Question
c++ programming let me know if you can do this grid.h and grid.cpp should already be in your working directory (if not, get files from
c++ programming let me know if you can do this
grid.h and grid.cpp should already be in your working directory (if not, get files from above)
Add Grid.cpp to your Source files
Create a new item to Source Files called 6B turnTestDriver.cpp
Include Grid.h in your 6B turnTestDriver.cpp using the following syntax: #include "Grid.h"
Also include
Open your Grid.h by selecting File / Open / File and select Grid.h
In Grid.h, add a new modifiers just below void turnLeft() (add: turnRight() and turnAround())
In Grid.cpp create the new methods (turnRight() and turnAround() ) in Grid.cpp (just below the turnLeft() method
(could just copy turnLeft(), change name, change code)
, if facing == west, facing = north
if facing == north, facing = south
Hint, hint: Type the code on page 168 into your turnTestDriver.cpp
in your
make calls to move the carrot to give the desired gri
I need this to be done in c++ and I need help printing the output
Grid.h file code
// File: grid // From: Computing Fundamentals with C++, Second Edition // Rick Mercer, Franklin, Beedle, and Associates #ifndef GRID_H #define GRID_H const int maxRows = 22; const int maxCols = 36; enum Direction { north, east, south, west }; /////////////////////////////////////////////////////////////////////////// // The grid class definition ////////////////////////////////////////////// /////////////////////////////////////////////////////////////////////////// class Grid { public: // -- Constructors Grid(); Grid(int totalRows, int totalCols); Grid(int totalRows, int totalCols, int startRow, int startCol, Direction startDirection); // -- Modifiers void move(); void move(int spaces); void turnLeft(); void putDown(); void putDown(int putDownRow, int putDownCol); void toggleShowPath(); void pickUp(); void block(int blockRow, int blockCol); // pre: The intersection at (blockRow, blockCol) has nothing at all // on it, not even the mover // post: The intersection at (blockRow, blockCol) is blocked. The // mover cannot move into this intersection // -- Accessors bool frontIsClear() const; bool rightIsClear() const; int row() const; int column() const; int nRows() const; int nColumns() const; void display() const; private: int lastRow; // the number of the last row int lastCol; // the number of the last column char rectangle[maxRows][maxCols]; int currentRow, currentCol; // where the mover is char icon; // the symbol in the currentRow, currentCol Direction facing; // Direction.north .. Direction.west int showPath; // whether or not the path is shown void checkForBlock(int r, int c); // used by the implementation only void setIcon(); // used in implementation only }; #endif // GRID_H
2nd half This is the Grid.cpp code
// File: Grid.cpp // From: Computing Fundamentals with C++, 3rd Edition // Rick Mercer // Franklin, Beedle, and Associates #include // for function assert #include // for function exit, rand() and srand() #include // for TIME #include // for endl and the objects cout, cin #include "Grid.h" /////////////////////////////////////////////////////////////////////////// ///// IMPLEMENT THE MEMBER FUNCTIONS ////////////////////////////////////// /////////////////////////////////////////////////////////////////////////// // The constant definitions const char intersectionChar = '.'; const char beenThereChar = ' '; const char blockChar = '#'; const char thingHereChar = 'O'; const char moverOnThingChar = '&'; // -- constuctors Grid::Grid() // creates a 5 by 5 grid with mover in 0,0 facing east { int totalRows = 5, totalCols = 5, startRow = 0, startCol = 0; Direction startDirection = east; // Check that the boundaries are okay. assert(totalRows <= maxRows); assert(totalRows > 0); assert(totalCols <= maxCols); assert(totalCols > 0); // Check the initial position of the mover is within the Grid lastRow = totalRows - 1; lastCol = totalCols - 1; assert(startRow >= 0); assert(startCol >= 0); assert(startRow <= lastRow); assert(startCol <= lastCol); showPath = 1; // Show path when 1, when it's 0 keep the intersection visible int r, c; for (r = 0; r <= lastRow; r++) for (c = 0; c <= lastCol; c++) rectangle[r][c] = intersectionChar; currentRow = startRow; currentCol = startCol; facing = startDirection; setIcon(); rectangle[currentRow][currentCol] = icon; } Grid::Grid(int totalRows, int totalCols) { // Set up a border on the edges with one escape route