Can I get help, with the exact information that I have on the pictures please.
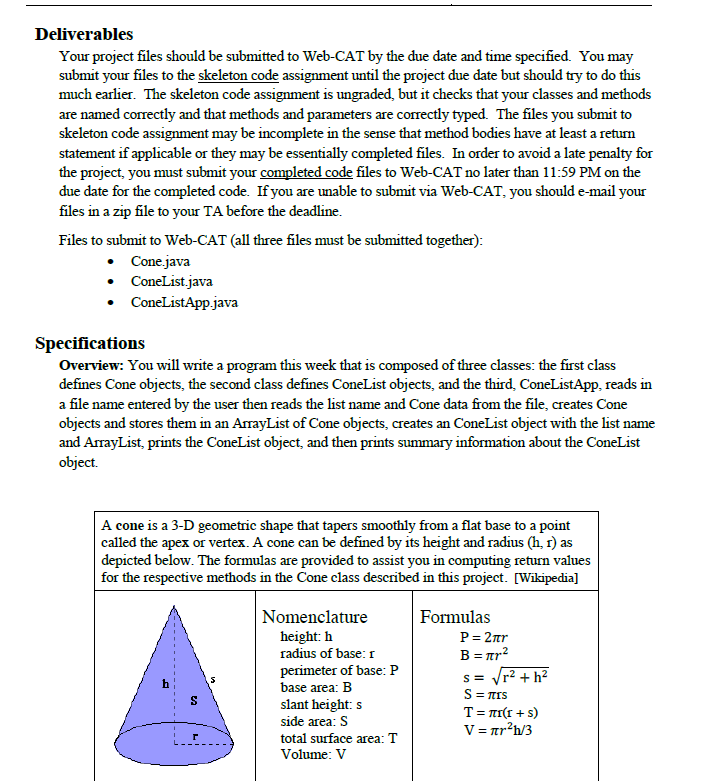
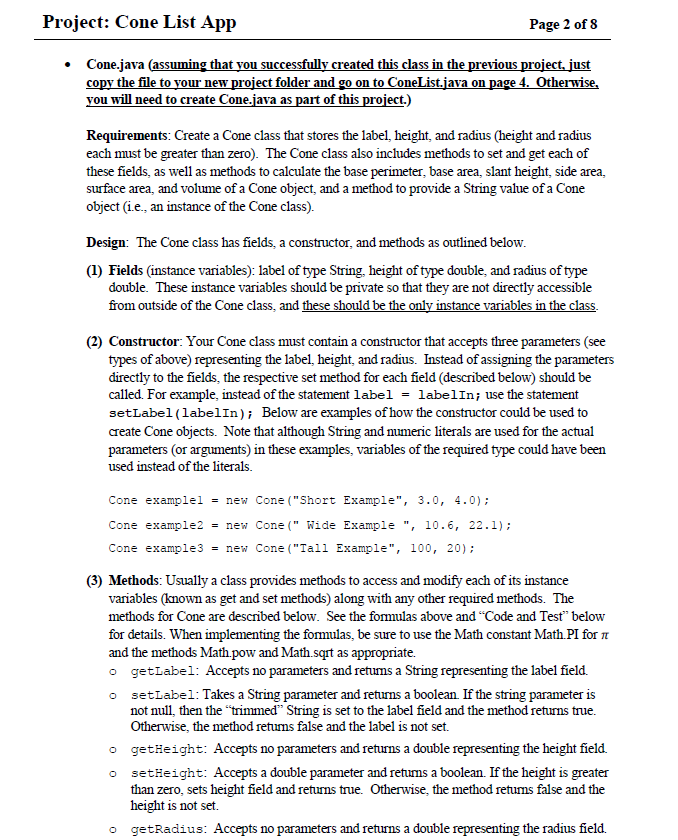
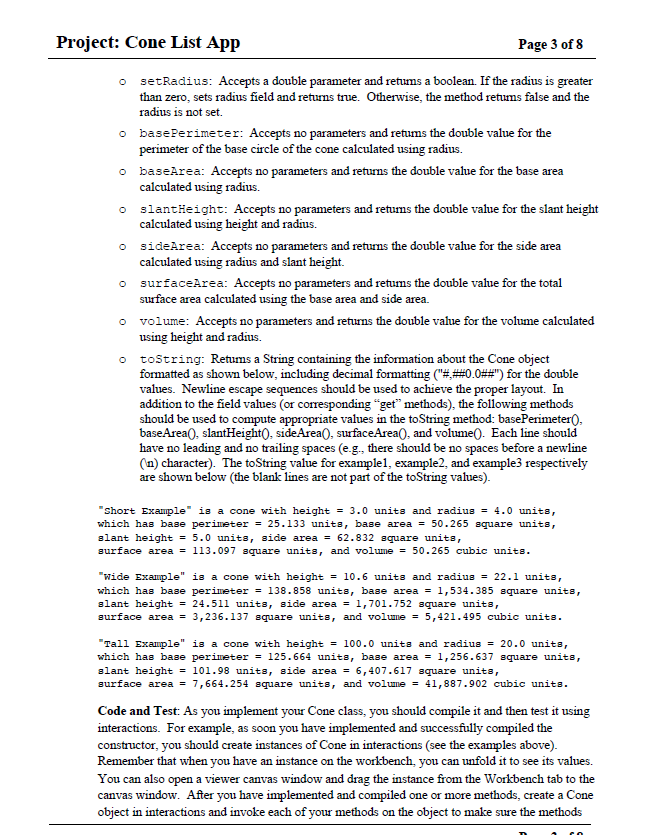
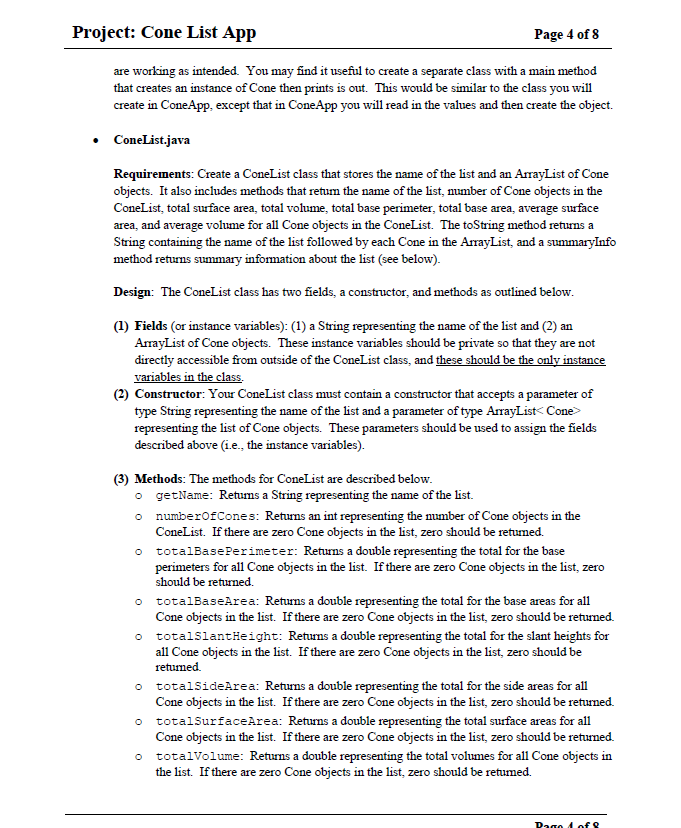
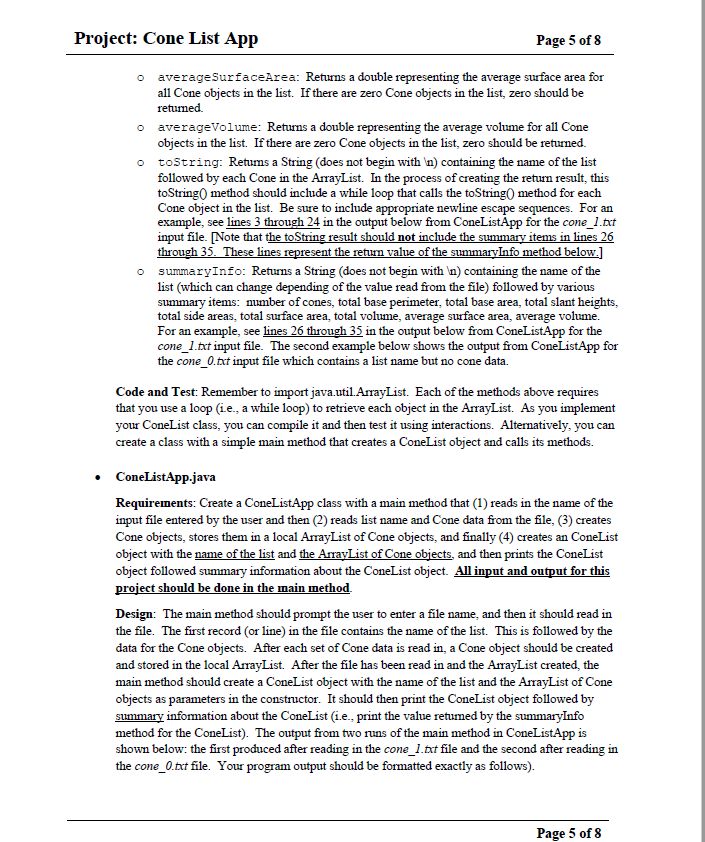
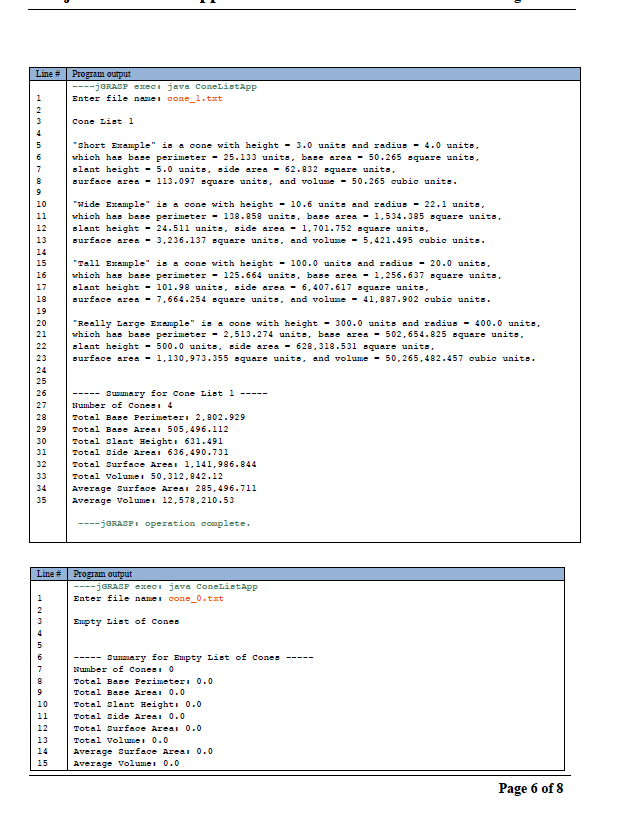
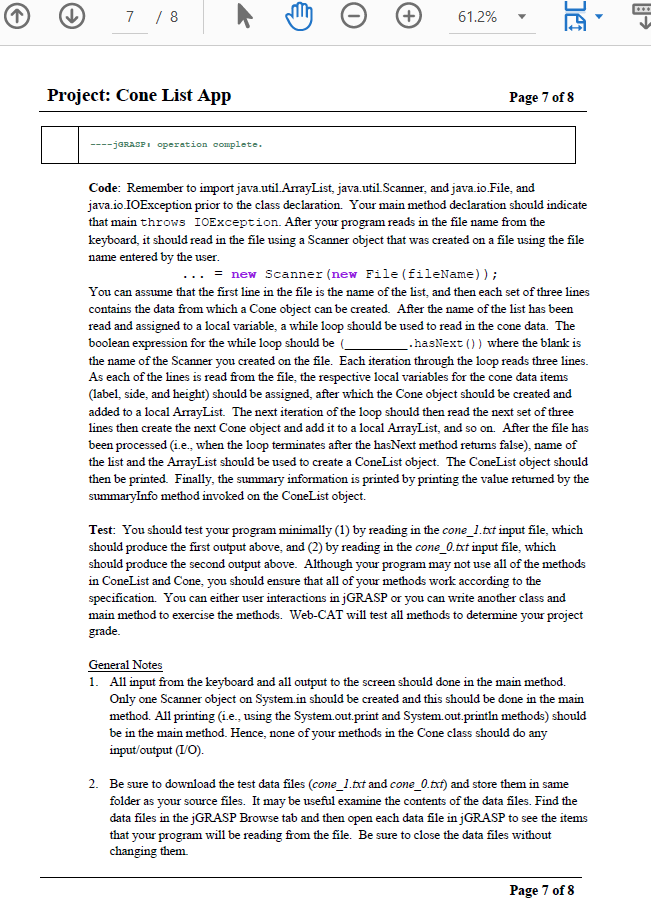
Deliverables Your project files should be submitted to Web-CAT by the due date and time specified. You may submit your files to the skeleton code assignment until the project due date but should try to do this much earlier. The skeleton code assignment is ungraded, but it checks that your classes and methods are named correctly and that methods and parameters are correctly typed. The files you submit to skeleton code assignment may be incomplete in the sense that method bodies have at least a return statement if applicable or they may be essentially completed files. In order to avoid a late penalty for the project, you must submit your completed code files to Web-CAT no later than 11:59 PM on the due date for the completed code. If you are unable to submit via Web-CAT, you should e-mail your files in a zip file to your TA before the deadline. Files to submit to Web-CAT (all three files must be submitted together): Cone.java ConeList.java ConeListApp.java Specifications Overview: You will write a program this week that is composed of three classes: the first class defines Cone objects, the second class defines ConeList objects, and the third, ConeListApp, reads in a file name entered by the user then reads the list name and Cone data from the file, creates Cone objects and stores them in an ArrayList of Cone objects, creates an ConeList object with the list name and ArrayList, prints the ConeList object, and then prints summary information about the ConeList object. A cone is a 3-D geometric shape that tapers smoothly from a flat base to a point called the apex or vertex. A cone can be defined by its height and