Question
C++ programming, please. Adding comments in the code would be extremely appreciated Test1.cpp #include #include Text.h #include config.h //-------------------------------------------------------------------- // // Function prototype void copyTester
C++ programming, please. Adding comments in the code would be extremely appreciated
Test1.cpp
#include
#include "Text.h"
#include "config.h"
//--------------------------------------------------------------------
//
// Function prototype
void copyTester ( Text copyText ); // copyText is passed by value
void print_help ( );
//--------------------------------------------------------------------
int main()
{
Text a("a"), // Predefined test text objects
alp("alp"),
alpha("alpha"),
epsilon("epsilon"),
empty,
assignText, // Destination for assignment
inputText, // Input text object
inputText2;
int n; // Input subscript
char ch, // Character specified by subscript
selection; // Input test selection
// Get user test selection.
print_help();
// Execute the selected test.
cin >> selection;
cout
switch ( selection )
{
case '1' :
// Test 1 : Tests the constructors.
cout
cout
alpha.showStructure();
cout
epsilon.showStructure();
cout
a.showStructure();
cout
empty.showStructure();
break;
case '2' :
// Test 2 : Tests the length operation.
cout
cout
cout
cout
cout
break;
case '3' :
// Test 3 : Tests the subscript operation.
cout
cin >> n;
ch = alpha[n];
cout
if ( ch == '\0' )
cout
else
cout
break;
case '4' :
// Test 4 : Tests the assignment and clear operations.
cout
cout
assignText = alpha;
assignText.showStructure();
cout
assignText = a;
assignText.showStructure();
cout
assignText = empty;
assignText.showStructure();
cout
assignText = epsilon;
assignText.showStructure();
cout
assignText = assignText;
assignText.showStructure();
cout
assignText = alpha;
assignText.showStructure();
cout
assignText.clear();
assignText.showStructure();
cout
alpha.showStructure();
break;
case '5' :
// Test 5 : Tests the copy constructor and operator= operations.
cout
cout
alpha.showStructure();
copyTester(alpha);
cout
alpha.showStructure();
cout
a.showStructure();
a = epsilon;
cout
a.showStructure();
cout
epsilon.showStructure();
break;
#if LAB1_TEST1
case '6' : // In-lab Exercise 2
// Test 6 : Tests toUpper and toLower
cout
cin >> inputText;
cout
inputText.showStructure();
cout
inputText.toUpper().showStructure();
cout
inputText.toLower().showStructure();
break;
#endif // LAB1_TEST1
#if LAB1_TEST2
case '7' : // In-lab Exercise 3
// Test 7 : Tests the relational operations.
cout "
cout
cout
epsilon)
cout
alpha)
cout
alpha)
cout
alpha)
cout
alp)
cout
alpha)
cout
a)
cout
alpha)
cout
empty)
cout
empty)
break;
#endif // LAB1_TEST2
#if LAB2_TEST1
case '8' :
cout
cin >> inputText;
inputText.toReverse(inputText, 0);
//inputText.showStructure();
break;
#endif // LAB2_TEST1
#if LAB2_TEST2
case '9':
cout
cin >> inputText;
cout
cin >> inputText2;
inputText += inputText2;
inputText.toReverse(inputText, 0);
break;
#endif // LAB2_TEST2
default :
cout
}
system("PAUSE");
return 0;
}
//--------------------------------------------------------------------
void copyTester ( Text copyText )
// Dummy routine that is passed a text object using call by value. Outputs
// copyText and clears it.
{
cout
copyText.showStructure();
cout
copyText.clear();
copyText.showStructure();
}
//--------------------------------------------------------------------
void print_help()
{
cout
cout
cout
cout
cout
cout
cout
#if LAB1_TEST1
#else
#endif // LAB1_TEST1
cout
#if LAB1_TEST2
#else
#endif // LAB1_TEST2
cout
cout
cout
}
Text.cpp
#include
#include
#include
#include
#include "Text.h"
Text::Text ( const char *charSeq )
{
}
Text::Text ( const Text &other )
{
}
void Text::operator = ( const Text &other )
{
}
Text::~Text ()
{
}
int Text::getLength () const
{
return -1;
}
char Text::operator [] ( int n ) const
{
return 0;
}
void Text::clear ()
{
}
void Text::showStructure() const
// Outputs the characters in a string. This operation is intended for
// testing/debugging purposes only.
