could fully complete the Programming Exercise.
I have provided the code below.
main.cpp
//Preprocessor directives, header functions #include
//Header file for dateType #include "DATETYPE.H" //Header file for setDate #include "SETDATE.H"
//Standard Library using namespace std;
int main() {//Begin void function main
int m, d, y; dateType date(0,0,0); //Constructor call
//Friendly message explaining the purpose of the program cout << "Hello There! " << endl;
cout << "This program's objective is to prove a leap year is a leap year" << endl; cout << "Another purpose is for the test program to test the class of this program" << endl;
cout << endl;
//Prompt the user to enter the values cout << "Please enter the Month : "; cin >> m;
cout << "Please enter the Day : "; cin >> d;
cout << "Please enter the Year : "; cin >> y;
date.setDate(m, d, y);
cout << endl; bool check = date.isLeapYear();
if (check) cout << "Year : Leap year";
cout << endl; cout << "Print year : "; date.printDate();
return 0; }//end main
dateTypeImp.cpp
//Preprocessor Directives #include
//dateType header file #include "DATETYPE.H" #include "SETDATE.H" //Standard Library using namespace std;
//Member function definitions int dateType::setMonth(int month) { int y; y = dYear;
if (month <= 12) {//condition to check whether the month is valid
//condition for month dMonth = month; switch(month) { //This is for the number of days in each month case 1: case 3: case 5: case 7: case 8: case 10: case 12: noofdays = 31; break; case 4: case 6: case 9: case 11: noofdays = 30; break; case 2: if (isLeapYear(year)) noofdays = 29; else noofdays = 28;
} } else { cout << "Invalid Month" << endl; dMonth = 0; } if(day <= noofdays) {//condition for days based on the number of days in a month //part b dDay = day; } //return number of days return noofdays; }
//Defining the function to set the day void dateType::setDay(int day) { //begin loop if (d <= noofdays) { d = d; } else { cout << "Invalid Day" << endl; d = 0; } } //Defining the function to set the year void dateType::setYear(int year) { dYear = year; }
}//End setYear
bool dateType::isLeapYear(int year ) { //Function to conform whether year is leap year or not if (year % 4 == 0) return 1; else return 0; }
//Part i //Defining the function to find the total number of passed days int dateType::TotalnumberofPassedDays(int m, int d) { int i, passedDays = 0; for (i = 1;i; i++) { if (i == m) { noofdays = noofdays + 1; } else passedDays += setMonth(i); } passedDays += dDay; numofPasseddays = passedDays; //return the passed days value return passedDays; }
//Part j //Defining the function to find the remaining days int dateType::Remainingdays(int y) { int remainingDays; //Begin if statement to find the days if (isLeapYear(y)) { remainingDays = 366 - numofPasseddays; } else remainingDays = 365 - numofPasseddays; return remainingDays; }
void dateType::CreateNewDate(int m, int d, int y, int dayadd) {//Definition of the function to create the new date if ((dDay + dayadd) % m) { dDay = dDay + dayadd; dDay = abs(dDay - 31); dDay = d; m++; dMonth = m; dYear = y; if (m > 12) { dMonth = 1; dYear++; } } }
void dateType::printDate()const { cout << "Date:DD-MM-YYYY" << dDay << "-" << dMonth << "-" << dYear << endl;
}
setdate.h
//Note:I replaced setdate with setyear in order //to make it easier to manage with finding/ identifying the leap year // and also because I copied it over from Programming Exercise #2.
//Standard Library using namespace std;
//part d int dateType::getMonth()const { return dMonth; };
//part e int dateType::getDay()const { return dDay; }
//part f int dateType::getYear() const { return dYear; }
datetype.h
//Standard Library using namespace std;
class dateType { private: int dMonth; //Variable to store the month int dDay; //variable to store the day int dYear; //variable to store the year int noofdays; //variable to store the number of days int numofPasseddays; //variable to store the number of passed days
public: //Member function declaration int setMonth(int month); void setYear(int day, int year); //Function to set the date. //The member variables dMonth, dDay, and dYear are set //according to parameters. //PostCondition: dMonth = month; dDay = day; dYear = year;
int getDay()const; //Function to return the day. //Postcondition: The value of dDay is returned. int getMonth()const; //Function to return the month. //Postcondition: The value of dMonth is returned.
int getYear()const; //Function to return the year. //Postcondition: The value of dYear is returned.
void printDate()const; //Function to output the date in the form mm-dd-yyy.
bool isLeapYear(int year); //Function to check if the year is LeapYear //Postcondition: bool value indicating leap year
int TotalnumberofPassedDays(int,int); //Function to find the total number of passed days
int Remainingdays(int); //Function to find the remaining number of days
void CreateNewDate(int,int,int,int); //Function to crease the new date
dateType(int month = 0, int day = 0, int year = 0);//Contructor };
//Constructor dateType::dateType(int month, int day, int year) { dMonth = month; dDay = day; dYear = year; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
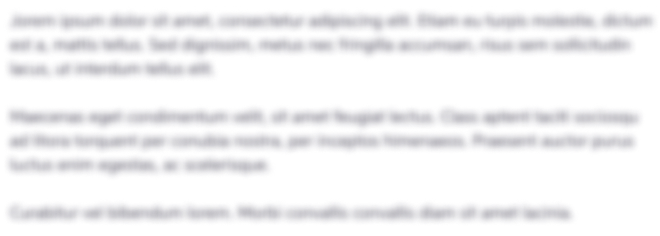
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started