Question
Can I please get help on this python project? Part 1: Dice Rolling Simulator Goal: This project involves writing a program that simulates the rolling
Can I please get help on this python project?
Part 1: Dice Rolling Simulator
Goal: This project involves writing a program that simulates the rolling of dice. When the program runs, it will randomly choose a number between 1 and 6. It will then use that number to index the LIST, and printed the result to the screen.
"""
import random
"""
Task 1: Create a LIST of the die faces, with the variable name of mydice_list.
Example:
- a die face of 1 should be represented with a single "."
- a die face of 6 should be represented by "..."
"""
### YOUR CODE GOES HERE ###
"""
Task 2: Print a random die face to the screen from mydice_list that corresponds to the value of random_dice.
random_dice: a variable that stores the value of what is returned from random.randint().
random.randint(0,5) will return a random value between 0 and 5.
Think about how you can use LIST indexes to select a value from a LIST of data """
# use random_dice value to select and print the die face to the screen
# from mydice_list random_dice = random.randint(0,5)
# print the die face to the screen
### YOUR CODE GOES HERE ###
"""
Part 2: Dice Rolling Simulator with Loops
Goal: This project involves writing a program that simulates the rolling of dice using LOOPS. Using your experience from Part 1, and your newly learned knowledge of LOOPS, complete the following Tasks
"""
Task 1: Get input from the user by asking them how many dice they would like to roll, and store that number in a variable named how_many.
Then write a FOR loop that "rolls" how_many number of dice, printing each die face to the screen.
TIP: You are doing what you did in Part 1 how_many number of times
Example: User supplies 3. Store 3 into variable named how_many. Then output the three random die faces to the screen from mydice_list
'.'
'....'
'..'
If user supplies 1, output should only show one random die face from mydice_list '......'
"""
# Task 1: for loop
### YOUR CODE HERE ###
"""
Task 2: Comment out the code you typed above.
When you run the code above, your FOR loop runs and the program ends.
Using a WHILE loop, continue to ask the user for input (how many dice to roll) until they input 0. Only when the user inputs 0 should the program end.
"""
# Task 2: while loop
### YOUR CODE HERE ###
"""
Part 3: Dice Rolling Simulator with Functions
Goal: This project involves writing a program that simulates the rolling of dice using FUNCTIONS. Using your experience from Part 1 and 2, and your newly learned knowledge of FUNCTIONS, complete the following Tasks
TIP: We are creating functions out of the code we wrote in Part 2.
"""
import random
"""
Task 1: In Part 1, you created a list of die faces named mydice_list. You are doing the same here, except now you will create a function that will create the list of die faces and return that list.
"""
def dice_list():
"""Create and return a list of die faces"""
### YOUR CODE HERE ###
"""
Task 2: In Part 1 and Part 2, you selected a random die face from mydice_list using random.randint(). You are doing the same here, except now you will create a function that will call dice_list() to create a list of die faces, and then select a random die face from that list and return it.
"""
def dice_roll():
"""
Return a randomly selected die face from the list of dice faces that is returned from calling dice_list() """
### YOUR CODE HERE ###
"""
Task 3: Create a function that creates a list of "rolled" dice. The function should take in 0 or 1 arguments. If no arguments are passed the function should default to 1.
TIP: What kind of loop can we use when we know exactly how many times it needs to run?
"""
def roll_lots_of_dice(how_many=1):
"""
Return a list of "rolled dice".
"""
### YOUR CODE HERE ###
"""
Task 4: All code below here should only run if we are in "__main__"
"""
### YOUR CODE HERE ###
"""
Task 5: Similar to Part 2, continue to ask the user for input (how many dice to roll) until they input 0. Only when the user inputs 0 should the program end.
You'll need to call a function to "roll" the dice
Output the LIST of all the "rolled" die faces
"""
### YOUR CODE HERE ###
"""
Part 4: Counting the Dice
Goal: Now that we can roll some dice, we need to be able to count each die and add up their total value.
Reuse some of the functions you wrote in Part 3, and we'll create two more functions that will find the value of each rolled die and sum them all up.
"""
import random def dice_list():
"""
Create and return a list of die faces
"""
### YOUR CODE HERE ###
def dice_roll():
"""
Return a randomly selected die face from the list of dice faces that is returned from calling dice_list()
"""
### YOUR CODE HERE ###
def roll_lots_of_dice(how_many=1):
"""Return a list of "rolled dice"."""
### YOUR CODE HERE ###
"""
Task 1: Create a function that takes in a die face, and returns its INTEGER value.
"""
def get_dice_value(die_face):
"""
Returns the INTEGER value of a die face.
Example:
-If ".." is passed as argument, then 2 should be returned
-If "....." is passed as argument, then 5 should be returned
"""
### YOUR CODE HERE ###
"""
Task 2: Create a function that takes in a LIST of the rolled die faces and returns the sum.
Example:
-If ['.', '...'] is passed in, should return 4
-If ['...', '..', '.'] is passed in, should return 6
"""
def add_up_rolled_dice_faces(list_of_rolled_faces):
""" Returns the sum of a list of rolled die faces."""
### YOUR CODE HERE ###
if __name__ == "__main__":
"""
Task 3: Keep asking the user for input (how many dice to roll) until they input 0. Output the LIST of all the "rolled" die faces each time, and the sum of those dice
"""
### YOUR CODE HERE ###
"""
PART 5: Guessing Game
Goal: Building upon Parts 1-4, we will now create a guessing game. This program will ask the user how many dice to roll, then allow the user to keep guessing what the total value of dice sum is until they get it correct. If the user guesses too high, you should output "Too high" If the user guesses too low, you should output "Too low"
BONUS:
-Give the user X number of guesses
-Give the user X number of guesses based on how many dice are rolled. ex. more dice means more guesses
-Read in the dice list from a file -Have the computer "guess" the random number
"""
import random
def dice_list():
"""Create and return a list of die faces"""
### YOUR CODE HERE ###
def dice_roll():
"""
Return a randomly selected die face from the list of dice faces that is returned from calling dice_list()
"""
### YOUR CODE HERE ###
def roll_lots_of_dice(how_many=1):
"""Return a list of "rolled dice"."""
### YOUR CODE HERE ###
def get_dice_value(die_face):
""" Returns the INTEGER value of a die face."""
### YOUR CODE HERE ###
def add_up_rolled_dice_faces(list_of_rolled_faces):
""" Returns the sum of a list of rolled die faces."""
### YOUR CODE HERE ###
if __name__ == "__main__":
"""
Task 1:Ask the user how many dice they would like to roll, and allow the user to guess what the total value is of the rolled dice until they get it right.
Give the user hints such as "too high" or "too low" to help them out after each guess.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
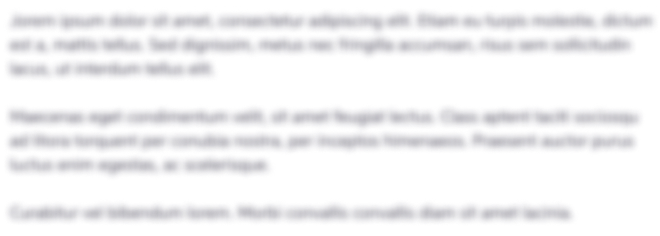
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started