int r, c; assert(totalRows <= maxRows); assert(totalRows >= 0); assert(totalCols <= maxCols); assert(totalCols > 0); showPath = 1; // Show path when 1, when it's 0 keep the intersection visible lastRow = totalRows - 1; lastCol = totalCols - 1; for (r = 0; r <= lastRow; r++) for (c = 0; c <= lastCol; c++) rectangle[r][c] = intersectionChar; for (c = 0; c <= lastCol; c++) { rectangle[0][c] = blockChar; // block first row rectangle[lastRow][c] = blockChar; // blocked last row } for (r = 0; r <= lastRow; r++) { rectangle[r][0] = blockChar; // block first column rectangle[r][lastCol] = blockChar; // block last column } // Put the mover somewhere in the Grid, but NOT a border srand((unsigned) time( NULL)); // use the clock to randomize currentRow = rand() % (lastRow - 1) + 1; currentCol = rand() % (lastCol - 1) + 1; // Pick a random direction int direct(rand() % 4); if (direct == 0) facing = north; else if (direct == 1) facing = east; else if (direct == 2) facing = south; else facing = west; setIcon(); rectangle[currentRow][currentCol] = icon; // Put one opening on any of the four edges if (rand() % 2 == 0) { // set on top or bottom at any column c = rand() % lastCol; if (c == 0) c++; // avoid upper and lower left corner exits (see below) if (c == lastCol) c--; // avoid upper and lower right corner exits (see below) if (rand() % 2 == 0) r = lastRow; // half the time. on the bottom else r = 0; // the other half, on the top } else { // set on left or right at any column r = rand() % lastRow; if (r == 0) // avoid upper right and left corner exits r++; if (r == lastRow) r--; // avoid lower left and lower right exits if (rand() % 2 == 0) c = lastCol; // half the time in the right column else c = 0; // the other half, put on left } rectangle[r][c] = intersectionChar; } Grid::Grid(int totalRows, int totalCols, int startRow, int startCol, Direction startDirection) { // Check that the boundaries are okay. assert(totalRows <= maxRows); assert(totalRows > 0); assert(totalCols <= maxCols); assert(totalCols > 0); // Check the initial position of the mover is within the Grid lastRow = totalRows - 1; lastCol = totalCols - 1; assert(startRow >= 0); assert(startCol >= 0); assert(startRow <= lastRow); assert(startCol <= lastCol); showPath = 1; // Show path when 1, when it's 0 keep the intersection visible int r, c; for (r = 0; r <= lastRow; r++) for (c = 0; c <= lastCol; c++) rectangle[r][c] = intersectionChar; currentRow = startRow; currentCol = startCol; facing = startDirection; setIcon(); rectangle[currentRow][currentCol] = icon; } // -accessors int Grid::row() const { return currentRow; } int Grid::column() const { return currentCol; } int Grid::nRows() const { // lastRow is the number of the last row as in 0..lastRow // so the total number of rows is one more than that return lastRow + 1; } int Grid::nColumns() const { // lastCol is the number of the last colukmn as in 0..lastCol // so the total number of columns is one more than that return lastCol + 1; } bool Grid::frontIsClear() const { switch (facing) { case north: if (currentRow == 0) return 0; else if (rectangle[currentRow - 1][currentCol] == blockChar) return 0; else return 1; case east: if (currentCol == lastCol) return 0; else if (rectangle[currentRow][currentCol + 1] == blockChar) return 0; else return 1; case south: if (currentRow == lastRow) return 0; else if (rectangle[currentRow + 1][currentCol] == blockChar) return 0; else return 1; case west: if (currentCol == 0) return 0; else if (rectangle[currentRow][currentCol - 1] == blockChar) return 0; else return 1; } return 1; } bool Grid::rightIsClear() const { switch (facing) { case west: if (currentRow == 0) return 0; else if (rectangle[currentRow - 1][currentCol] == blockChar) return 0; else return 1; case north: if (currentCol == lastCol) return 0; else if (rectangle[currentRow][currentCol + 1] == blockChar) return 0; else return 1; case east: if (currentRow == lastRow) return 0; else if (rectangle[currentRow + 1][currentCol] == blockChar) return 0; else return 1; case south: if (currentCol == 0) return 0; else if (rectangle[currentRow][currentCol - 1] == blockChar) return 0; else return 1; } return 1; } void Grid::display() const { int r, c; std::cout << "The Grid: " << std::endl; for (r = 0; r <= lastRow; r++) { for (c = 0; c <= lastCol; c++) std::cout << rectangle[r][c] << ' '; std::cout << std::endl; } } // -modifiers void Grid::turnLeft() { if (facing == north) facing = west; else if (facing == east) facing = north; else if (facing == south) facing = east; else if (facing == west) facing = south; setIcon(); rectangle[currentRow][currentCol] = icon; } void Grid::setIcon() { if (!