Question
C++ Programming // You will need to implement a program that converts a decimal number to hexadecimal using the ADT stack with a singly linked
C++ Programming //
You will need to implement a program that converts a decimal number to hexadecimal using the ADT stack with a singly linked list.
Your program will receive a decimal number from the user and display its equivalent hexadecimal number.
Use "Stack using a Singly Link List" program posted on D2L, (create the template Stack class), and make necessary changes to solve the problem. Your code must include exception handling.
================================
dNode.h
================================
#ifndef DNODE_H #define DNODE_H
class dNode { private: double data; dNode* next; public: dNode(double); virtual ~dNode(); //for later use of polymorphism, review the topic again
friend class dStack; // allows dStack for private member access };
#endif
================================
dNode.cpp
================================
#include "dNode.h"
// constructor: create a new Node with d as data dNode::dNode(double data) { this->data = data; next = 0; }
dNode::~dNode() { }
================================
dStack.h
================================
#ifndef DSTACK_H #define DSTACK_H
#include #include #include "dNode.h" using namespace std;
class dStack { private: dNode* topNode; public:
class StackEmptyException { };
dStack(); dStack(const dStack& copy); dStack& operator=(const dStack&); virtual ~dStack(); void push(double); void pop(); double top(); bool isEmpty(); bool isFull(); };
#endif
================================
dStack.cpp
================================
#include //for exit() #include "dStack.h"
using namespace std;
// constructor: new stack is created. topNode is null. dStack::dStack() { topNode = 0; }
//copy constructor dStack::dStack(const dStack& copy) {
dNode* tempNode = copy.topNode; if(tempNode == NULL) { //same as !tempNode because NULL is 0 in C++ topNode = NULL; } else { dNode* newNode = topNode = new dNode(tempNode->data); while(tempNode->next) { tempNode = tempNode->next; newNode->next = new dNode(tempNode->data); newNode = newNode->next; } } }
dStack& dStack::operator =(const dStack &original) { dStack localStack(original);
dNode* tempNode = this->topNode; this->topNode = localStack.topNode; localStack.topNode = tempNode;
return *this;
}
// destructor: free all the memory allocated for the stack dStack::~dStack() { while (!isEmpty()) pop(); }
// push a data onto the stack void dStack::push(double data) { try { dNode* newNode = new dNode(data); newNode->next = topNode; topNode = newNode; } catch (bad_alloc &e) { //e.what(): returns a char sequence for exception details cout << "memory allocation exception: " << e.what() << endl; exit(1); } }
// pop the data from the stack void dStack::pop() { if (isEmpty()) throw StackEmptyException();
dNode* discard = topNode; topNode = topNode->next; delete discard; }
// read the data on the top of the stack double dStack::top() { if (isEmpty()) throw StackEmptyException();
return topNode->data;
}
// is stack empty? bool dStack::isEmpty() { return (topNode == 0); }
// is stack full? bool dStack::isFull() { return false; // never, unless memory is full }
================================
main.cpp
================================ #include #include "dStack.h" using namespace std;
void stack_test();
int main() {
stack_test();
return 0; }
void stack_test() { dStack s1;
// Stack empty test if (s1.isEmpty()) { cout << "s1 is empty at the beginning." << endl; } else { cout << "s1 must be empty. Something's wrong!" << endl; }
for(int i = 0; i < 5; i++) s1.push(1.1 * (i + 1));
dStack s2(s1); cout << "S2: Expected: 5.5 4.4 3.3 2.2 1.1 -->" << endl; for (int i = 0; i < 5; i++) { cout << s2.top() << endl; s2.pop(); }
// pop() test: reverses the items cout << "S1: Expected: 5.5 4.4 3.3 2.2 1.1 -->" << endl; for (int i = 0; i < 5; i++) { cout << s1.top() << endl; s1.pop(); }
// Stack empty test if (s1.isEmpty()) { cout << "s1 is empty after five pop() calls." << endl; } else { cout << "s1 must be full. Something's wrong!" << endl; }
// StackEmptyException test try { cout << "One more pop when the stack is empty..." << endl; s1.pop(); } catch (dStack::StackEmptyException) { cout << "Exception: cannot pop, stack is empty" << endl; }
dStack s3; s3 = s1; for(int i = 0; i < 5; i++) s3.push(1.1 * (i + 1));
}
include exception handling
Step by Step Solution
There are 3 Steps involved in it
Step: 1
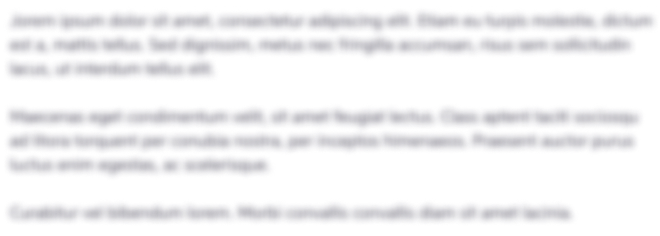
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started