Answered step by step
Verified Expert Solution
Question
1 Approved Answer
( C + + ) Refactor the Binary class to add one or more proxies to generate the desired output. Overload a COMBO combination of
CRefactor the Binary class to add one or more proxies to generate the desired output. Overload a COMBO combination of AND XOR to change its behavior. o Instead of doing the binary operations do a three element addition. o a & b c a b c Do not ADD any files to the project No free functions do all overloading inside classes as methods No modern C o No Lambdas, Autos, templates, etc... o No Boost NO Streams o Used fopen, fread, fwrite... No code in MACROS o Code needs to be in cpp files to see and debug it easy Exception: o implicit problem needs templates Binary.h cpp #ifndef BINARYH #define BINARYH struct Binary Binary; Binaryunsigned int x; Binaryconst Binary &r; ~Binary; Binary operator const Binary &r; struct XOR; struct AND; struct COMBO; XOR operator const Binary& r const; AND operator & const Binary& r const; COMBO operator & const XOR& r const; TODO : Need to Fix. operator unsigned int const; Data: do not add or modify the data unsigned int x; ; struct Binary::XOR XORconst Binary& a const Binary& b; operator Binary const; operator unsigned int const; const Binary& a; const Binary& b; ; struct Binary::AND ANDconst Binary& a const Binary& b; operator Binary const; operator unsigned int const; const Binary& a; const Binary& b; ; struct Binary::COMBO COMBOconst Binary& a const XOR& b; operator Binary const; operator unsigned int const; const Binary& a; const XOR& b; ; #endif End of File Binary.cpp cpp #include "Binary.h Binary::Binary DO NOT MODIFY thisx ; Binary::Binaryunsigned int x DO NOT MODIFY thisx x; Binary::Binaryconst Binary& r DO NOT MODIFY thisx rx; Binary::~Binary DO NOT MODIFY Binary Binary::operator const Binary& r DO NOT MODIFY thisx rx; return this; Binary::XOR Binary::operator const Binary& r const return XORthis r; Binary::AND Binary::operator & const Binary& r const return ANDthis r; Binary::COMBO Binary::operator & const XOR& r const TODO : Need to fix return COMBOthis r; Binary::operator unsigned int const return thisx; Binary::XOR::XORconst Binary& a const Binary& b : aa bb Binary::XOR::operator Binary const return Binaryax bx; Binary::XOR::operator unsigned int const return ax bx; Binary::AND::ANDconst Binary& a const Binary& b : aa bb Binary::AND::operator Binary const return Binaryax & bx; Binary::AND::operator unsigned int const return ax & bx; Binary::COMBO::COMBOconst Binary& a const XOR& b : aa bb Binary::COMBO::operator Binary const return Binaryax bax bbx; Binary::COMBO::operator unsigned int const return ax bax bbx; End of File Sample Test Code : Combo Binary ax; Binary ax; Binary ax; Binary aBinary; unsigned int aValue; Binary tBinary; unsigned int tValue; Binary ttBinary; unsigned int ttValue; Trace::outaxx ax; Trace::outaxx ax; Trace::outaxx ax; Trace::out ; aBinary a & a a; aValue a & a a; Trace::outaBinary a & a axx aBinary.x; Trace::outaValue a & a axx aValue; Trace::out ; Sample Test Output : ax ax ax aBinary a & a ax aValue a & a ax However, the problem currently occurring in the above code output : ax ax ax aBinary a & a ax aValue a & a ax The test fails, x is an invalid output. If I calculate a & aand a a separately a & ax a ax it seems like aa should be calculated first to get the result of x but I don't know how. Please modify the COMBO part so that I get the same result as the sample output, a & a ax NEVER NEVER NEVER Chenge test code to like a & a a Please fix Binary.h and Binary.cpp Chegg give wrong answer. I didn't ask about Java project in NetBeans
CRefactor the Binary class to add one or more proxies to generate the desired output.
Overload a COMBO combination of AND XOR to change its behavior.
o Instead of doing the binary operations do a three element addition.
o a & b c a b c
Do not ADD any files to the project
No free functions do all overloading inside classes as methods
No modern C
o No Lambdas, Autos, templates, etc...
o No Boost
NO Streams
o Used fopen, fread, fwrite...
