Question
C++ Project Modify the Date Class: Standards Your program must start with comments giving your name and the name of the assignment. Your program must
C++ Project Modify the Date Class:
Standards
Your program must start with comments giving your name and the name of the assignment.
Your program must use good variable names.
All input must have a good prompt so that the user knows what to enter.
All output must clearly describe what is output.
Using the date class, make the following modifications:
Make the thanksgiving function you wrote for project 1 into a method of the Date class. It receive the current year and returns the date for Thanksgiving.
In the Date class there are only a few overloaded operators for comparison. Add all of the following: <=, >=, and !=
Add a method to return the season as an integer: 0=winter, 1=spring, 2=summer and 3=fall for the northern hemisphere.
Your main program should illustrate the use of each method.
You may use March 20th for the first day of Spring. Use June 20th for the first day of summer, September 22 for the first day of fall, and December 21 as the first day of winter. You will find the overloaded operators handy for this. A nice solution for this is to use an array to store the first date for each season, then loop to see where a date falls.
Main.cpp:
#include "Date.h"
#include
using namespace std;
// Start of main
void bubbleSort(Date date[], int n)//is an array of dates and is the size of the array
{
int i, j;
for (i = 0; i < n - 1; i++)
// Last i elements are already in place
for (j = 0; j < n - i - 1; j++)
if (date[j] > date[j + 1])
{
Date temp = date[j];
date[j] = date[j + 1];
date[j + 1] = temp;
}
}
int main() {
// Declare arrays and variables
const int SIZE = 7; // Size of array elements
const int COUNT = 6; // Number of holidays
Date weekDays[SIZE]; // Weekday array
Date holidays[COUNT]; // Holiday array
string holidaysDescription[COUNT];
int year = 0;
Date easterSunday(04, 01, 2018); // Easter Sunday
holidays[0] = easterSunday;
holidaysDescription[0] = "Easter Sunday";
Date mothersDay(05, 13, 2018); // Mother's Day
holidays[1] = mothersDay;
holidaysDescription[1] = "Mother's Day";
Date memorialDay(05, 28, 2018); // Memorial Day
holidays[2] = memorialDay;
holidaysDescription[2] = "Memorial Day";
Date fathersDay(06, 17, 2018); // Fathers Day
holidays[3] = fathersDay;
holidaysDescription[3] = "Father's Day";
Date independenceDay(07, 04, 2018); // Independence Day
holidays[4] = independenceDay;
holidaysDescription[4] = "Independence Day";
// Prompt the user for current year
cout << "Please enter the current year: ";
cin >> year;// Read in year
cout << " ";
// Search for the date of Thanksgiving and add it to
// the holiday array
Date giveThanks = giveThanks.thanksGiving(year);
holidays[5] = giveThanks;
holidaysDescription[5] = "Thanksgiving";
bubbleSort(holidays, COUNT);//Sort the list of the array
cout << "The holidays are sorted by date: ";
for (int index = 0; index < COUNT; index++)
{
// Display the holiday
int day = holidays[index].weekday();
cout << holidaysDescription[index] << " is on " << holidays[index] << " which falls on a ";
// Display the day
switch (day) {
case 0: cout << "Sunday" << endl; break;
case 1: cout << "Monday" << endl; break;
case 2: cout << "Tuesday" << endl; break;
case 3: cout << "Wednesday" << endl; break;
case 4: cout << "Thursday" << endl; break;
case 5: cout << "Friday" << endl; break;
case 6: cout << "Saturday" << endl; break;
}
}
system("pause");
return 0;
}
Date.cpp:
#include "Date.h"
