Question
Can anyone solve these in Java Eclipse? package practicePackage._06_sorting.attempts; public class Stage2 { /** * * @param data * @return true if data is sorted
Can anyone solve these in Java Eclipse?
package practicePackage._06_sorting.attempts;
public class Stage2 {
/**
*
* @param data
* @return true if data is sorted in either ascending or descending order, false
* otherwise.
*/
public static boolean isSorted(int[] data) {
return false; //to be completed
}
/**
* ADVANCED: there exists an O(nlogn) solution using merge sort (which will be
* covered in 2010)
*
* @param data
* @return the number of swaps needed to sort the array using bubble sort.
* Return -1 if data is invalid.
*/
public static int bubbleSortCount(int[] data) {
return -1; //to be completed
}
/**
*
* @param data
* @return the number of items you need to shift to sort the array using
* insertion sort. Return -1 if data is invalid.
*/
public static int insertionSortCount(int[] data) {
return -1; //to be completed
}
}
package practicePackage._06_sorting.testAttempts;
import static org.junit.jupiter.api.Assertions.*;
import java.util.Arrays;
import org.junit.jupiter.api.Test;
import practicePackage._06_sorting.attempts.*;
public class TestStage2 {
@Test
public void testIsSorted() {
assertFalse(Stage2.isSorted(null));
assertFalse(Stage2.isSorted(new int[] { 10, 20, 70, 90, 5 }));
assertFalse(Stage2.isSorted(new int[] { 90, 70, 4, 20, 10 }));
assertFalse(Stage2.isSorted(new int[] { 5, 90, 70, 20, 10 }));
assertFalse(Stage2.isSorted(new int[] { 10, 10, 10, 10, 5, 40, 80, 90 }));
assertTrue(Stage2.isSorted(new int[] { 10 }));
assertTrue(Stage2.isSorted(new int[] { 5, 10 }));
assertTrue(Stage2.isSorted(new int[] { 10, 5 }));
assertTrue(Stage2.isSorted(new int[] { 10, 20, 70, 90 }));
assertTrue(Stage2.isSorted(new int[] { 90, 70, 20, 10 }));
assertTrue(Stage2.isSorted(new int[] { 10, 20, 20, 70, 90 }));
assertTrue(Stage2.isSorted(new int[] { 90, 70, 20, 10, 10 }));
assertTrue(Stage2.isSorted(new int[] { 10, 10, 10, 10, 35, 40, 80, 90 }));
assertTrue(Stage2.isSorted(new int[] { 10, 10, 10, 10, 9, 8, 7 }));
}
@Test
public void testBubbleSortCount() {
assertEquals(-1, Stage2.bubbleSortCount(null));
assertEquals(0, Stage2.bubbleSortCount(new int[] {}));
assertEquals(0, Stage2.bubbleSortCount(new int[] { 10, 20, 70, 90 }));
assertEquals(1, Stage2.bubbleSortCount(new int[] { 10, 70, 20, 90 }));
assertEquals(3, Stage2.bubbleSortCount(new int[] { 10, 70, 5, 20, 90 }));
assertEquals(4, Stage2.bubbleSortCount(new int[] { 10, 70, 5, 20, 20, 90 }));
assertEquals(5, Stage2.bubbleSortCount(new int[] { 10, 70, 5, 20, 90, 20 }));
assertEquals(10, Stage2.bubbleSortCount(new int[] { 90, 70, 20, 10, 5 }));
assertEquals(11, Stage2.bubbleSortCount(new int[] { 40, 70, 20, 90, 30, 80, 20 }));
assertEquals(14, Stage2.bubbleSortCount(new int[] { 2, 3, -4, 7, 5, 1, 10, 12, 6, 8, 9 }));
}
@Test
public void testInsertionSortCount() {
assertEquals(-1, Stage2.insertionSortCount(null));
assertEquals(0, Stage2.insertionSortCount(new int[] {}));
assertEquals(0, Stage2.insertionSortCount(new int[] { 10, 20, 70, 90 }));
assertEquals(1, Stage2.insertionSortCount(new int[] { 10, 70, 20, 90 }));
assertEquals(3, Stage2.insertionSortCount(new int[] { 10, 70, 5, 20, 90 }));
assertEquals(5, Stage2.insertionSortCount(new int[] { 10, 70, 5, 20, 20, 90 }));
assertEquals(6, Stage2.insertionSortCount(new int[] { 10, 70, 5, 20, 90, 20 }));
assertEquals(10, Stage2.insertionSortCount(new int[] { 90, 70, 20, 10, 5 }));
assertEquals(12, Stage2.insertionSortCount(new int[] { 40, 70, 20, 90, 30, 80, 20 }));
assertEquals(14, Stage2.insertionSortCount(new int[] { 2, 3, -4, 7, 5, 1, 10, 12, 6, 8, 9 }));
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
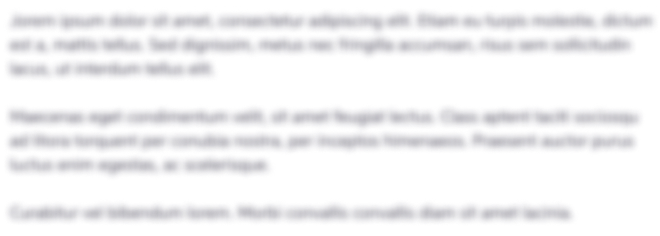
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started