(rectangle[currentRow][currentCol] == thingHereChar)) { switch (facing) { case north: icon = '^'; break; case east: icon = '>'; break; case south: icon = 'v'; break; case west: icon = '<'; break; } } } void Grid::move() { this->move(1); } // Precondition: spaces >= 1 void Grid::move(int spaces) { int oldRow(currentRow); int oldCol(currentCol); switch (facing) { case north: currentRow -= spaces; break; case east: currentCol += spaces; break; case south: currentRow += spaces; break; case west: currentCol -= spaces; break; } assert(currentRow >= 0); assert(currentCol >= 0); assert(currentRow <= lastRow); assert(currentCol <= lastCol); // Fix the starting intersection if (rectangle[oldRow][oldCol] == moverOnThingChar) rectangle[oldRow][oldCol] = thingHereChar; else if (rectangle[oldRow][oldCol] == icon && showPath) rectangle[oldRow][oldCol] = beenThereChar; else rectangle[oldRow][oldCol] = intersectionChar; int r, c; switch (facing) { case north: for (r = oldRow; r > currentRow; r--) { checkForBlock(r - 1, currentCol); if (rectangle[r][currentCol] != thingHereChar && showPath) rectangle[r][currentCol] = beenThereChar; } break; case east: for (c = oldCol; c < currentCol; c++) { checkForBlock(currentRow, c + 1); if (rectangle[currentRow][c] != thingHereChar && showPath) rectangle[currentRow][c] = beenThereChar; } break; case south: for (r = oldRow; r < currentRow; r++) { checkForBlock(r + 1, currentCol); if (rectangle[r][currentCol] != thingHereChar && showPath) rectangle[r][currentCol] = beenThereChar; } break; case west: for (c = oldCol; c > currentCol; c--) { checkForBlock(currentRow, c - 1); if (rectangle[currentRow][c] != thingHereChar && showPath) rectangle[currentRow][c] = beenThereChar; } } // end switch if (rectangle[currentRow][currentCol] == thingHereChar) rectangle[currentRow][currentCol] = moverOnThingChar; else rectangle[currentRow][currentCol] = icon; } void Grid::block(int blockRow, int blockCol) { assert(blockRow <= lastRow); assert(blockRow >= 0); assert(blockCol <= lastCol); assert(blockCol >= 0); // Can't block the place where the mover is assert(rectangle[blockRow][blockCol] != icon); // Can't block the place where the an thing has been placed assert(rectangle[blockRow][blockCol] != thingHereChar); // Now, if everything is alright, put the block down rectangle[blockRow][blockCol] = blockChar; } void Grid::putDown() { rectangle[currentRow][currentCol] = moverOnThingChar; } void Grid::putDown(int putDownRow, int putDownCol) { assert(putDownRow <= lastRow); assert(putDownRow >= 0); assert(putDownCol <= lastCol); assert(putDownCol >= 0); if (rectangle[putDownRow][putDownCol] == blockChar) { std::cout << std::endl << "Attempt to place block where the mover is at Grid(" << putDownRow << ", " << putDownCol << ")" << std::endl; std::cout << "...Program terminating..." << std::endl; } else rectangle[putDownRow][putDownCol] = thingHereChar; } void Grid::pickUp() { assert( rectangle[currentRow][currentCol] == thingHereChar || rectangle[currentRow][currentCol] == moverOnThingChar); // assert: Program terminated if there was nothing to pickup rectangle[currentRow][currentCol] = icon; } void Grid::toggleShowPath() { if (showPath) showPath = 0; else showPath = 1; } void Grid::checkForBlock(int r, int c) { if (rectangle[r][c] == blockChar) { std::cout << std::endl << "Attempt to move through the block at Grid(" << r << ", " << c << ")" << std::endl; if (facing == north) // must be moving north rectangle[r + 1][c] = icon; if (facing == east) // must be moving east rectangle[r][c - 1] = icon; if (facing == south) // must be moving south rectangle[r - 1][c] = icon; if (facing == west) // must be moving west rectangle[r][c + 1] = icon; for (r = 0; r < lastRow; r++) { for (c = 0; c < lastCol; c++) std::cout << rectangle[r][c] << ' '; std::cout << std::endl; } std::cout << "...Program terminating..." << std::endl; //I added this pause system("pause"); exit(0); } } The Grid: This is the output . . . . . . . . . . . . . . . . . . . . . > . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . The Grid: . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . < . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
Step by Step Solution
There are 3 Steps involved in it
Step: 1
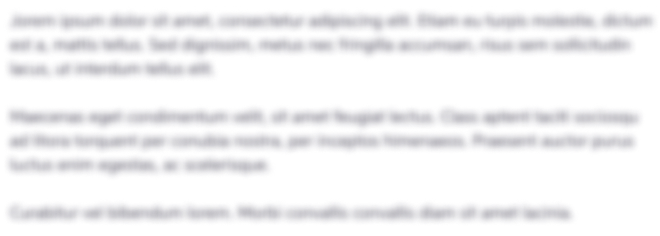
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started