radius (h. 1) as depicted below. The formulas are provided to assist you in computing return values for the respective methods in the Cone class described in this project. (Wikipedia] Nomenclature height: h radius of base: 1 perimeter of base:P base area: B slant height: s side area: S total surface area: T Volume: V Formulas P=2r = 2 s= r2 + ha S = TIS T = 1/(1+) V=nr2h/3 Project: Cone List App Page 2 of 8 Cone.java (assuming that you successfully created this class in the previous project, just copy the file to your new project folder and go on to ConeList.java on page 4. Otherwise, you will need to create Cone.java as part of this project.) Requirements: Create a Cone class that stores the label, height, and radius (height and radius each must be greater than zero). The Cone class also includes methods to set and get each of these fields, as well as methods to calculate the base perimeter, base area, slant height, side area, surface area, and volume of a Cone object, and a method to provide a String value of a Cone object (i.e. an instance of the Cone class). Design: The Cone class has fields, a constructor, and methods as outlined below. (1) Fields (instance variables): label of type String, height of type double, and radius of type double. These instance variables should be private so that they are not directly accessible from outside of the Cone class, and these should be the only instance variables in the class. (2) Constructor: Your Cone class must contain a constructor that accepts three parameters (see types of above) representing the label, height, and radius. Instead of assigning the parameters directly to the fields, the respective set method for each field (described below) should be called. For example, instead of the statement label = labelIn; use the statement setLabel(labelIn); Below are examples of how the constructor could be used to create Cone objects. Note that although String and numeric literals are used for the actual parameters (or arguments) in