Question
Can some one help me on how to debug the error below. Exception in thread main java.lang.IndexOutOfBoundsException: Index: 0, Size: 0 at java.util.ArrayList.rangeCheck(ArrayList.java:653) at java.util.ArrayList.get(ArrayList.java:429)
Can some one help me on how to debug the error below.
Exception in thread "main" java.lang.IndexOutOfBoundsException: Index: 0, Size: 0
at java.util.ArrayList.rangeCheck(ArrayList.java:653)
at java.util.ArrayList.get(ArrayList.java:429)
at ArrayVsArrayList.increment(ArrayVsArrayList.java:235)
at ArrayVsArrayList.main(ArrayVsArrayList.java:64)
----jGRASP wedge2: exit code for process is 1.
----jGRASP: operation complete.
import java.util.ArrayList; import java.util.Random; import java.text.DecimalFormat; import java.util.*; import java.nio.file.*; import java.io.*; import java.text.*; import java.util.concurrent.TimeUnit; /*
Description Compare ArrayList and array for time efficiency in multiple operations.
*/ public class ArrayVsArrayList{
private ArrayList
static int trials;
public ArrayVsArrayList(){ listInt = new ArrayList
public void fill(){ int i, j; float lapsedTimeArrayListFloat; int lapsedTimeArrayInt; float lapsedTimeArrayFloat; int lapsedTimeArrayListInt; int lapsedArrayListForCpuInt; float lapsedArrayListForCpuFloat; int lapsedArrayForCpuInt; float lapsedArrayForCpuFloat; // fill the Array lapsedTimeArrayInt = 0; lapsedTimeArrayFloat = 0.1f; lapsedArrayForCpuInt = 0; lapsedArrayForCpuFloat = 0.1f; startTimeInt = (int) System.currentTimeMillis(); startSystemTimeInt = (int) System.nanoTime(); for (j = 0; j < intArray.length; j++){ intArray[j] = rand.nextInt(DATA_LIMIT_INT); } endTimeInt = (int) System.currentTimeMillis(); endSystemTimeInt = (int) System.nanoTime(); lapsedTimeArrayInt = (endTimeInt - startTimeInt)/MILLI_SECONDS_INT; lapsedArrayForCpuInt = (endSystemTimeInt - startSystemTimeInt)/NON_SECONDS_INT; startTimeFloat = (float) System.currentTimeMillis(); startSystemTimeFloat = (float) System.nanoTime(); for (j = 0; j < floatArray.length; j++){ floatArray[j] = rand.nextFloat(); } endTimeFloat = (float) System.currentTimeMillis(); endSystemTimeFloat = (float) System.nanoTime(); lapsedTimeArrayFloat = (endTimeInt - startTimeInt)/MILLI_SECONDS_FLOAT; lapsedArrayForCpuFloat = (endSystemTimeFloat - startSystemTimeFloat)/NON_SECONDS_FLOAT; // fill ArrayList lapsedTimeArrayListFloat = 0.1f; lapsedTimeArrayListInt = 0; lapsedArrayForCpuFloat = 0.1f; lapsedArrayListForCpuInt = 0; startTimeInt = (int) System.currentTimeMillis(); startSystemTimeInt = (int) System.nanoTime(); for (j = 0; j < SIZE_INT; j++){ listInt.add(j, rand.nextInt(DATA_LIMIT_INT)); } endTimeInt = (int) System.currentTimeMillis(); endSystemTimeInt = (int) System.nanoTime(); lapsedTimeArrayListInt = (endTimeInt - startTimeInt)/MILLI_SECONDS_INT; lapsedArrayListForCpuInt = (endSystemTimeInt - startSystemTimeInt)/NON_SECONDS_INT; startTimeFloat = (float) System.currentTimeMillis(); startSystemTimeFloat = (float) System.nanoTime(); for (j = 0; j < SIZE_INT; j++){ listInt.add(j, rand.nextInt(DATA_LIMIT_INT)); } endTimeFloat = (float) System.currentTimeMillis(); endSystemTimeFloat = (float) System.nanoTime(); lapsedTimeArrayListFloat = (endTimeFloat - startTimeFloat)/MILLI_SECONDS_FLOAT; lapsedArrayForCpuFloat = (endSystemTimeFloat - startSystemTimeFloat)/NON_SECONDS_FLOAT; outputString = "Run # " + trials + LS + "Fill the list: Number of Elements: " + DATA_LIMIT_INT + LS + " int array - wall clock : " + formatter.format(lapsedTimeArrayInt) + LS + " int ArrayList - wall clock : " + formatter.format(lapsedTimeArrayListInt) + LS + LS + " int array - CPU time : " + formatter.format(lapsedArrayForCpuInt) + LS + " int ArrayList - CPU time : " + formatter.format(lapsedArrayListForCpuInt) + LS + LS + " float array - wall clock : " + formatter.format(lapsedTimeArrayFloat) + LS + " float ArrayList - wall clock : " + formatter.format(lapsedTimeArrayListFloat) + LS + LS + " float array - CPU time : " + formatter.format(lapsedArrayForCpuFloat) + LS + " float ArrayList - CPU time : " + formatter.format(lapsedArrayListForCpuInt) + LS + LS; System.out.println(outputString); writeReport(outputString); }// end of fill
