Question
can someone help me with this in C++ You are asked to create a program for storing the catalog of movies at a DVD store
can someone help me with this in C++
You are asked to create a program for storing the catalog of movies at a DVD store using functions. The program should let the user add, remove, sort, and output movies.
The main function is provided (you need to modify the code of the main function to call the user-defined functions described below). You must write the definition and implementation of the following functions:
1) printCatalog:
The function print all movies in the dynamic array movies.
2) findMovie:
The function returns the index at which the movie name is located in the dynamic array with the names of the movies in the catalog. If the movie is not in the catalog, the function must return -1.
3) addMovie:
The function adds the information about the new movie to the dynamic array and does not return a value.
4) removeMovie:
if the movie is in the catalog, the function removes all the information about the movie from the dynamic array representing the catalog. Otherwise, print out "cannot fine the movie", the function does not return a value.
this is the code I have so far
#include
using namespace std;
struct movie{ int year; string genre; string name; };
// Write the definition and implementation of the printCatalog function here void printCatalog(movie *_movies, int _nMovies){ } // Write the definition and implementation of the findMovie function here int findMovie(movie *_movies, int _nMovies, const string &_name){ } // Write the definition and implementation of the addMovie function here void addMovie(const string &_name, int _year, const string &_genre, movie *_movies, int _size, int &_nMovies){ } // Write the definition and implementation of the removeMovie function here void removeMovie(const string &_name, movie *_movies, int &_nMovies){ }
int main() { movie *movies; int size; int nMovies = 0;
//get the size of our dynamic array from keyboard //create dynamic array with the size
//call addMovie with name: Free Guy year: 2021 Genre: Action //call addMovie with name: Deadpool year: 2016 Genre: Action //call removeMovie with name : Deadpool //call removeMovie with name : Terminator //call printCatalog //delete the dynamic array movies; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
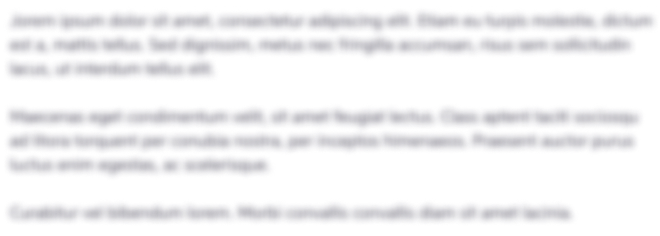
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started