Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C++ Programming Language Create a new project in Visual Studio named Lab06. Add a class named Dealership, a class named Car, and a source code
C++ Programming Language
Create a new project in Visual Studio named Lab06. Add a class named Dealership, a class named Car, and a source code file named lab06.cpp. Copy the following source code into source code files as required.
Complete the code as required. Instructions to complete the code are in the comments of the following source code in each part.
/* * Topic: Lab 6 - Composition * File name: Dealership.h */
#pragma once #include "Car.h" class Dealership { private: string name; Car** inventory; int carCount; int brandCount; int modelCount; public: Dealership(); Dealership(string); ~Dealership(); void setName(string); void addCarToInventory(string,string); void sellCar(string, string); int getCarCount(); int getModelCount(); void orderInventory(); void printSummaryInventory(); void printDetailedInventory(); };
/* * Topic: Lab 6 - Composition * File name: Dealership.cpp */ #include "Dealership.h" // the default constructor initilizes strings to "" // integers to 0, and inventory to the null pointer Dealership::Dealership() { } // the parametrized constructor initilizes the string to // the parameter value, integers to 0, and inventory to the null pointer Dealership::Dealership(string) { } // destroys the Dealership object, and all car objects // associated with it. Prints the phrase // "Dealership [name] has been closed" // Use a cycle to 'destroy' all cars in inventory Dealership::~Dealership() { } // set the dealership's name to the parameter's value void Dealership::setName(string); // adds a Car object to the inventory by creating // a new Car object using the parameters as data // fed to the constructor void Dealership::addCarToInventory(string, string); // removes a Car object from the inventory void Dealership::sellCar(string, string); // returns the count of cars in inventory int Dealership::getCarCount() const; // returns the count of a single model of cars in inventory int Dealership::getModelCount() const; // orders the inventory by brand name and then by model name // HINT: can use the serial number value! void Dealership::orderInventory(); // prints the dealer's inventory by brand an model // format is brand name, model name, model count void Dealership::printSummaryInventory() const; // prints the dealer's detailed inventory in the form // serial number, brand name, model name void Dealership::printDetailedInventory() const;
/* * Topic: Lab 6 - Composition * File name: Car.h */ #pragma once #include using namespace std; class Car { private: string brand; string model; string serialNumber; static int count; void setSerialNumber(); public: Car(); Car(string, string); ~Car(); void setBrand(string); void setModel(string); string getBrand() const; string getModel() const; string getSerialNumber() const; int getCount() const; void printCar() const; };
/* * Topic: Lab 6 - Composition * File name: Car.cpp */ #include "Car.h" // initialize static variable to 0 // The default constructor initializes strings to "" and adds 1 to count Car::Car() { } // The parametrized constructor initializes the name and brand fields // to the parameter value and adds 1 to count // calls the method to set the serial number Car::Car(string, string) { } // destroys a Car object and prints the phrase // "Car [brand name] [model name], serial number [serial number] has been removed" Car::~Car() { } // this is a private method which can only be invoked by the object itself // sets the serial number for each new Car object // the serial number is composed of the first two letters of the brand and model // with the count value, in three digits, appended at the end. // for example, if brand is Kia, model is Rio, and count is 1 // the serialnumber would be KiRi001 void Car::setSerialNumber() { } // Sets the brand field to the parameter value void Car::setBrand(string) { } // Sets the model field to the parameter value void Car::setModel(string) { } // returns the brand field's value string Car::getBrand() const { } // returns the model field's value string Car::getModel() const { } // returns a car's serial number string Car::getSerialNumber() const { } // returns the count of Car objects created int Car::getCount() const { } // prints the details about each car in the form // serial number, brand name, model name void Car::printCar() const { }
/* * Topic: Lab 6 - Composition * File name: lab06.cpp */ // which include statement is required? int main() { // create a Dealership object named Poli Auto Sales // add 10 cars to the inventory, calling the method // to print the detailed inventory after each car is added // call the method to print the summary inventory // sell three cars, calling the method to print the // summary inventory after each car is sold // call the method to print the detailed inventory }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
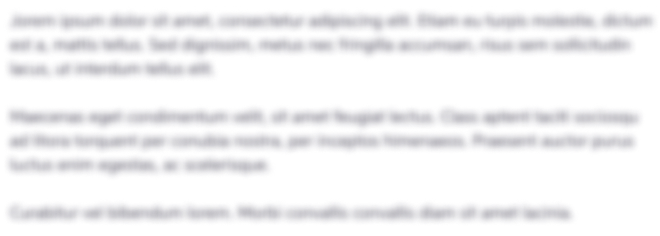
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started