No code in MACROS
o Code needs to be in cpp files to see and debug it easy
Exception:
o implicit problem needs templates
Binary.h
cpp
#ifndef BINARYH
#define BINARYH
struct Binary
Binary;
Binaryunsigned int x;
Binaryconst Binary &r;
~Binary;
Binary operator const Binary &r;
struct XOR;
struct AND;
struct COMBO;
XOR operator const Binary& r const;
AND operator & const Binary& r const;
COMBO operator & const XOR& r const; TODO : Need to Fix.
operator unsigned int const;
Data: do not add or modify the data
unsigned int x;
;
struct Binary::XOR
XORconst Binary& a const Binary& b;
operator Binary const;
operator unsigned int const;
const Binary& a;
const Binary& b;
;
struct Binary::AND
ANDconst Binary& a const Binary& b;
operator Binary const;
operator unsigned int const;
const Binary& a;
const Binary& b;
;
struct Binary::COMBO
COMBOconst Binary& a const XOR& b;
operator Binary const;
operator unsigned int const;
const Binary& a;
const XOR& b;
;
#endif
End of File
Binary.cpp
cpp
#include "Binary.h
Binary::Binary DO NOT MODIFY
thisx ;
Binary::Binaryunsigned int x DO NOT MODIFY
thisx x;
Binary::Binaryconst Binary& r DO NOT MODIFY
thisx rx;
Binary::~Binary DO NOT MODIFY
Binary Binary::operator const Binary& r DO NOT MODIFY
thisx rx;
return this;
Binary::XOR Binary::operator const Binary& r const
return XORthis r;
Binary::AND Binary::operator & const Binary& r const
return ANDthis r;
Binary::COMBO Binary::operator & const XOR& r const TODO : Need to fix
return COMBOthis r;
Binary::operator unsigned int const
return thisx;
Binary::XOR::XORconst Binary& a const Binary& b : aa bb
Binary::XOR::operator Binary const
return Binaryax bx;
Binary::XOR::operator unsigned int const
return ax bx;
Binary::AND::ANDconst Binary& a const Binary& b : aa bb
Binary::AND::operator Binary const
return Binaryax & bx;
Binary::AND::operator unsigned int const
return ax & bx;
Binary::COMBO::COMBOconst Binary& a const XOR& b : aa bb
Binary::COMBO::operator Binary const
return Binaryax bax bbx;
Binary::COMBO::operator unsigned int const
return ax bax bbx;
End of File
Sample Test Code :
Combo
Binary ax;
Binary ax;
Binary ax;
Binary aBinary;
unsigned int aValue;
Binary tBinary;
unsigned int tValue;
Binary ttBinary;
unsigned int ttValue;
Trace::outaxx
ax;
Trace::outaxx
ax;
Trace::outaxx
ax;
Trace::out
;
aBinary a & a a;
aValue a & a a;
Trace::outaBinary a & a axx
aBinary.x;
Trace::outaValue a & a axx
aValue;
Trace::out
;
Sample Test Output :
ax
ax
ax
aBinary a & a ax
aValue a & a ax
However, the problem currently occurring in the above code output :
ax
ax
ax
aBinary a & a ax
aValue a & a ax
The test fails, x is an invalid output.
If I calculate a & aand a a separately
a & ax
a ax
it seems like aa should be calculated first to get the result of x but I don't know how.
Please modify the COMBO part so that I get the same result as the sample output,
a & a ax
NEVER NEVER NEVER Chenge test code to like a & a a
Please fix Binary.h and Binary.cpp
Chegg give wrong answer. I didn't ask about Java project in NetBeans
Step by Step Solution
There are 3 Steps involved in it
Step: 1
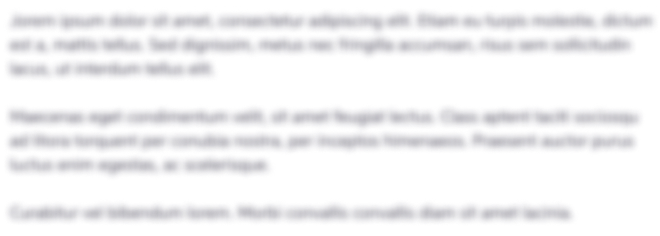
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started