Question
C++ Read in employee information, calculate pay, and output to screen. We just learned structures and I'm confused how to read it in with file
C++ Read in employee information, calculate pay, and output to screen. We just learned structures and I'm confused how to read it in with file IO. I've set it up to where it reads in the name but breaks when it tries to read in the next information (payrate, hours, employee type). I know I need to use strcpy for name but the other ones I figured I could read in using the structure alone. I also need help declaring my variables in main since it gives me errors when I try to call the empReport functions which outputs everything. What can I fix or how would you go about this? I appreciate any help, thanks. I have it split into a few files:
employee.cpp
#pragma once #define _CRT_SECURE_NO_WARNINGS #include "employee.h" #include #include #include
using std::cout; using std::endl; using std::setw; using std::ifstream;
const char* WORKER_TYPE[] = { "Full-time", "Part-time" }; const char EMP_DATA[] = "employee.dat"; const char REPORT[] = "pay.txt";
void readEmpData(EmpData* employee[], int entries) { char buffer[100]; char* temp_type; float temp_payrate; float temp_hours; char* temp_name = nullptr; entries = 0;
ifstream input(EMP_DATA);
input >> buffer; if (input.is_open()) { while (!input.eof()) { const int len = strlen(buffer) + 1; // allocate space of name + null temp_name = new char[len]; // employee_name is equal to new space strcpy_s(temp_name, len, buffer); //employee[entries]->name = temp_name;
input >> employee[entries]->payrate; input >> employee[entries]->hours; input >> employee[entries]->employee_type;
//if (temp_type == 'F') //{ // employee[entries]->employee_type = F; //} //else // employee[entries]->employee_type = P;
entries++; input >> buffer; } } }
EmpData* createEmp(const char* name, float payrate, float hours, EmpType employee_type) { const int len = strlen(name) + 1; // allocate space of name + null char* employee_name = new char[len]; // employee_name is equal to new space strcpy_s(employee_name, len, name); // copy name to employee_name with correct length
//return new EmpData(employee_name, payrate, hours, employee_type, pay, deductions); EmpData* employee = new EmpData; employee->payrate = payrate; employee->hours = hours; employee->employee_type = employee_type; return employee; }
void freeEmp(EmpData* employee) { delete[] employee->name; delete employee; } void empReport(const EmpData* employee[100], int entries) { cout name payrate hours employee_type] pay deductions
employee.h
#pragma once
enum EmpType { F, P };
struct EmpData { char* name; float payrate; float hours; EmpType employee_type; double pay; int deductions; };
void readEmpData(EmpData* employee[], int entries); void empReport(const EmpData* employee[100], int entries); EmpData* createEmp(const char* name, float payrate, float hours, EmpType employee_type);
main.cpp
#include #include "employee.h";
using std::endl; using std::cin; using std::cout; using std::ifstream; using std::ofstream;
const char EMP_DATA[] = "employee.dat"; const char REPORT[] = "pay.txt";
int main() { int entries = 0; EmpData* employee[100]; char* name; float payrate; float hours; EmpType employee_type;
readEmpData(employee, entries); //empReport(employee, entries); return 0; }
SPECS:
- main.cpp - contains only the main function
- Main will mostly call other functions to do the work
- It should be easy to see, from a high level, what the program does
- employee.h - a header file with the declaration of your data types and functions
- employee.cpp - contains the implementation of your functions
The functions you must include
- a function to create a new instance of your data structure
- NOTE: This function does not read the data from the input file, pass it in as parameters
- a function to calculate (process) the data
- a function to print your report
- a function to cleanup any memory allocated in your create function
- any other functions you think you should add
In short, this program will read the input data of:
- a first name
- The buffer to read the name should be at least 100 characters
- The struct MUST use new to allocate memory to store the name in
- a decimal number for their rate of pay
- a decimal number for the number of hours worked
- a letter representing the type of employee they are
- F is full time
- P is part time
- The structure MUST use an enum to store this information
The calculation done is to calculate how much pay is needed for the given person:
- Pay rate times the number of work hours
- If the person is full time
- Deduct $5.00 for Union dues
Data Types
- An enum to represent the employee type (convert to and from the F and P of the input file)
- A struct to hold all of the data of one employee including calculated values
- An array of pointers to the struct
- The array MUST hold at least 100 of these pointers
- The struct MUST be allocated with new
Step by Step Solution
There are 3 Steps involved in it
Step: 1
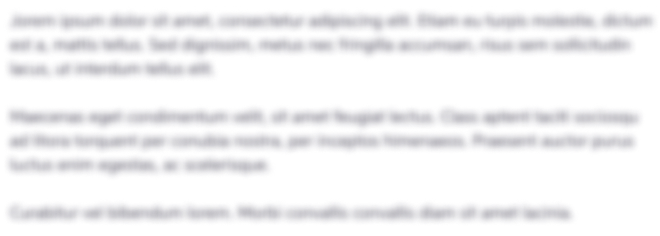
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started