public void increment(){ int i, j; float lapsedTimeArrayListFloat; int lapsedTimeArrayInt; float lapsedTimeArrayFloat; int lapsedTimeArrayListInt; int lapsedArrayListForCpuInt; float lapsedArrayListForCpuFloat; int lapsedArrayForCpuInt; float lapsedArrayForCpuFloat; // sum Array lapsedTimeArrayInt = 0; lapsedTimeArrayFloat = 0.1f; lapsedArrayForCpuInt = 0; lapsedArrayForCpuFloat = 0.1f; startTimeInt = (int) System.currentTimeMillis(); startSystemTimeInt = (int) System.nanoTime(); for( j = 0; j < intArray.length; j++){ intArray[j]++; } endTimeInt = (int) System.currentTimeMillis(); endSystemTimeInt = (int) System.nanoTime(); lapsedTimeArrayInt = (endTimeInt - startTimeInt)/MILLI_SECONDS_INT; lapsedArrayForCpuInt = (endSystemTimeInt - startSystemTimeInt)/NON_SECONDS_INT; startTimeFloat = (float) System.currentTimeMillis(); startSystemTimeFloat = (float) System.nanoTime(); for( j = 0; j < floatArray.length; j++){ floatArray[j]++; } endTimeFloat = (float) System.currentTimeMillis(); endSystemTimeFloat = (float) System.nanoTime(); lapsedTimeArrayFloat = (endTimeInt - startTimeInt)/MILLI_SECONDS_FLOAT; lapsedArrayForCpuFloat = (endSystemTimeFloat - startSystemTimeFloat)/NON_SECONDS_FLOAT; // sum ArrayList lapsedTimeArrayListFloat = 0; lapsedArrayListForCpuInt = 0; startTimeInt = (int) System.currentTimeMillis(); startSystemTimeInt = (int) System.nanoTime(); for( i = 0; i < COLLECTION_SIZE; i++){ int incremented = listInt.get(i) + 1; listInt.set(i, incremented); } endTimeInt = (int) System.currentTimeMillis(); endSystemTimeInt = (int) System.nanoTime(); lapsedTimeArrayListInt = (endTimeInt - startTimeInt)/MILLI_SECONDS_INT; lapsedArrayListForCpuInt = (endSystemTimeInt - startSystemTimeInt)/NON_SECONDS_INT; startTimeFloat = (float) System.currentTimeMillis(); startSystemTimeFloat = (float) System.nanoTime(); for( i = 0; i < COLLECTION_SIZE; i++){ float incremented = floatList.get(i) + 1; floatList.set(i, incremented); } endTimeFloat = (float) System.currentTimeMillis(); endSystemTimeFloat = (float) System.nanoTime(); lapsedTimeArrayListFloat = (endTimeFloat - startTimeFloat)/MILLI_SECONDS_FLOAT; lapsedArrayForCpuFloat = (endSystemTimeFloat - startSystemTimeFloat)/NON_SECONDS_FLOAT; outputString = "Increment the elements in the list: " + LS + " int array - wall clock : " + formatter.format(lapsedTimeArrayInt) + LS + " int ArrayList - wall clock : " + formatter.format(lapsedTimeArrayListInt) + LS + LS + " int array - CPU time : " + formatter.format(lapsedArrayForCpuInt) + LS + " int ArrayList - CPU time : " + formatter.format(lapsedArrayListForCpuInt) + LS + LS + " float array - wall clock : " + formatter.format(lapsedTimeArrayFloat) + LS + " float ArrayList - wall clock : " + formatter.format(lapsedTimeArrayListFloat) + LS + LS + " float array - CPU time : " + formatter.format(lapsedArrayForCpuFloat) + LS + " float ArrayList - CPU time : " + formatter.format(lapsedArrayListForCpuInt) + LS + LS; System.out.println(outputString); writeReport(outputString); listInt.clear(); floatList.clear(); }//end of increment private void writeReport(String reportString){ try{ BufferedWriter writer = new BufferedWriter(new FileWriter(new File(fileName), true)); writer.write(reportString); writer.close(); }catch(Exception ex){ System.out.println("try a different file name"); } }
}// end of class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
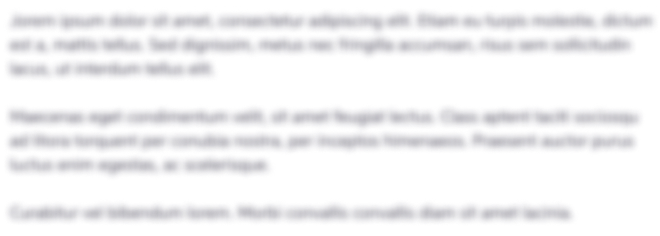
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started