Question
C Programming Instructions: Make sure your #1 goal is readability. Comment on each problem in your code so it's easy to grade. For example: //
C Programming
Instructions:
Make sure your #1 goal is readability.
Comment on each problem in your code so it's easy to grade. For example:
// --------------------------------------------------------------------------------
// Problem #1 Print all the whole numbers from 1 to 100.
// --------------------------------------------------------------------------------
printf( "Problem #1 Print all the whole numbers from 1 to 100. " );
...
Problem(s):
Put the following in one program:
1- Write a loop that will print all the whole numbers from 1 and 100.
2- Write a loop that will add all the whole numbers from 1 and 100 and print the total.
3- Write a loop that will add all the ODD whole numbers from 7 and 313 and print the total.
4- Write a loop that will add all the EVEN whole numbers from -2 and -146 and print the total.
5- Write a loop that will add every 3rd number from 2000 and -60 and print the total.
6- Write a loop that will prompt the user to enter a number from 1 to 100. The program will continue to loop until a number within that range is entered. After the loop, print out the square root of the number. Be sure to test the loop by entering numbers outside the range.
7- Write a loop that will prompt the user to enter test scores from 0 to 100. The program will continue to loop until a -1 is entered. Sum all test scores entered that are in the range 0 to 100 (inclusive). After the loop, calculate the average and print out the letter grade. Assume a 10-point grading scale. For example:
Enter a test score from 0 to 100 (-1 to end): 70
Enter a test score from 0 to 100 (-1 to end): 80
Enter a test score from 0 to 100 (-1 to end): 90
Enter a test score from 0 to 100 (-1 to end): -1
The average was 80 and the letter grade is a B.
8- If we list all the natural numbers below 10 that are multiples of 3 or 5, we get 3, 5, 6 and 9. The sum of these multiples is 23. Write the code that will calculate and print the sum of all the multiples of 3 or 5 below 1000.
9- Write a loop that will prompt the user to enter an uppercase letter. The code should continue to loop until an uppercase letter is entered. After an uppercase letter is entered print out the letter in both uppercase and lowercase. You can't use the built-in tolower function. For example:
Enter an uppercase letter: 5
Enter an uppercase letter: X
Here is the letter in uppercase X and lowercase x.
Extra Credit:
1- Hangman. Make a constant in your code with a value from 1 to 100. Prompt the user to guess the number. If the user guesses correctly end the game and display a "Congratulation. You won." message. If the guess is too high print "Too high." If the guess is too low print "Too low." Give the user at most eight guesses. If the user hasn't guessed the number after eight guesses print a "You lose." message.
2- A prime number is a number greater than one that is evenly divisible only by one and itself. The first few prime numbers are: 2, 3, 5, 7, 11, 13, 17, 19. Write the code that will prompt the user to enter a number from 2 to 100,000. Loop until a number in that range is entered. Print out if the number is prime or not.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
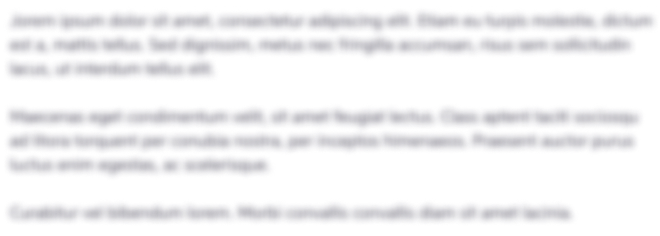
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started