these examples, variables of the required type could have been used instead of the literals. Cone examplel = new Cone ("Short Example", 3.0, 4.0); Cone example2 = new Cone(" Wide Example ", 10.6, 22.1); Cone example3 = new Cone ("Tall Example", 100, 20); (3) Methods: Usually a class provides methods to access and modify each of its instance variables (known as get and set methods) along with any other required methods. The methods for Cone are described below. See the formulas above and "Code and Test" below for details. When implementing the formulas, be sure to use the Math constant Math.PI for it and the methods Math.pow and Math.sqrt as appropriate. o getLabel: Accepts no parameters and retums a String representing the label field. osetLabel: Takes a String parameter and returns a boolean If the string parameter is not null, then the "trimmedString is set to the label field and the method returns true. Otherwise, the method retums false and the label is not set. o getHeight: Accepts no parameters and returns a double representing the height field. o setHeight: Accepts a double parameter and returns a boolean. If the height is greater than zero, sets height field and returns true. Otherwise, the method returns false and the height is not set. o getRadius: Accepts no parameters and returns a double representing the radius field. Project: Cone List App Page 3 of 8 o setRadius: Accepts a double parameter and retums a boolean. If the radius is greater than zero, sets radius field and returns true. Otherwise, the method retums false and the radius is not set. o basePerimeter: Accepts no parameters and retums the double value for the perimeter of the base circle of the cone calculated using radius. o baseArea: Accepts no parameters and returns the double value for the base area calculated using radius. o slantHeight: Accepts no parameters and returns the double value for the slant height calculated using height and radius. o sideArea: Accepts no parameters and returns the