Question
Can someone explain a few things about the code below? 1.The tools, libraries, and APIs utilized 2.Search methods used and how they contributed toward the
Can someone explain a few things about the code below?
1.The tools, libraries, and APIs utilized
2.Search methods used and how they contributed toward the program goal
3.Inclusion of any deep learning models
4.Aspects of the program that utilize expert system concepts
5.How the program represent knowledge
6.How symbolic planning is used in the program
CODE BELOW:
import numpy as np
# Define a simple function for the neural network to learn
def function(x):
return x**2 + 2*x + 1
# Define the neural network architecture
input_size = 1
hidden_size = 16
output_size = 1
# Initialize the weights and biases
W1 = np.random.randn(input_size, hidden_size)
b1 = np.random.randn(hidden_size)
W2 = np.random.randn(hidden_size, output_size)
b2 = np.random.randn(output_size)
# Define the sigmoid activation function
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# Define the derivative of the sigmoid function
def sigmoid_derivative(x):
return x * (1 - x)
# Define the neural network forward pass
def forward(x):
z1 = np.dot(x, W1) + b1
a1 = sigmoid(z1)
z2 = np.dot(a1, W2) + b2
a2 = sigmoid(z2)
return a2
# Define the neural network backward pass
def backward(x, y, a2):
dz2 = a2 - y
dw2 = np.dot(a1.T, dz2)
db2 = np.sum(dz2, axis=0)
da1 = np.dot(dz2, W2.T)
dz1 = da1 * sigmoid_derivative(a1)
dw1 = np.dot(x.T, dz1)
db1 = np.sum(dz1, axis=0)
return dw1, db1, dw2, db2
# Define the A* search algorithm
def a_star(start, goal):
# Define the heuristic function
def heuristic(x):
return abs(x - goal)
# Create a priority queue and add the starting point
queue = [(start, 0)]
# Keep track of the path taken
path = {}
path[start] = None
# Keep track of the cost of each point
cost = {}
cost[start] = 0
while queue:
# Get the point with the lowest f value
current = min(queue, key=lambda x: x[1])[0]
# Check if we have reached the goal
if current == goal:
return path, cost[goal]
# Remove the current point from the queue
queue.remove((current, cost[current] + heuristic(current)))
# Generate the neighbors of the current point
for neighbor in [current - 1, current + 1]:
if neighbor in path:
continue
# Calculate the cost of reaching the neighbor
new_cost = cost[current] + 1
# Add the neighbor to the queue
queue.append((neighbor, new_cost + heuristic(neighbor)))
# Update the path and cost
path[neighbor] = current
cost[neighbor] = new_cost
#Train the neural network
num_epochs = 10000
learning_rate = 0.1
#for epoch in range(num_epochs):
# Generate a random input
x = np.random.randn(1)
# Calculate the output of the neural network
a2 = forward(x)
# Calculate the error
error = function(x) - a2
# Calculate the gradients
dw1, db1, dw2, db2 = backward(x, function(x), a2)
# Update the weights and biases
W1 -= learning_rate * dw1
b1 -= learning_rate * db1
W2 -= learning_rate * dw2
b2 -= learning_rate * db2
#Test the neural network
x = 3
a2 = forward(x)
print("Input:", x)
print("Expected output:", function(x))
print("Predicted output:", a2[0])
#Test the A* search algorithm
start = 0
goal = 10
path, cost = a_star(start, goal)
print("Path from", start, "to", goal, ":", path)
print("Cost:", cost)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
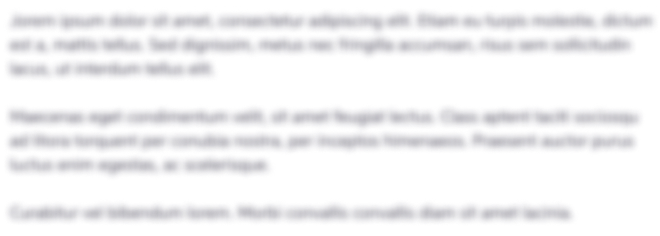
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started