{
int j; // Loop counter
for (j = 0; j
cout
cout
for (j = 0; buffer[j] != '\0'; j++)
cout
cout
}
Text Text::toUpper( ) const
{
Text temp;
return temp;
}
Text Text::toLower( ) const
{
Text temp;
return temp;
}
bool Text::operator == ( const Text& other ) const
{
return false;
}
bool Text::operator
{
return false;
}
bool Text::operator > ( const Text& other ) const
{
return false;
}
// ********** Lab 2 *************
Text Text::operator += (const Text &input2)
{
// add your code here
return NULL;
}
char Text::toReverse(Text t1, int t) const
{
// add your code here
return ' ';
}
// *******************************
Textio.cpp
#include
#include
#include "Text.h"
//--------------------------------------------------------------------
istream & operator >> ( istream &input, Text &inputText )
// Text input function. Extracts a string from istream input and // returns it in inputText. Returns the state of the input stream.
{ const int textBufferSize = 256; // Large (but finite) char textBuffer [textBufferSize]; // text buffer
// Read a string into textBuffer, setw is used to prevent buffer // overflow.
input >> setw(textBufferSize) >> textBuffer;
// Apply the Text(char*) constructor to convert textBuffer to // a string. Assign the resulting string to inputText using the // assignment operator.
inputText = textBuffer;
// Return the state of the input stream.
return input; }
//--------------------------------------------------------------------
ostream & operator
// Text output function. Inserts outputText in ostream output. // Returns the state of the output stream.
{ output
Text.h
#ifndef TEXT_H #define TEXT_H
#include #include
using namespace std;
class Text { public:
// Constructors and operator= Text ( const char *charSeq = "" ); // Initialize using char* Text ( const Text &other ); // Copy constructor void operator = ( const Text &other ); // Assignment
// Destructor ~Text ();
// **** Lab 2 **** Text operator += (const Text &input2); char toReverse(Text t1, int t) const; // ***************
// Text operations int getLength () const; // # characters char operator [] ( int n ) const; // Subscript void clear (); // Clear string
// Output the string structure -- used in testing/debugging void showStructure () const;
//-------------------------------------------------------------------- // In-lab operations // toUpper/toLower operations (Programming Exercise 2) Text toUpper( ) const; // Create upper-case copy Text toLower( ) const; // Create lower-case copy
// Relational operations (Programming Exercise 3) bool operator == ( const Text& other ) const; bool operator ( const Text& other ) const;
private:
// Data members int bufferSize; // Size of the string buffer char *buffer; // Text buffer containing a null-terminated sequence of characters
// Friends
// Text input/output operations (In-lab Exercise 1) friend istream & operator >> ( istream& input, Text& inputText ); friend ostream & operator
};
#endif
Q4. (30 pts) On the foundation of the previous Text ADT homework, add the following methods to the Text class: 1. Implement the function toReverse) to reverse recursively the order of letters of the given Text object. Test your implementation by activating LAB2_TESTI. (15 pts) Function prototype: Text toReverse(Text obj, int position) const, Implement the operators +=. Test it by activating LAB-TEST2. (15 pts) Function prototype. Text operator += (const Text & input2) Notel: Source code with the test cases are provided (Lab2.rip). There were added he following two lines into the configuration file: #define LAB2TEST1 0 #define LAB2. TEST2 - // Programming operation + and recursion Plus, there are included test cases to test your implementationStep by Step Solution
There are 3 Steps involved in it
Step: 1
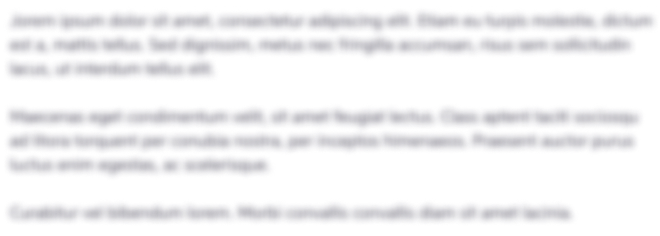
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started