double value for the side area calculated using radius and slant height. o surfaceArea: Accepts no parameters and retums the double value for the total surface area calculated using the base area and side area. o volume: Accepts no parameters and returns the double value for the volume calculated using height and radius. otoString: Retums a String containing the information about the Cone object formatted as shown below, including decimal formatting ("0.0") for the double values. Newline escape sequences should be used to achieve the proper layout. In addition to the field values (or corresponding "get" methods), the following methods should be used to compute appropriate values in the toString method: base Perimeter), baseArea(), slantHeight(), side Area, surface Area), and volume(). Each line should have no leading and no trailing spaces (eg., there should be no spaces before a newline (n) character). The toString value for examplel, example2, and example3 respectively are shown below (the blank lines are not part of the toString values). "Short Example" is a cone with height = 3.0 units and radius = 4.0 units, which has base perimeter = 25.133 units, base area = 50.265 square units, slant height = 5.0 units, side area = 62.832 aquare units, surface area = 113.097 square units, and volume = 50.265 cubic units. "Wide Example" is a cone with height = 10.6 units and radius = 22.1 units, which has base perimeter = 138.858 units, base area = 1,534.385 square units, slant height = 24.511 units, side area = 1,701.752 square units, surface area = 3,236.137 square units, and volume = 5,421.495 cubic units. "Tall Example" is a cone with height = 00.0 units and radius = 20.0 units, which has base perimeter = 125.664 units, base area = 1,256.637 square units, slant height = 101.98 units, side area = 6,407.617 square units, surface area = 7,664.254 square units, and volume = 41,887.902 cubic units. Code and Test: As you implement your Cone class, you should compile it and then test