Date::Date() { time_t t = time(0); // get time now struct tm * now = localtime(&t); month = now->tm_mon + 1; day = now->tm_mday; year = now->tm_year + 1900; setDays(); }//constructor for today: cross platform /*Date::Date() { struct tm newtime; __time64_t long_time; char timebuf[26]; errno_t err; // Get time as 64-bit integer. _time64( &long_time ); // Convert to local time. err = _localtime64_s( &newtime, &long_time ); month=newtime.tm_mon+1; day=newtime.tm_mday; year=newtime.tm_year+1900; setDays(); }//constructor for today for Visual Studio */ bool validDate(int m, int d, int y) { int days2[] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 }; bool valid=true; //assume it is valid until found to be invalid if(y<1000) valid=false; if(m<1 || m>12) valid=false; if (valid) { if (leapYear(y)) days2[2] = 29; if (d<1 || d>days2[m]) valid = false; } return valid; }//validDate void Date::setDays(void) { int days2[] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 }; for (int m = 0; m < 13; m++) days[m] = days2[m]; if (leapYear()) days[2] = 29; } Date::Date(int m, int d, int y) { //constructor to assign values to month day and year if(validDate(m,d,y)) { month=m; day=d; year=y; } else { month=day=1; year=1970; //Unix time starting point } //not valid: set to default valid date for(int m=0;m<13;m++) days[m]=days2[m]; } //constructor with assigned values Date::Date(int julian) { //Fliegel-Van Flandern algorithm to convert Julian date to Gregorian number month, day, and year gregorian(julian,month,day,year); setDays(); }//Date Julian Date::Date (string str) { //constructor for todays date as "mm/dd/year //Parse str by adding one char at a s time to the token until a "/" is encounter. //When "/" is encountered start the next token //int p=0; int count=0; int num[3]; string token[3]; int len=str.length(); for(int p=0; p(const Date& otherDate) { //Convert both dates to Julian and compare the Julian dates int jd1=julian(); int jd2=otherDate.julian(); return jd1>jd2; }//operator> ostream& operator << (ostream &output, const Date &d) { output << d.toString(); return output; } // operator << istream& operator >> (istream &input, Date &d) { string s; input >> s; Date other(s); //create a new Date d=other; //assign the new Date to d return input; } // operator >>
Date.h
#ifndef Date_H
#define Date_H #include#include #include #include using namespace std; class Date { private: int month, day, year; int days[13]; void setDays(); public: //constructors Date(); //constructor for todays date Date(int m, int d, int y); //constructor to assign date Date(string str); //constructor for todays date as "mm/dd/year Date(int gregorian); //constructor to convert a Gregorian date to Date //methods int getMonth() const; //returns the private variable month int getDay() const; //returns the private variable day int getYear() const; //returns the private variable year string toString() const; //returns the string mm/dd/yyyy bool leapYear() const; //determines if the year is a leap year int dayofYear() const; //returns the day of the year: ie 2/1/???? is the 32 day of year int julian() const; int weekday() const; //returns 0 for Sunday, 1 for Monday, etc. //overloaded operators bool operator==(const Date& otherDate); //2 dates are equal if month, day and year are equal bool operator<(const Date& otherDate); //a date is < another date if it is earlier bool operator>(const Date& otherDate); //a date is > another date if it is later Date operator=(const Date& otherDate); //let's you copy one date to another. Date operator+(int); //Assign new values to the date after adding the number of days friend ostream& operator << (ostream &output, const Date &d); friend istream& operator >> (istream &input, Date &d); }; bool validDate(int m, int d, int y); //test other date bool leapYear(int y); //let's you test any year, not just the year for the instance int julian(int m, int d, int y); //convert any date to Julian void gregorian(int jd, int &mth, int &d, int &y); static int days2[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
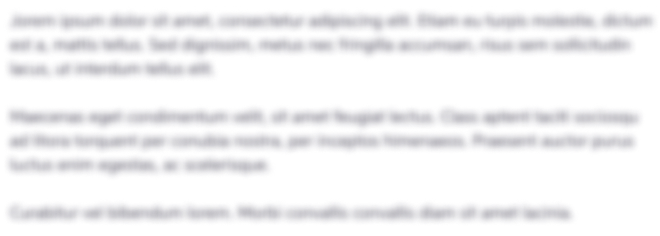
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started