Question
C++ Programming Quick Assignment: Please make sure ALL parts are done and the code compiles and runs for a thumbs up :-) config.h: /** *
C++ Programming Quick Assignment: Please make sure ALL parts are done and the code compiles and runs for a thumbs up :-)
config.h:
/**
* List class (Lab 3/Lab 4) configuration file.
* Activate test #N by defining the corresponding LAB3_TESTN to have the value 1.
*
* Because you will copy the List class code to your ordered list directory, having
* two "config.h" files presented the risk of accidentally replacing the one in the
* ordered list directory. So the two configuration files are combined for labs 3 and 4.
*
* NOTE!!! There was an error in the printed book. TEST1 shows up twice in the book.
* The basic List implementation uses TEST1 as described below, then exercise 2
* is activated by TEST2
*/
#define LAB3_TEST1 0 // 0 => test with char, 1 => test with int
#define LAB3_TEST2 0 // Prog exercise 2: moveToNth
#define LAB3_TEST3 0 // Prog exercise 3: find
/**
* Ordered list class tests.
*/
#define LAB4_TEST1 0 // merge: programming exercise 2
#define LAB4_TEST2 0 // subset: programming exercise 3
ListArray.h:
//--------------------------------------------------------------------
//
// Laboratory 3 ListArray.h
// **Instructor's Solution**
// Class declaration for the array implementation of the List ADT
//
//--------------------------------------------------------------------
#ifndef LISTARRAY_H
#define LISTARRAY_H
#include
#include
using namespace std;
#pragma warning( disable : 4290 )
template
class List
{
public:
static const int MAX_LIST_SIZE = 10; // Default maximum list size
// Constructors
List ( int maxNumber = MAX_LIST_SIZE ); // Default constructor
List ( const List& source ); // Copy constructor
// Overloaded assignment operator
List& operator= ( const List& source );
// Destructor
virtual ~List ();
// List manipulation operations
virtual void insert ( const DataType& newDataItem ) // Insert after cursor
throw ( logic_error );
void remove () throw ( logic_error ); // Remove data item
virtual void replace ( const DataType& newDataItem ) // Replace data item
throw ( logic_error );
void clear (); // Clear list
// List status operations
bool isEmpty () const; // List is empty
bool isFull () const; // List is full
// List iteration operations
void gotoBeginning () // Go to beginning
throw ( logic_error );
void gotoEnd () // Go to end
throw ( logic_error );
bool gotoNext () // Go to next data item
throw ( logic_error );
bool gotoPrior () // Go to prior data item
throw ( logic_error );
DataType getCursor () const
throw ( logic_error ); // Return data item
// Output the list structure -- used in testing/debugging
virtual void showStructure () const;
// In-lab operations
void moveToNth ( int n ) // Move data item to pos. n
throw ( logic_error );
bool find ( const DataType& searchDataItem ) // Find data item
throw ( logic_error );
protected:
// Data members
int maxSize,
size, // Actual number of data item in the list
cursor; // Cursor array index
DataType *dataItems; // Array containing the list data item
};
#endif
ListArray.cpp:
#include "ListArray.h"
template
List
{
}
template
List
{
}
template
List
{
return *this;
}
template
List
{
}
template
void List
throw ( logic_error )
{
}
template
void List
{
}
template
void List
throw ( logic_error )
{
}
template
void List
{
}
template
bool List
{
return false;
}
template
bool List
{
return false;
}
template
void List
throw ( logic_error )
{
}
template
void List
throw ( logic_error )
{
}
template
bool List
throw ( logic_error )
{
return false;
}
template
bool List
throw ( logic_error )
{
return false;
}
template
DataType List
throw ( logic_error )
{
DataType t;
return t;
}
#include "show3.cpp"
template
void List
throw ( logic_error )
{
}
template
bool List
throw ( logic_error )
{
return false;
}
show3.cpp:
//--------------------------------------------------------------------
//
// Laboratory 3 show3.cpp
//
// Array implementation of the showStructure operation for the
// List ADT
//
//--------------------------------------------------------------------
#include "ListArray.h"
template
void List
// outputs the data items in a list. if the list is empty, outputs
// "empty list". this operation is intended for testing/debugging
// purposes only.
{
int j; // loop counter
if ( size == 0 )
cout
// The Ordered List code blows up below. Since this is just debugging
// code, we check for whether the OrderedList is defined, and if so,
// print out the key value. If not, we try printing out the entire item.
// Note: This assumes that you have used the double-inclusion protection
// in your OrderedList.cpp file by doing a "#ifndef ORDEREDLIST_CPP", etc.
// If not, you will need to comment out the code in the section under
// the "else", otherwise the compiler will go crazy in lab 4.
// The alternative is to overload operator
// the ordered list.
else
{
cout
for ( j = 0 ; j
cout
cout
for ( j = 0 ; j
if( j == cursor ) {
cout
cout
#ifdef ORDEREDLIST_CPP
.getKey()
#endif
;
cout
cout
}
else
cout
#ifdef ORDEREDLIST_CPP
.getKey()
#endif
}
cout
}
}
test3dna.cpp:
//--------------------------------------------------------------------
//
// Laboratory 3, In-lab Exercise 1 test3dna.cpp
//
// Test program for the countbases function
//
//--------------------------------------------------------------------
// Reads a DNA sequence from the keyboard, calls function countBases
// countBases (which uses a list to represent a DNA sequence), and
// outputs the number of times that each base (A, G, C and T) occurs
// in the sequence.
#include
#include "ListArray.cpp"
using namespace std;
//--------------------------------------------------------------------
//
// Function prototype
//
void countBases ( List
int &aCount,
int &cCount,
int &tCount,
int &gCount );
//--------------------------------------------------------------------
int main ()
{
List
char base; // DNA base
int aCount, // Number of A's in the sequence
cCount, // Number of C's in the sequence
tCount, // Number of T's in the sequence
gCount; // Number of G's in the sequence
// Read the DNA sequence from the keyboard.
cout
cin.get(base);
while ( base != ' ' )
{
dnaSequence.insert(base);
cin.get(base);
}
// Display the sequence.
cout
if( dnaSequence.isEmpty() )
cout
else
{
dnaSequence.gotoBeginning();
do
{
cout
} while ( dnaSequence.gotoNext() );
cout
}
// Count the number of times that each base occurs.
countBases(dnaSequence,aCount,cCount,tCount,gCount);
// Output the totals.
cout
cout
cout
cout
}
//--------------------------------------------------------------------
//
// Insert your countBases function below.
//
Programming Exercise 1 The genetic information encoded in a strand of deoxyribonucleic acid (DNA) is stored in the purine and pyrimidine bases (adenine, guanine, cytosine, and thymine) that form the strand. Biologists are keenly interested in the bases in a DNA sequence because these bases determine what the sequence does. By convention DNA sequences are represented by using lists containing the letters "A" "G", "C", and "T" or adenine, guanine cytosine, and thymine, respectively). The following function computes one property of a DNA sequence-the number of times each base occurs in the sequence
Step by Step Solution
There are 3 Steps involved in it
Step: 1
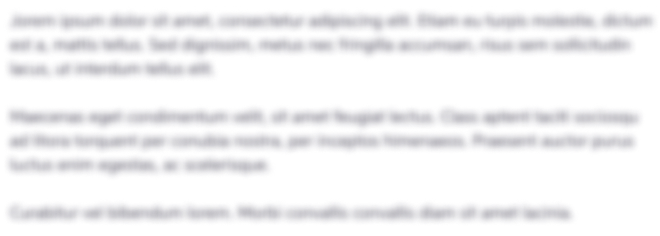
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started