Answered step by step
Verified Expert Solution
Question
1 Approved Answer
can someone ease help me with the implementatio of the following game of cribbage using the following guidelines Requirements To consolidate: Create a library and
can someone ease help me with the implementatio of the following game of cribbage using the following guidelines
Requirements To consolidate: Create a library and sample program for above, and following the marking scheme below Make sure to follow proper Cribbage scoring for hands/cribs .Take special note of where you should have toString's Tips and Suggestions Of course, the most important thing is to work out what you're doing long before you start coding. However, in addition to that: This isn't a data structures course. You're welcome to use Java's built-in LinkedList, ArrayList, etc. Of course, you need basic conventions: o Put your name and student number at the top of each file in commenting o Document each class and method, in JavaDoc convention Everything goes into a deck package Actually, it's up to you whether or not you want your Main class/program to be in the same package or a different one, but everything else does need to be in there There's a reason the cribbage hand is 'four cards plus the turn-up? when playing for real, the turn-up is counted with both player's hands (as well as with the crib) o So, while although most cards are drawn directly into hands, your main program will need to first o Well, I guess the better reason is it's also necessary for counting 'his nobs' There are multiple ways to count the same thing. So long as the total points work out identically, don't worry too much about how you add them o eg. for [7H | 8S, 7S, 7C, AD], the three of a kind' is worth 6 points. Why? You can think of it as 'three pairs': [7H, 7S], 17H, 7C], and [7S, 7C] -You can simply think of it as 'three of a kind', so long as it gives the same 6 points In case it's never come up for you: the reason we include toString so often is because Java invokes it automatically when it expects a String representation of an object o e.g. System.out.println (myCard) wll totally work It's considered acceptable for the sequence of a hand to be changed during tallying, if that helps o i.e. even though you probably won't need to, if your tallying ends up shuffling the cards within a hand, that doesn't matter (so long as it still knows which is the turn-up card) . Much of the Cribbage scoring is easier if you break it into separate recursive functions (e.g. one to check for 'fifteens', etc.) o Easier doesn't mean you have to do it; just that you might want to save yourself some work Take a look at the marking scheme below. What do you see? o You aren't graded on how much time you put into the assignment, nor on whether or not the output vaguely resembles what one might expect. You're being told to create and usea well-defined specification. Cobbling together bits and bobs isn't sufficient o Also, some things are explicit, and some aren't; don't assume there's "one way" to solve a problem Corollary to the previous point: if you have a single print statement anywhere other than within your Main class, don't be surprised when you don't receive a passing grade The FaceCard, NumberCard, and Ace: Fully-realized concrete classes .Each has an appropriate toString function FaceCards have a rank (or sequence) of 11 for Jacks, 12 for Queens, and 13 for Kings; but a value of 10 Aces have both a rank and value of 1 . Yes, they collectively only add up to 1 point, since the implementations are nearly trivial The Deck: /2 A newly-instantiated Deck always starts in the same sequence: A-K for Hearts, A- K for Diamonds, . A K for Clubs, and A K for Spades . You'll also have the ability to shuffle it . You need the ability to deal a single PlayingCard . You also need the ability to draw a specified number of cards into an existing (possibly empty) Hand Finally, you'll need the ability to see how many cards are left in the Deck The Hand: /2 Must be an interface Must have accessors for getting the number of cards in the hand (its current size), and for retrieving a specific card (zero-based indexing) Also include a function for removing the card at a zero-based index (which returns said PlayingCard), and for adding a PlayingCard (there's no need to worry about index for adding; let the concrete classes worry about where new cards go) Include either a header for toString or a default implementation, per your preference Finally, and very importantly, you need a default implementation for a tally function o The default tally is simply the sum of the ranks of the cards in the hand The CribbageHand: /2 Provides implementations of everything promised in the Hand interface (of course) Separate from the hand, but tallied with it, is the turn-up/starter card (which you'll need to provide as a constructor parameter) Provide a Cribbage-specific tally implementation o Note that flushes are calculated differently depending on whether or not it's the crib, so you'll also need to add a parameter/instance variable to keep track of which the instance of CribbageHand is The Main class/program: /1 You need to write a simple command-line program to demonstrate the tools you created The specifics are up to you, but make sure to show: o o o Creation and shuffling of a deck (this might simply be implied by the next points) Dealing hands Displaying hands, as well as their scores It should allow for multiple iterations, and be very easy for the marker to test If the marker can't use your program, you don't have a program o Seriously, this may only be worth one point directly, but it's what lets you earn the other points! - Additional: There are several things that couldn't fit into one of the above, including