Question
C# question #region Question 1 - 13 marks /* * Write a method that does not take any argument nor does it * return a
C# question
#region Question 1 - 13 marks
/*
* Write a method that does not take any argument nor does it
* return a value. The method only displays the following text.
*
* YOU MUST INSERT YOUR NAME IN THE TOP LINE OF THE MENU
*
* +---------------------COMP100------------------------+
* |a) Working with Newton's Law|
* |b) Time Converter|
* |c) Square and Cube Table|
* |d) Number Frequency|
* ||
* |x) To exit the program|
* +----------------------------------------------------+
* Press the letter corresponding to your choice->
*
*/
#endregion
#region Question 2 - 26 marks
/*
* In your Main() method, using a suitable looping structure, write the
* code to call this method repeatedly.
* Using a suitable branching structure, write the code to implement the
* following required functionality for all valid responses.
* Valid responses includes both upper and lower case of the input. The
* following must be implemented:
* A will call the DemoQuestion3() method
* B will call the DemoQuestion4() method
* C will call the DemoQuestion5() method
* D will call the DemoQuestion6() method
* X will terminate the program
* Any other key will produce an error message
*
* YOU DON'T HAVE TO CREATE A NEW METHOD FOR THIS QUESTION
*/
#endregion
#region Question 3 - 15 marks
/*CalculateForce - 9 marks
*"Newton's second law of Motion F=ma."
*
* Write a method with the following specifications:
* Name: CalculateForce
* Parameter:
*A double representing the mass of the first body
*A double representing Acceleration of the body
*
* Returns: a double representing the force
* Displays : Nothing
* Task: Calculate and return the force .
*[F= m*a].
*/
/* Driver for question 3 - 6 marks
*
* Write the statements to call the above method with the masses of the earth,
* moon and the distance between them and display the resulting force.
*
*Mass of Object = 56.78, Acceleration= 123.67.
*
*You shoul use right format specifier in your output,keep only two decimal values
*/
static void DemoQuestion3()
{
Console.WriteLine(" **********Begin Question 3 **********");
//code for invoking question 3 goes here
Console.WriteLine(" **********End Question 3 ********** ");
}
#endregion
#region Question 4 - 10 marks
/* Write a method with the following specifications: - 9 marks
* Name: TimeConversion
* Parameter: an int representing the time value in minute
* Returns: Nothing
* Displays : the hour andminute equivalent
* Tasks: Converts the argument to hours and minutes and display
* it on the console.
*/
/* Driver for question 4 - 3 marks
* Write the code to call the above method below three times
* with argument 75, 100 and 290.
*/
static void DemoQuestion4()
{
Console.WriteLine(" **********Begin Question 4 **********");
//code for invoking question 4here
Console.WriteLine(" **********End Question 4 ********** ");
}
#endregion
#region Question 5 - 18 marks
/* SquareCube - 10 marks
* Write a method with the following specifications:
* Name: SquareCube
* Parameter:
*A int that represents a number
*A int that represents the square of the first argument
*A int that represents the cube of the first argument
* Returns: Nothing
* Displays : Nothing
* Task: Calculates the square and cube of the first argument and
* assigns the result values to the second and third Parameter
*
* NOTE: the second and thred arguemnts are decorated so that the
* method is able to change the actual value of the variable
*/
/* Driver for question 5 - 8 marks
* Write the code statements(loop) to call this method ten times with values
* 2, 3, 4 ... 11and printout the values for number,
* square and cube in a tabular format. It is expected to use some
* kind of repetitive structure
*/
static void DemoQuestion5()
{
Console.WriteLine(" **********Begin Question 5 **********");
//code for invoking question 5 goes here
Console.WriteLine(" **********End Question 5 ********** ");
}
#endregion
#region Question 6 - 20 marks
/* Write a method with the following specifications: //13 marks
* Name: CalculateNumberFrequencies
* Argument: an int array with values ranging from 0 to 999
* Returns: int array of 20 integers. The first element will
*indicate the number of values which are multiple of 2, the second element will indicate
*the number of values which are multiple of 3, the
*third element will indicate the number of values which are multiple of 5.
* Display: Nothing
* Tasks: The method will create an int array of 20 elements
*(call this the result).
*Each item of the argument is examined and the
*appropriate element of the result array is incremented.
*/
/* Driver for Question 6 - 7 marks
* Write the code to do the following:
*Call the GenerateRandomIntArray() and assign the results to a suitable variable
*Use the DisplayIntArray() method to display the above variable
*Print an empty line
*Call CalculateNumberFrequencies() method and display the resulting value
*/
static void DemoQuestion6()
{
Console.WriteLine(" **********End Question 6 **********");
//code for invoking question 6 goes here
Console.WriteLine(" **********End Question 6 ********** ");
}
/*
* FREE CODE
*/
static void DisplayIntArray(int[] numbers)
{
foreach (var x in numbers)
Console.Write("{0,3}", x);
}
/*
* FREE CODE
*/
static int[] GenerateRandomIntArray(int numberOfItems, int largestValue)
{
int[] result = new int[numberOfItems];
Random generator = new Random();
for (int i = 0; i < numberOfItems; i++)
result[i] = generator.Next(largestValue);
return result;
}
#endregion
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
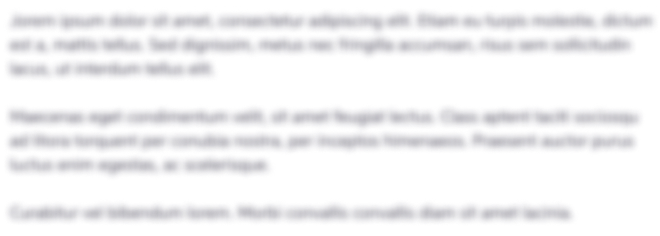
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started