Question
Can someone help me with my java code. I'm not sure how to do the rest. Just with ChatClient and ChatServer CHAT CLIENT: package lab3in;
Can someone help me with my java code. I'm not sure how to do the rest. Just with ChatClient and ChatServer
CHAT CLIENT:
package lab3in;
import ocsf.client.AbstractClient;
public class ChatClient extends AbstractClient{
public ChatClient() {
super("localhost",8300);
}
public void handleMessageFromServer(Object msg) {
if (msg instanceof String){
System.out.println("Server Message sent to Client "+ (String)msg);
}
}
public void connectionException (Throwable exception) {
System.out.println(exception.getMessage());
exception.printStackTrace();
}
public void connectionEstablished()
{ }
}
Chat SERVER
package lab3in;
import java.io.IOException;
import ocsf.server.AbstractServer; import ocsf.server.ConnectionToClient;
public class ChatServer extends AbstractServer{
public ChatServer() { super(12345); this.setTimeout(500); } @Override protected void handleMessageFromClient(Object arg0, ConnectionToClient arg1) { // TODO Auto-generated method stub String a = (String) arg0; int b = (int) arg1.getId(); System.out.println("Client " + " "+b +" "+ a ); try { arg1.sendToClient("Hello Client"); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void listeningException(Throwable exception) { System.out.println("Listening Exception Occurred"); System.out.println(exception.getMessage()); exception.printStackTrace(); } public void serverStarted() { System.out.println("Server Started"); } }
Hook method for client. Iavoked whenever the client has successfully connected to the server Set the status JLabel of the GUI to the word "Connected" and colors it Green within this method. One Client Connects to the Server The Client Sends any message to the Server (in this case it's in the form of a String) The Server will send the exact same message it received back to the Client Lab 3out will utilize 3 classes implemented in the previous labs -ChatServs ServeGu and ClientGUI. You will complete the implementation of ChatClien that was started in lab3in. ChatClient must extend AbstractClient public void bandlekessaeeEnonsecvec(object arge) Slot method for client. Invoked whenever the server has sent a message to the Client Display the contents of parameter arge (for this lab you can assume arge is always a String) to the TextArea of the GUI that contains the messages received from the Server. The secvecMsg data field corresponds to where this message should be displayed. The displayed messape should be formatted as follows: You will also need to perform 0me modifications to the Chas ner. SezerGAZ and Client LZ classes. Server. arg0 Where arg0 represents the String sent from the server. For instance, suppose the server sent the words "Hello Everyone" to the client. Then the client should display the following in the Server Response extArea of the ClientGI class: ChatClient Implement a Java Class named ChatClient (ChatClierst must extend AkstactClnt Make sure that Implement abstract methods" is selected when creating the ChatClient class. This class should implement the following private data fields Server: Hello Everyone Note: The server will be sending two types of messages b ack to the Client: private ahel status; private 3TextAcea SSCMEME: private Jextield clieotin: 1. The server will send back exactly what the client has sent it (via client's submit button) Where status is the topmost status JLabel of the GUI displayed by the ClientGUI class, secuscusg is the TetArea corresponding to messages received from the ChatServer class, and clientIn is the topmost, non editable Textield containing the clientID that eventually is assigned by the server 2. The server will send back a key/value pair response expecting the Client to use the response im some way For this system, the second type of response involves the server sending the client a key/value pair that the client must parse and use in some way (similar to a command). The responses are in the form of key/value pairs formatted as follows: The method description for ChaClient is given below public chatslient) Default Constructor for the ChatClignt, Should invoke the Base class Constructor that accepts 2 parameters- host and port. You can just set the host and port to some default values in this method. Where the" separates the key from the value. There should be no white space in either the key or the value. Only one such response of this type will be utilized in this lab it will have the following form: public void setstatusaabel status) Sets the private data field status to the input parameter status. The input parameter corresponds to the status JLabel created in the ClieniGU Where usemame is the key, while client-id is the client id as assigned by the server (ie, the client cannot choose their own id). Sets the private data field secverusg to the input parameter secve The input parameter corresponds to the JTetArea containing the server responses created in the ClisGU! public void Sets the private data field slieatIe to the input parameter lient. The input parameter corresponds to the JTextField containing the client ID created in the ClintGU. The server should send this response back to the client as soon as the client has successfully connected to the server (performed within the clientsonpected method of the server SlientID) An example response of this form is shown below public void SDosctisnEstablisbed) username: client-18 Where