style, coding and commenting conventions, etc. The absence of glaring mistakes earns 1 point Requirements To consolidate: Create a library and sample program for above, and following the marking scheme below Make sure to follow proper Cribbage scoring for hands/cribs .Take special note of where you should have toString's Tips and Suggestions Of course, the most important thing is to work out what you're doing long before you start coding. However, in addition to that: This isn't a data structures course. You're welcome to use Java's built-in LinkedList, ArrayList, etc. Of course, you need basic conventions: o Put your name and student number at the top of each file in commenting o Document each class and method, in JavaDoc convention Everything goes into a deck package Actually, it's up to you whether or not you want your Main class/program to be in the same package or a different one, but everything else does need to be in there There's a reason the cribbage hand is 'four cards plus the turn-up? when playing for real, the turn-up is counted with both player's hands (as well as with the crib) o So, while although most cards are drawn directly into hands, your main program will need to first o Well, I guess the better reason is it's also necessary for counting 'his nobs' There are multiple ways to count the same thing. So long as the total points work out identically, don't worry too much about how you add them o eg. for [7H | 8S, 7S, 7C, AD], the three of a kind' is worth 6 points. Why? You can think of it as 'three pairs': [7H, 7S], 17H, 7C], and [7S, 7C] -You can simply think of it as 'three of a kind', so long as it gives the same 6 points In case it's never come up for you: the reason we include toString so often is because Java invokes it automatically when it expects a String representation of an object o e.g. System.out.println (myCard) wll totally work It's considered acceptable for the sequence of a hand to be changed during tallying, if that helps o i.e. even though you probably won't need to, if your tallying ends up shuffling the cards within a hand, that doesn't matter (so long as it still knows which is the turn-up card) . Much of the Cribbage scoring is easier if you break it into separate recursive functions (e.g. one to check for 'fifteens', etc.) o Easier doesn't mean you have to do it; just that you might want to save yourself some work Take a look at the marking scheme below. What do you see? o You aren't graded on how much time you put into the assignment, nor on whether or not the output vaguely resembles what one might expect. You're being told to create and usea well-defined specification. Cobbling together bits and bobs isn't sufficient o Also, some things are explicit, and some aren't; don't assume there's "one way" to solve a problem Corollary to the previous point: if you have a single print statement anywhere other than within your Main class, don't be surprised when you don't receive a passing grade The FaceCard, NumberCard, and Ace: Fully-realized concrete classes .Each has an appropriate toString function FaceCards have a rank (or sequence) of 11 for Jacks, 12 for Queens, and 13 for Kings; but a value of 10 Aces have both a rank and value of 1 . Yes, they collectively only add up to 1 point, since the implementations are nearly trivial The Deck: /2 A newly-instantiated Deck always starts in the same sequence: A-K for Hearts, A- K for Diamonds, . A K for Clubs, and A K for Spades . You'll also have the ability to shuffle it . You need the ability to deal a single PlayingCard . You also need the ability to draw a specified number of cards into an existing (possibly empty) Hand Finally, you'll need the ability to see how many cards are left in the Deck The Hand: /2 Must be an interface Must have accessors for getting the number of cards in the hand (its current size), and for retrieving a specific card (zero-based indexing) Also include a function for removing the card at a zero-based index (which returns said PlayingCard), and for adding a PlayingCard (there's no need to worry about index for adding; let the concrete classes worry about where new cards go) Include either a header for toString or a default implementation, per your preference Finally, and very importantly, you need a default implementation for a tally function o The default tally is simply the sum of the ranks of the cards in the hand The CribbageHand: /2 Provides implementations of everything promised in the Hand interface (of course) Separate from the hand, but tallied with it, is the turn-up/starter card (which you'll need to provide as a constructor parameter) Provide a Cribbage-specific tally implementation o Note that flushes are calculated differently depending on whether or not it's the crib, so you'll also need to add a parameter/instance variable to keep track of which the instance of CribbageHand is The Main class/program: /1 You need to write a simple command-line program to demonstrate the tools you created The specifics are up to you, but make sure to show: o o o Creation and shuffling of a deck (this might simply be implied by the next points) Dealing hands Displaying hands, as well as their scores It should allow for multiple iterations, and be very easy for the marker to test If the marker can't use your program, you don't have a program o Seriously, this may only be worth one point directly, but it's what lets you earn the other points! - Additional: There are several things that couldn't fit into one of the above, including style, coding and commenting conventions, etc. The absence of glaring mistakes earns 1 point
Step by Step Solution
There are 3 Steps involved in it
Step: 1
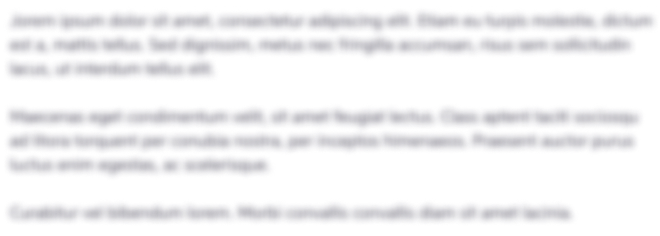
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started