it using interactions. For example, as soon you have implemented and successfully compiled the constructor, you should create instances of Cone in interactions (see the examples above). Remember that when you have an instance on the workbench, you can unfold it to see its values. You can also open a viewer canvas window and drag the instance from the Workbench tab to the canvas window. After you have implemented and compiled one or more methods, create a Cone object in interactions and invoke each of your methods on the object to make sure the methods Project: Cone List App Page 4 of 8 are working as intended. You may find it useful to create a separate class with a main method that creates an instance of Cone then prints is out. This would be similar to the class you will create in Cone App, except that in Cone App you will read in the values and then create the object. ConeList.java Requirements: Create a ConeList class that stores the name of the list and an ArrayList of Cone objects. It also includes methods that retum the name of the list, number of Cone objects in the ConeList, total surface area, total volume, total base perimeter, total base area, average surface area, and average volume for all Cone objects in the ConeList. The toString method returns a String containing the name of the list followed by each Cone in the ArrayList, and a summaryInfo method returns summary information about the list (see below). Design: The ConeList class has two fields, a constructor, and methods as outlined below. (1) Fields (or instance variables): (1) a String representing the name of the list and (2) an ArrayList of Cone objects. These instance variables should be private so that they are not directly accessible from outside of the ConeList class, and these should be the only instance variables in the class. (2) Constructor: Your ConeList class must contain a constructor that accepts a parameter of type String representing the name of the list and a parameter of type ArrayList