client-18 is the username for the client as assigned by the server You will need to add the following private data field to the Cli class: private Chatclient client; This private data field should be instantiated with the Constructor of the ClieniGU class The ClieGU Constructor should set the host, port, stats, serse, and gliextD data fields method encounters this type of response, Whenever the handleessageEcgserver should parse the username from the value and then display the newly assigned client's id in the 1st ITextField labeled as Client ID the method This method should set the Client ID client-id sent from the server extField created within the gientGUI class to the of the client object Pressing the "Connect" JButton will invoke the openconnection method of the client object. Pressing the "Submit" JButton will send the message in the Client TextArea to the Server Pressing the "Stop" JButton will invoke the closeconnection method on the client object. public void cannestianclosed.c) Hook method. Displays the String "Not Connected" in the status JLabel colored Red. Echo Server Process Sequence Start the ServerGUII class and fill in the GUI as follows Modify the following methods: Server Status: Not Consected public void Display the message in the Server Log TestArea using the following format: ject arge, CnnestionTaclient arg1) Port # Where client-id is the id of the client retrieved by invoking method getid on parameter argi (e.g., argi.getId)) and messags.fron.slicnt is the String contained in parameter arge Server Log Below Next, send the message contained in arge (assume arge is String for this lab) exactly back to the Client identified by argi. public void Sends client) following String formatted as: a response back to the client in the Listes CloseSIop Quit Where the usemame is considered a key, while the value associated with this key, client-id, is a String which is constructed as follows: Press the Listen" Button and the SenverGUI should be displayed as follows: "Client, Guentetto Also display in the Server Log TextArea "Client client-id Connected" Server Stas Listaning Port imeost Setver Log Server Log Below Note how the clieutiD is set automatically by the server. Next enter "Hello Everyone" in the "Client Data"mArea as shown below: Listen CloseStopQuit Nest, the should be started and the TextFields should be filled in as shown below Client #1 Cent #1 Server URL 127001 Server URL 12700.1 Receired Server Deta usemameclent1 ConnectSubmit Sop Pressing the "Connecf" button results in the following modifications to the Serve and Pressing "Submit results in the following modifications to both the ClientGUI and SevedGLI simultaneously Client #1 Server tatus Listening Server Port Hell Evryone Server Log Below Clent 19 Connece Helo Everyone Hello Everyone Listen CleseSop u Consect Subit Stop Pressing the "Stop" Button on the ClientGU invokes theloseconestion method on the ChatClient object and displays "Not Connected" in the status JLabel colored Red. The user will have to press "Connect"Buttoa again before the client can reconnect to the server. Pressing the "Close", "Stop" and "Quit',uttona on the SenerGU[ results in the same functionality as in lab2out The ClieatGUI should still receive the 1 command line argument as in lablout- the title of application. The ServerGJI shoud still receive the 1 command line argument as in lablout and lab2out- the title of the application Hook method for client. Iavoked whenever the client has successfully connected to the server Set the status JLabel of the GUI to the word "Connected" and colors it Green within this method. One Client Connects to the Server The Client Sends any message to the Server (in this case it's in the form of a String) The Server will send the exact same message it received back to the Client Lab 3out will utilize 3 classes implemented in the previous labs -ChatServs ServeGu and ClientGUI. You will complete the implementation of ChatClien that was started in lab3in. ChatClient must extend AbstractClient public void bandlekessaeeEnonsecvec(object arge) Slot method for client. Invoked whenever the server has sent a message to the Client Display the contents of parameter arge (for this lab you can assume arge is always a String) to the TextArea of the GUI that contains the messages received from the Server. The secvecMsg data field corresponds to where this message should be displayed. The displayed messape should be formatted as follows: You will also need to perform 0me modifications to the Chas ner. SezerGAZ and Client LZ classes. Server. arg0 Where arg0 represents the String sent from the server. For instance, suppose the server sent the words "Hello Everyone" to the client. Then the client should display the following in the Server Response extArea of the ClientGI class: ChatClient Implement a Java Class named ChatClient (ChatClierst must extend AkstactClnt Make sure that Implement abstract methods" is selected when creating the ChatClient class. This class should implement the following private data fields Server: Hello Everyone Note: The server will be sending two types of messages b ack to the Client: private ahel status; private 3TextAcea SSCMEME: private Jextield clieotin: 1. The server will send back exactly what the client has sent it (via client's submit button) Where status is the topmost status JLabel of the GUI displayed by the ClientGUI class, secuscusg is the TetArea corresponding to messages received from the ChatServer