Question
Can someone help me fill in the code for these test scenarios for the StudentCollection Class. The JUnit test should show all of these test
Can someone help me fill in the code for these test scenarios for the StudentCollection Class. The JUnit test should show all of these test cases passing. Code in Java.
//StudentCollectionTest
import org.junit.After;
import org.junit.AfterClass; import org.junit.Before; import org.junit.BeforeClass; import org.junit.Test; import static org.junit.Assert.*;
public class StudentCollectionTest { public StudentCollectionTest() { }
@BeforeClass public static void setUpClass() { }
@AfterClass public static void tearDownClass() { }
@Before public void setUp() { }
@After public void tearDown() { }
@Test public void testGetCapacity() { System.out.println("getCapacity"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); int expResult = 0; int result = instance.getCapacity(); assertEquals(expResult, result); fail("The test case is a prototype."); }
@Test public void testReset() { System.out.println("reset"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); instance.reset(); fail("The test case is a prototype."); }
@Test public void testIsFull() { System.out.println("isFull"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); boolean expResult = false; boolean result = instance.isFull(); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testIsEmpty() { System.out.println("isEmpty"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); boolean expResult = false; boolean result = instance.isEmpty(); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testGetStudentCount() { System.out.println("getStudentCount"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); int expResult = 0; int result = instance.getStudentCount(); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testGetSpacesRemaining() { System.out.println("getSpacesRemaining"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); int expResult = 0; int result = instance.getSpacesRemaining(); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testAddStudent() { System.out.println("addStudent"); studentspec.StudentSpec aStudent = null; studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); instance.addStudent(aStudent); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testRetrieveStudentBySID() { System.out.println("retrieveStudentBySID"); java.lang.String sidKey = ""; studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); studentspec.StudentSpec expResult = null; studentspec.StudentSpec result = instance.retrieveStudentBySID(sidKey); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testRemoveStudentBySID() { System.out.println("removeStudentBySID"); java.lang.String sidKey = ""; studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); studentspec.StudentSpec expResult = null; studentspec.StudentSpec result = instance.removeStudentBySID(sidKey); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test public void testToString() { System.out.println("toString"); StudentCollectionSpec instance = StudentCollection.createStudentCollection(); String expResult = ""; String result = instance.toString(); assertEquals(expResult, result); // TODO review the generated test code and remove the default call to fail. fail("The test case is a prototype."); }
@Test
public void testCreateIterator() { System.out.println("createIterator"); studentcollection.StudentCollection instance = new studentcollection.StudentCollection(); java.util.Iterator expResult = null; java.util.Iterator result = instance.createIterator(); assertEquals(expResult, result); fail("The test case is a prototype."); } }
================================================================================
//StudentCollection.java
package studentcollection;
public class StudentCollection implements StudentCollectionSpec { public StudentCollection(int capacity) { }
public StudentCollection() {
}
@Override public int getCapacity() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void reset() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public boolean isFull() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public boolean isEmpty() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public int getStudentCount() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public int getSpacesRemaining() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void addStudent( studentspec.StudentSpec aStudent ) throws studentcollection.StudentCollectionSpec.StudentCollectionException { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public studentspec.StudentSpec retrieveStudentBySID( java.lang.String sidKey ) { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public studentspec.StudentSpec removeStudentBySID( java.lang.String sidKey ) { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public java.util.Iterator
}
===================================================================================
package studentcollection;
//StudentCollectionSpec.java
import java.io.Serializable; import studentspec.StudentSpec;
import java.util.Iterator;
public interface StudentCollectionSpec extends Serializable { public int getCapacity();
public void reset();
public boolean isFull();
public boolean isEmpty();
public int getStudentCount();
public int getSpacesRemaining();
public void addStudent(StudentSpec aStudent) throws StudentCollectionException;
public StudentSpec retrieveStudentBySID(String sidKey);
public StudentSpec removeStudentBySID(String sidKey);
@Override public String toString();
public Iterator
public class StudentCollectionException extends RuntimeException { private static final long serialVersionUID = 8157997298448463708L;
/* constructor that accepts a direct reason why the exception is to be created. */ public StudentCollectionException(String cause) { super(cause); }
public StudentCollectionException(String cause, Throwable causalException) { super(cause, causalException); }
}
}
===============================================================================
//Student.java
package studentspec;
public class Student implements StudentSpec {
public Student( String theSID, String theFirstName, String theMiddleInitial, String theLastName, String theMajor, double theTotalDegreeCredits, double theTotalQualityPoints ) {
}
@Override public java.lang.String getFirstName() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } public Student( String theSID, String theFirstName, String theMiddleInitial, String theLastName, String theMajor ) {
}
@Override public java.lang.String getMiddleInitial() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public java.lang.String getLastName() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public java.lang.String getSID() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public double getTotalDegreeCredits() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public double getTotalQualityPoints() { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public studentspec.StudentSpec clone() throws java.lang.CloneNotSupportedException { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void setFirstName( java.lang.String theFirstName ) throws studentspec.StudentSpec.StudentSpecException { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void setMiddleInitial( java.lang.String theMiddleInitial ) { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void setLastName( java.lang.String theLastName ) throws studentspec.StudentSpec.StudentSpecException { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void setSID( java.lang.String theSID ) throws studentspec.StudentSpec.StudentSpecException { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void setGPAIngredients( double theTotalDegreeCredits, double theTotalQualityPoints ) throws studentspec.StudentSpec.StudentSpecException { throw new java.lang.UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
}
===============================================================================
//StudentSpec.java
package studentspec;
import java.io.Serializable;
public interface StudentSpec extends Cloneable, Serializable { public static final double MAX_GPA = 4.0; public static final double MIN_GPA = 0.0; public static final double DEFAULT_TOTAL_DEGREE_CREDITS = 0.0; public static final double DEFAULT_TOTAL_QUALITY_POINTS = 0.0;
public String getFirstName();
public String getMiddleInitial();
public String getLastName();
public String getSID();
public double getTotalDegreeCredits();
public double getTotalQualityPoints();
@Override public String toString();
public StudentSpec clone() throws CloneNotSupportedException;
public void setFirstName(String theFirstName) throws StudentSpecException;
public void setMiddleInitial(String theMiddleInitial);
public void setLastName(String theLastName) throws StudentSpecException;
public void setSID(String theSID) throws StudentSpecException;
public void setGPAIngredients(double theTotalDegreeCredits, double theTotalQualityPoints) throws StudentSpecException;
public class StudentSpecException extends RuntimeException implements Serializable { private static final long serialVersionUID = 5790641133393241596L; public StudentSpecException(String cause) { super(cause); }
public StudentSpecException(String cause, Exception causalException) { super(cause, causalException); }
}
}
==============================================================================
Step by Step Solution
There are 3 Steps involved in it
Step: 1
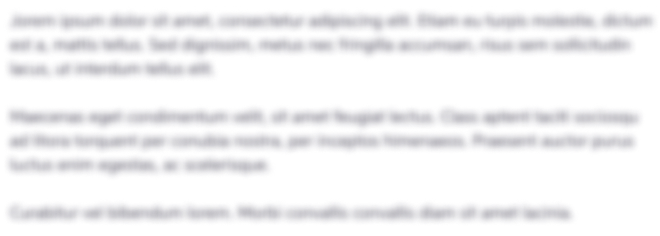
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started