Question
C++ Programming: Pointers Assignment Instructions Overview The objective of this assignment is to demonstrate the use of pointers in a program utilizing c-strings and tokenization.
C++ Programming: Pointers Assignment Instructions
Overview
The objective of this assignment is to demonstrate the use of pointers in a program utilizing c-strings and tokenization. The use of pointers is foundational in C++. This program provides an exercise in using pointers, passing them into functions, using them as return data types, and leveraging them in traversing arrays.
Instructions
Since many of you are studying the field of Cyber Security, you may find this assignment to be especially interesting as it uses a cipher that has been around for many years.
Your job is to write a program to encrypt blocks of text that can be sent over unsecured channels and decrypt blocks of text that have been received. The encryption and decryption will be accomplished using a Vigenere Cypher.
In this assignment, you will parse a string of words from a file into tokens using the strtok_s command with pointers and then use the Vigenere Cypher algorithm to encrypt and decrypt the parsed words.
If you are unfamiliar with the "Vigenere Cypher," an excellent description of it is provided in this short video:https://www.youtube.com/watch?v=SkJcmCaHqS0
Note: Don't panic as you watch the video. I included it only to provide you with a visual depiction of how this cipher works. In this assignment, you will be given the exact formulas to use to encrypt and decrypt the text.
Before performing any encryption/decryption activities, the first step is to read blocks of text from a file and parse the text into individual words.
The Program: Setting up classes and understanding tokenization
The first step in encrypting/decrypting blocks of text is to parse the text into words before applying the encryption/decryption algorithms to them. This parsing process is called "tokenization."
Tokenization:
The process of parsing sentences into words is called tokenization. To tokenize a sentence into words, use the C++ function strtok_s. Note: Do not try to use the C++ strtok function because it has been deemed unsafe and has therefore been deprecated.]
In your "client" code (i.e., the file that contains your main function), you will need to declare a character array that will hold 1000 characters.
Read the contents of a file into this array using the getline command. To view a discussion on how to use getline with a file object, see this link:https://stackoverflow.com/questions/13035674/how-to-read-line-by-line-or-a-whole-text-file-at-once
Note: I realize that it would be easier to read the file's contents into a string variable; that way, you wouldn't have to tokenize the block of text. HOWEVER... one of the objectives of this assignment is to illustrate the use of tokenization of c-strings using the strtok_s function and pointers. Therefore, you MUST use a character array to read the data from the file and tokenize it as described below to receive credit for this assignment.
Using the character array, your client should call the function strtok_s() to tokenize the character array into separate words. Here is an excellent discussion of tokenizing and the strtok_s function. Note that if you scroll down the web page to the "Example" section, you will see some code you can tweak your program.
https://msdn.microsoft.com/en-us/library/ftsafwz3.aspx
Class Construction: General Overview
There should be two classes in your program: Vigenere and MyText.
Vigenere contains the encryption key and the logic for encrypting and decrypting individual words.
MyText contains a vector of words that have been encrypted or decrypted and the logic for calling the functions in the Vigenere class to encrypt or decrypt a word. The MyText class serves as a middle-man between your client code and the Vigenere class and holds the encrypted/decrypted results in a vector.
Class Construction: Details
Vigenere Class
Data Member:
- key - string
Functions:
- Vigenere(string k) - one-argument constructor
- setKey(string k) - data member setter
- getKey() - data member getter
- toUpperCase(string k) - function that converts the string received to upper case and then returns that string
- encrypt(string w) - function (provided below) that encrypts the string received and then returns that string
- decrypt(string w) - function (provided below) that decrypts the string received and then returns that string
The Vigenere class should store an encryption key in a data member called "key."
The class should have a one-argument constructor that receives a string that represents the encryption key. The encryption key must be in all capital letters for the encryption and decryption algorithms to work. Therefore, before setting the encryption key's value, it should first be converted entirely to upper case. Do this in your toUpperCase function.
Note: Due to the way the Vigenere class is connected to the MyText class, you may find you have to go directly to the Vigenere class setKey() function to get the key into the class.
To convert a string to all upper case, loop over all the characters in the string and use the toupper function (http://www.cplusplus.com/reference/cctype/toupper/ ) to set each character in the string equal to the uppercase version of the character.
The purpose of this assignment is not to measure your ability to create encryption and decryption algorithms but rather to demonstrate your ability to use the strtok_s function, along with pointers, to tokenize character arrays and to leverage object-oriented programming in the design of your program. Therefore, you may use the following code for the encrypt and decrypt functions in your Vigenere class. Cite your source.
stringVigenere::encrypt(stringword)
{
string output;
for (int i = 0, j = 0; i char c =word[i]; if (c >='a' && c c +='A' -'a'; elseif (c 'Z') continue; output+= (c + key[j] - 2 *'A') % 26 +'A';//added 'A' to bring it in range of ASCII alphabet [ 65-90 | A-Z ] j = (j + 1) % key.length(); } return output; } stringVigenere::decrypt(stringword) { string output; for (int i = 0, j = 0; i char c =word[i]; if (c >='a' && c c +='A' -'a'; elseif (c 'Z') continue; output+= (c - key[j] + 26) % 26 +'A';//added 'A' to bring it in range of ASCII alphabet [ 65-90 | A-Z ] j = (j + 1) % key.length(); } return output; } [source:https://www.tutorialspoint.com/cplusplus-program-to-implement-the-vigenere-cypher ] MyText Class Data members: Functions: The MyText class contains a vector of strings that holds the words that were either encrypted or decrypted. The class should contain functions to encrypt a word, decrypt a word, create a file containing the vector of encrypted or decrypted words, and print the vector of encrypted or decrypted words. Logic of the program Main() In main(), call a function to create a menu that displays options to Encrypt a file, Decrypt a file, and Quit. The function should be called displayMenu and return an integer for the selected option. After each selection, the menu should be re-displayed until the user enters "3" for Quit. The menu should look like the one in the screenshot below:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
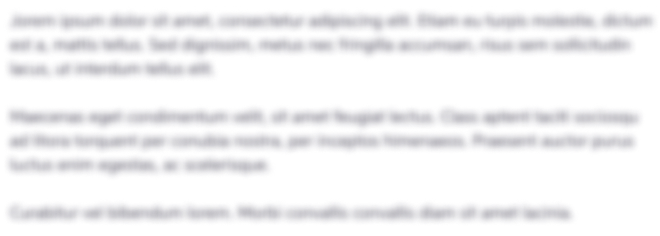
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started