class, and clientIn is the topmost, non editable Textield containing the clientID that eventually is assigned by the server 2. The server will send back a key/value pair response expecting the Client to use the response im some way For this system, the second type of response involves the server sending the client a key/value pair that the client must parse and use in some way (similar to a command). The responses are in the form of key/value pairs formatted as follows: The method description for ChaClient is given below public chatslient) Default Constructor for the ChatClignt, Should invoke the Base class Constructor that accepts 2 parameters- host and port. You can just set the host and port to some default values in this method. Where the" separates the key from the value. There should be no white space in either the key or the value. Only one such response of this type will be utilized in this lab it will have the following form: public void setstatusaabel status) Sets the private data field status to the input parameter status. The input parameter corresponds to the status JLabel created in the ClieniGU Where usemame is the key, while client-id is the client id as assigned by the server (ie, the client cannot choose their own id). Sets the private data field secverusg to the input parameter secve The input parameter corresponds to the JTetArea containing the server responses created in the ClisGU! public void Sets the private data field slieatIe to the input parameter lient. The input parameter corresponds to the JTextField containing the client ID created in the ClintGU. The server should send this response back to the client as soon as the client has successfully connected to the server (performed within the clientsonpected method of the server SlientID) An example response of this form is shown below public void SDosctisnEstablisbed) username: client-18 Where client-18 is the username for the client as assigned by the server You will need to add the following private data field to the Cli class: private Chatclient client; This private data field should be instantiated with the Constructor of the ClieniGU class The ClieGU Constructor should set the host, port, stats, serse, and gliextD data fields method encounters this type of response, Whenever the handleessageEcgserver should parse the username from the value and then display the newly assigned client's id in the 1st ITextField labeled as Client ID the method This method should set the Client ID client-id sent from the server extField created within the gientGUI class to the of the client object Pressing the "Connect" JButton will invoke the openconnection method of the client object. Pressing the "Submit" JButton will send the message in the Client TextArea to the Server Pressing the "Stop" JButton will invoke the closeconnection method on the client object. public void cannestianclosed.c) Hook method. Displays the String "Not Connected" in the status JLabel colored Red. Echo Server Process Sequence Start the ServerGUII class and fill in the GUI as follows Modify the following methods: Server Status: Not Consected public void Display the message in the Server Log TestArea using the following format: ject arge, CnnestionTaclient arg1) Port # Where client-id is the id of the client retrieved by invoking method getid on parameter argi (e.g., argi.getId)) and messags.fron.slicnt is the String contained in parameter arge Server Log Below Next, send the message contained in arge (assume arge is String for this lab) exactly back to the Client identified by argi. public void Sends client) following String formatted as: a response back to the client in the Listes CloseSIop Quit Where the usemame is considered a key, while the value associated with this key, client-id, is a String which is constructed as follows: Press the Listen" Button and the SenverGUI should be displayed as follows: "Client, Guentetto Also display in the Server Log TextArea "Client client-id Connected" Server Stas Listaning Port imeost Setver Log Server Log Below Note how the clieutiD is set automatically by the server. Next enter "Hello Everyone" in the "Client Data"mArea as shown below: Listen CloseStopQuit Nest, the should be started and the TextFields should be filled in as shown below Client #1 Cent #1 Server URL 127001 Server URL 12700.1 Receired Server Deta usemameclent1 ConnectSubmit Sop Pressing the "Connecf" button results in the following modifications to the Serve and Pressing "Submit results in the following modifications to both the ClientGUI and SevedGLI simultaneously Client #1 Server tatus Listening Server Port Hell Evryone Server Log Below Clent 19 Connece Helo Everyone Hello Everyone Listen CleseSop u Consect Subit Stop Pressing the "Stop" Button on the ClientGU invokes theloseconestion method on the ChatClient object and displays "Not Connected" in the status JLabel colored Red. The user will have to press "Connect"Buttoa again before the client can reconnect to the server. Pressing the "Close", "Stop" and "Quit',uttona on the SenerGU[ results in the same functionality as in lab2out The ClieatGUI should still receive the 1 command line argument as in lablout- the title of application. The ServerGJI shoud still receive the 1 command line argument as in lablout and lab2out- the title of the applicationStep by Step Solution
There are 3 Steps involved in it
Step: 1
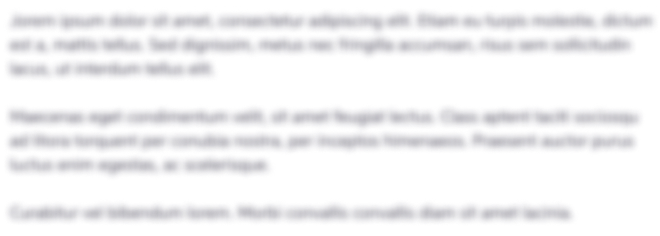
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started