Question
C++ Stack Class Program: In this assignment, you will be writing and testing the Stack data structure class. The stack data structure will stack the
C++ Stack Class Program: In this assignment, you will be writing and testing the Stack data structure class. The stack data structure will stack the data. This means it will add new data to the top of the stack, and remove data from the top. For this assignment, we will be building a stack that will hold integers.
Task: Write and test your Stack class by implementing the following member functions:
constructor: create the empty stack and initialize your all member variables
OPTIONAL: destructor: to release any memory for data that is still in the stack
push: add an integer argument to the top of the stack
pop: return the integer argument that is at the top of the stack and removes it from the stack. If the stack is empty, then return -999.
top: returns a copy of the top integer from the stack without removing any data. If the stack is empty, then return -999.
isEmpty: returns true/false on whether the stack is empty or not
getCount: returns the number of integers that are currently stored in the stack. This count should be kept up to date as integers are being pushed and poped off the stack
USE THE CODE BELOW:
#include
using namespace std;
class Node {
public:
Node(int num ) {
data = num;
next = nullptr;
}
int data; //purposely public
Node* next; }; // end of Node class
class Stack {
public:
Stack(){ // default constructor: create the empty stack and initialize your all member variables // your code... }
~Stack() { // OPTIONAL destructor // your code...and so on for each of the member functions }
void push( int num ) { } // you will need to add real code
int pop( ) { return -99; }
int top( ) { return -99; }
bool isEmpty( ) { return true; }
int getCount( ) { return -99; }
private:
Node* head;
int count;
}; // end of Stack class
int main( ){
Stack stack; // calls constructor
cout << "Before pushing ";
cout << "Stack size: " << stack.getCount() << endl;
cout << "Stack is " << (stack.isEmpty() == true? "empty ": "not empty ");
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
cout << " After pushing 1-5 ";
cout << "Stack size: " << stack.getCount() << endl;
cout << "Stack is " << (stack.isEmpty() == true ? "empty ": "not empty ");
cout << "Top of the stack is: " << stack.top() << " "; // example output given below for above lines
cout << " Popping off the stack: " << stack.pop() << endl;
cout << "Popping off the stack: " << stack.pop() << endl;
cout << "Stack size: " << stack.getCount() << endl;
cout << "Stack is " << (stack.isEmpty() == true ? "empty ": "not empty ");
cout << "Top of the stack is: " << stack.top() << " ";
stack.push(6);
stack.push(7);
cout << "After pushing 6-7 ";
cout << "Stack size: " << stack.getCount() << endl;
cout << "Stack is " << (stack.isEmpty() == true ? "empty ": "not empty ");
cout << "Top of the stack is: " << stack.top() << " ";
stack.push(8);
stack.push(9);
cout << "After pushing 8-9 ";
cout << "Stack size: " << stack.getCount() << endl;
cout << "Stack is " << (stack.isEmpty() == true ? "empty ": "not empty ");
cout << "Top of the stack is: " << stack.top() << " ";
while( stack.isEmpty() == false) {
cout << "Popping off the stack: " << stack.pop() << endl;
}
cout << "Stack size: " << stack.getCount() << endl;
cout << "Stack is " << (stack.isEmpty() == true ? "empty ": "not empty ");
cout << "Top of the stack is: " << stack.top() << " ";
} // end of main
Example output for first part of main, if functioning correctly:
Stack size: 0
Stack is empty
After pushing 1-5
Stack size: 5
Stack is not empty
Top of the stack is 5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
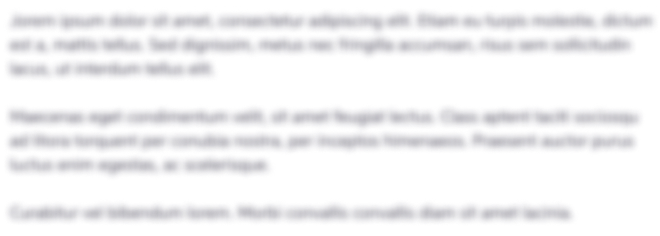
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started